How to Link an Image in React
Related: How to Use an Image as a Link in React
To link a local image in React, import
the image at the top of the file and assign it to the src
prop of an img
element.
For example:
App.js
// 👇 import image from file
import myImage from './my-image.jpg';
export default function App() {
return (
<div>
{/* 👇 show image */}
<img src={myImage} alt="Trees" height="200" />
<br />
<span
style={{
color: 'green',
fontSize: '1.2em',
fontWeight: 'bold',
}}
>
Trees
</span>
</div>
);
}
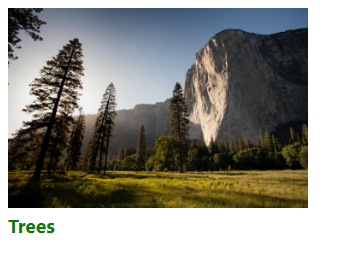
This approach works when using a Webpack-based tool like Create React App.
Note that the image file must be within the project directory to be imported successfully. An error will occur if the file is outside the project directory.
Link image in React with require()
function
Alternatively, we can use the require()
function to link an image in React.
The following code example will produce precisely the same result on the page as the first example.
App.js
export default function App() {
return (
<div>
{/* 👇 */}
<img
src={require('./my-image.jpg')}
alt="Trees"
height="200"
/>
<br />
<span
style={{
color: 'green',
fontSize: '1.2em',
fontWeight: 'bold',
}}
>
Trees
</span>
</div>
);
}
The advantage require()
has here is that it doesn’t need to be at the top of the page. We can simply assign the result of require()
to the src
prop without having to store it in a variable.
Link image in public
folder
Another way to link an image in React is to place it in the public
folder and reference it with its relative path.
For example, if we placed the my-image.png
file in the public
folder, we’ll be able to display it in the page like this:
App.js
export default function App() {
return (
<div>
{/* 👇 show image */}
<img src="./my-image.jpg" alt="Trees" height="200" />
<br />
<span
style={{
color: 'green',
fontSize: '1.2em',
fontWeight: 'bold',
}}
>
Trees
</span>
</div>
);
}
Using the public
folder is advantageous when we have many images that we want to display dynamically.
In the following examples, we dynamically display 100 images placed in a grid
subfolder under public
, and named with a certain pattern (image-1.jpg
, image-2.jpg
, …, image-100.png
) in a grid.
App.js
export default function App() {
return (
<div>
<div
style={{
display: 'grid',
gridTemplateColumns:
'repeat(auto-fit, minmax(min-content, 250px))',
gap: '8px',
}}
>
{[...Array(100)].map((_, i) => {
return (
<div>
{/* 👇 linking images dynamically */}
<img
key={i}
src={`./grid/image-${i + 1}.jpg`}
style={{ width: '100%', height: 'auto' }}
/>
</div>
);
})}
</div>
</div>
);
}
Link remote image
If the image is stored online, then we’ll just have to set the URL of the image to the src
prop to link and display it, like we do in plain HTML.
App.js
export default function App() {
return (
<div>
{/* 👇 show remote image */}
<img
src="http://example.com/trees/my-image.jpg"
alt="Trees"
height="200"
/>
<br />
<span
style={{
color: 'green',
fontSize: '1.2em',
fontWeight: 'bold',
}}
>
Trees
</span>
</div>
);
}