Related: How to Link an Image in React
To use an image as a link in React, wrap the image in an anchor (a
) tag. Clicking an image link will make the browser navigate to the specified URL.
For example:
App.js
import cbLogo from './cb-logo.png';
export default function App() {
return (
<div>
Click the logo to navigate to the site
<br />
<br />
<a href="https://codingbeautydev.com" target="_blank" rel="noreferrer">
<img src={cbLogo} alt="Coding Beauty logo"></img>
</a>
</div>
);
}
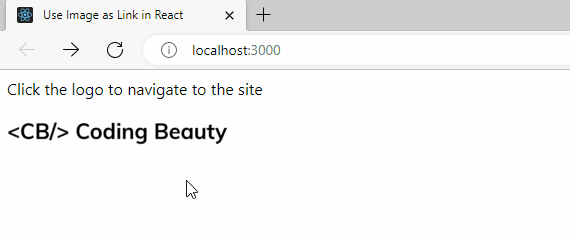
We use an import
statement to link the image into the file, and assign it to the src
prop of the img
element to display it.
The properties set on an a
element will work as usual when it wraps an image. For instance, in the example, we set the a
element’s target property to _blank
, to open the URL in a new tab. Removing this will make it open in the same tab as normal.
We also set the rel
prop to noreferrer
for security purposes. It prevents the opened page from gaining access to any information about the page from which it was opened from.
Use image as React Router link
For React Router, you can use an image as link by wrapping the image in a Link
element.
For example:
ImagePages.jsx
import { Link } from 'react-router-dom';
export default function ImagesPage() {
return (
<div>
<Link to="/nature" target="_blank" rel="noreferrer">
<img src="/photos/tree-1.png" alt="Nature"></img>
</Link>
</div>
);
}
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
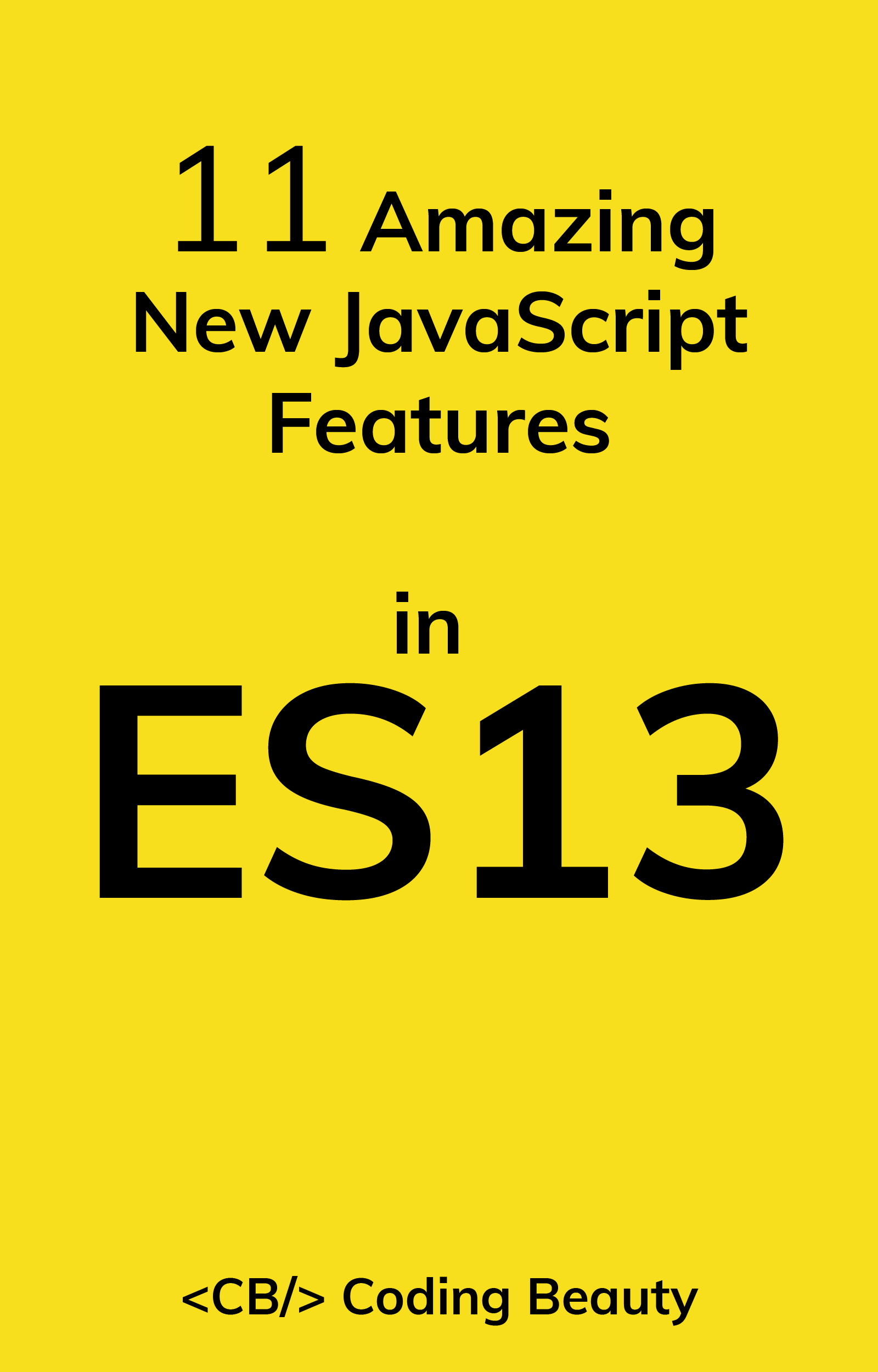