In this article, we’ll learn how to easily remove an element onclick in React, whether it’s in a list or it’s a standalone element.
Remove stand-alone element onclick in React
To remove a stand-alone element onclick:
- Store the visibility state in a state variable as a
Boolean
value, and use its value to conditionally render the element. - Attach an event handler to the
onClick
event of the element. - In the event handler, negate the value of the visibility state to remove the element from the DOM.
One example should make this clear:
import { useState } from 'react';
export default function App() {
const [visible, setVisible] = useState(true);
const removeElement = () => {
setVisible((prev) => !prev);
};
return (
<div>
Click to remove element
<br />
{visible && (
<button onClick={removeElement}>Remove</button>
)}
</div>
);
}
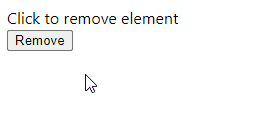
We use the useState()
to create a state variable in the component. This hook returns an array of two variables, generally called state
and setState
. The state
variable (visible
) holds the current visibility state, and the setState
function (setVisible
) updates it.
const [visible, setVisible] = useState(true);
We set an event handler to the onClick
event of the button, so when the button is clicked, the handler function will be called.
<button onClick={removeElement}>Remove</button>
In the handler function, we use the setState
function for the visibility state to update the state.
const removeElement = () => {
setVisible((prev) => !prev);
};
Instead of passing the negated value directly, we pass a callback function to setState
that returns the negated value. This ensures that we always get the most recent state value.
Tip
React batches state changes for performance reasons, so the state may not be updated immediately after setState
is called, in the order we might expect. This is why we always pass a function to setState
when the new state is computed from the data of the previous state.
Remove element from list onclick
To remove an element from a list onclick:
- Attach an event handler to the
onClick
event of every element in the array representing the list. - In the event handler for a particular element, call the
filter()
method on the array, specifying a condition that istrue
for every element in the array apart from the one to be removed. - Use
setState
to update the state array with the result returned fromfilter()
.
For example:
import { useState } from 'react';
export default function App() {
const [fruits, setFruits] = useState([
'Orange',
'Banana',
'Apple',
]);
const removeElement = (index) => {
const newFruits = fruits.filter((_, i) => i !== index);
setFruits(newFruits);
};
return (
<div>
{fruits.map((fruit, index) => (
<div key={index}>
<button
onClick={() => removeElement(index)}
>
{fruit}
</button>
<br />
<br />
</div>
))}
</div>
);
}
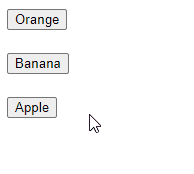
With the map()
method, we render a button for each element in the array. For each button, we attach an event handler that will call the removeElement()
method, passing as an argument the index of the element that the button represents.
{fruits.map((fruit, index) => (
<div key={index}>
<button
onClick={() => removeElement(index)}
>
{fruit}
</button>
<br />
<br />
</div>
))}
removeElement()
removes an element by returning a condition from the filter() callback that is true
only for elements in the array that don’t have the index
passed to removeIndex()
. Doing this excludes the element with that index
from the array, so when the array state is updated, the button representing that element is no longer rendered.
const fruits = ['Orange', 'Banana', 'Apple'];
const newFruits = fruits.filter((_, index) => index !== 1);
console.log(newFruits); // [ 'Orange', 'Apple' ]
Note: don’t modify state directly in React
Trying to remove the element from the array by modifying it using a function like splice()
will not work:
const removeElement = (index) => {
// ⚠️ Mutating the array like this will not update the view
fruits.splice(index, 1);
};
State is meant to be immutable in React, so we can’t update the array by mutating it. It has to be replaced with a new array returned from filter()
for the view to update.
Remove object element from list onclick
We can also use this approach to remove an element represented by an object from a list onclick.
import { useState } from 'react';
export default function App() {
const [fruits, setFruits] = useState([
{ id: 1, name: 'Orange' },
{ id: 2, name: 'Banana' },
{ id: 3, name: 'Apple' },
]);
const removeElement = (id) => {
const newFruits = fruits.filter(
(fruit) => fruit.id !== id
);
setFruits(newFruits);
};
return (
<div>
{fruits.map((fruit) => (
<div key={fruit.id}>
<button onClick={() => removeElement(fruit.id)}>
{fruit.name}
</button>
<br />
<br />
</div>
))}
</div>
);
}
Instead of filtering by index, this time we filter by the id
property to remove an item from the array and remove the element from the list in the DOM.
const fruits = [
{ id: 1, name: 'Orange' },
{ id: 2, name: 'Banana' },
{ id: 3, name: 'Apple' },
];
const newFruits = fruits.filter((fruit) => fruit.id !== 2);
console.log(newFruits);
// [ { id: 1, name: 'Orange' }, { id: 3, name: 'Apple' } ]
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
