To make text bold in React, wrap the text with a span
element, and set the fontWeight
style property of the span
to bold
.
For example:
App.js
export default function App() {
return (
<div>
Coding{' '}
<span style={{ fontWeight: 'bold' }}>Beauty</span>
</div>
);
}
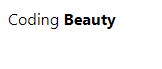
We used inline styles to make the text bold. The style
attribute of a React element accepts a JavaScript object with camelCased properties instead of a CSS kebab-cased string. So, fontWeight
sets the font-weight
CSS property.
If we don’t need to control the boldness with a condition, then we can just wrap the text with a b
element.
App.js
export default function App() {
return (
<div>
Coding <b>Beauty</b>
</div>
);
}
Conditionally bold text with inline styles
Sometimes we might want to make a piece of text bold only if a certain condition is true
. Here’s how we can do this:
App.js
import { useState } from 'react';
export default function App() {
const [bold, setBold] = useState(false);
const handleBoldChange = (event) => {
setBold(event.target.checked);
};
return (
<div>
Coding{' '}
<span
style={{ fontWeight: bold ? 'bold' : 'normal' }}
>
Beauty
</span>
<br></br>
<input
name="bold"
type="checkbox"
value={bold}
onChange={handleBoldChange}
></input>
<label htmlFor="bold">Bold</label>
</div>
);
}
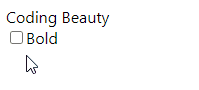
We use a state variable named bold
to store the current checked state of the checkbox and to determine whether the relevant text should be bold or not.
We attach an event listener to the onChange
event, so it is called when the checkbox is checked or unchecked. In this listener, we use the setBold
function to update the value of bold
and change the boldness of the text.
Bold text with custom component
If we frequently need make text bold, we can abstract the logic into a reusable custom component.
App.js
function BoldText({ children }) {
return (
<span style={{ fontWeight: 'bold' }}>{children}</span>
);
}
export default function App() {
return (
<div>
Coding <BoldText>Beauty</BoldText>
</div>
);
}
This code will produce the same result on the web page.
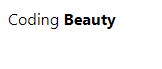
Whatever text is place within the <BoldText>
and </BoldText>
tags will have a bold font.
Conditionally bold text with custom component
To make text bold conditionally with a custom component, we can create a boolean bold
property that will determine whether the child text of the component should be bold or not.
App.js
import { useState } from 'react';
function BoldText({ children, bold }) {
return (
<span style={{ fontWeight: bold ? 'bold' : 'normal' }}>
{children}
</span>
);
}
export default function App() {
const [bold, setBold] = useState(false);
const handleBoldChange = (event) => {
setBold(event.target.checked);
};
return (
<div>
Coding <BoldText bold={bold}>Beauty</BoldText>
<br></br>
<input
name="bold"
type="checkbox"
value={bold}
onChange={handleBoldChange}
></input>
<label htmlFor="bold">Bold</label>
</div>
);
}
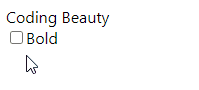
Bold text with class
Alternatively, we can make text bold in React by defining a bold
class in a CSS file, e.g., App.css
:
App.css
.bold {
font-weight: bold;
}
We would then import the App.css
file and apply the bold
class to a span
element to make all the text within it bold.
App.js
import './App.css';
export default function App() {
return (
<div>
Coding <span className="bold">Beauty</span>
</div>
);
}
And this would produce exactly the same result as the previous two methods:
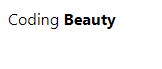
Conditionally bold text with class
Here’s how we would use a class name to make text bold only if a particular condition is true
:
App.js
import { useState } from 'react';
import './App.css';
export default function App() {
const [bold, setBold] = useState(false);
const handleBoldChange = (event) => {
setBold(event.target.checked);
};
return (
<div>
Coding{' '}
<span className={bold ? 'bold' : ''}>Beauty</span>
<br></br>
<input
name="bold"
type="checkbox"
value={bold}
onChange={handleBoldChange}
></input>
<label htmlFor="bold">Bold</label>
</div>
);
}
Using the ternary operator, we set the className
property to the bold
class if the bold
variable is true
. Otherwise, we set className
to an empty string (''
).
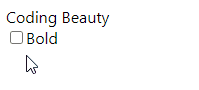
Tip: Instead of the ternary operator, you can use the clsx utility from NPM to more easily construct class names from a set of conditions in React.
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
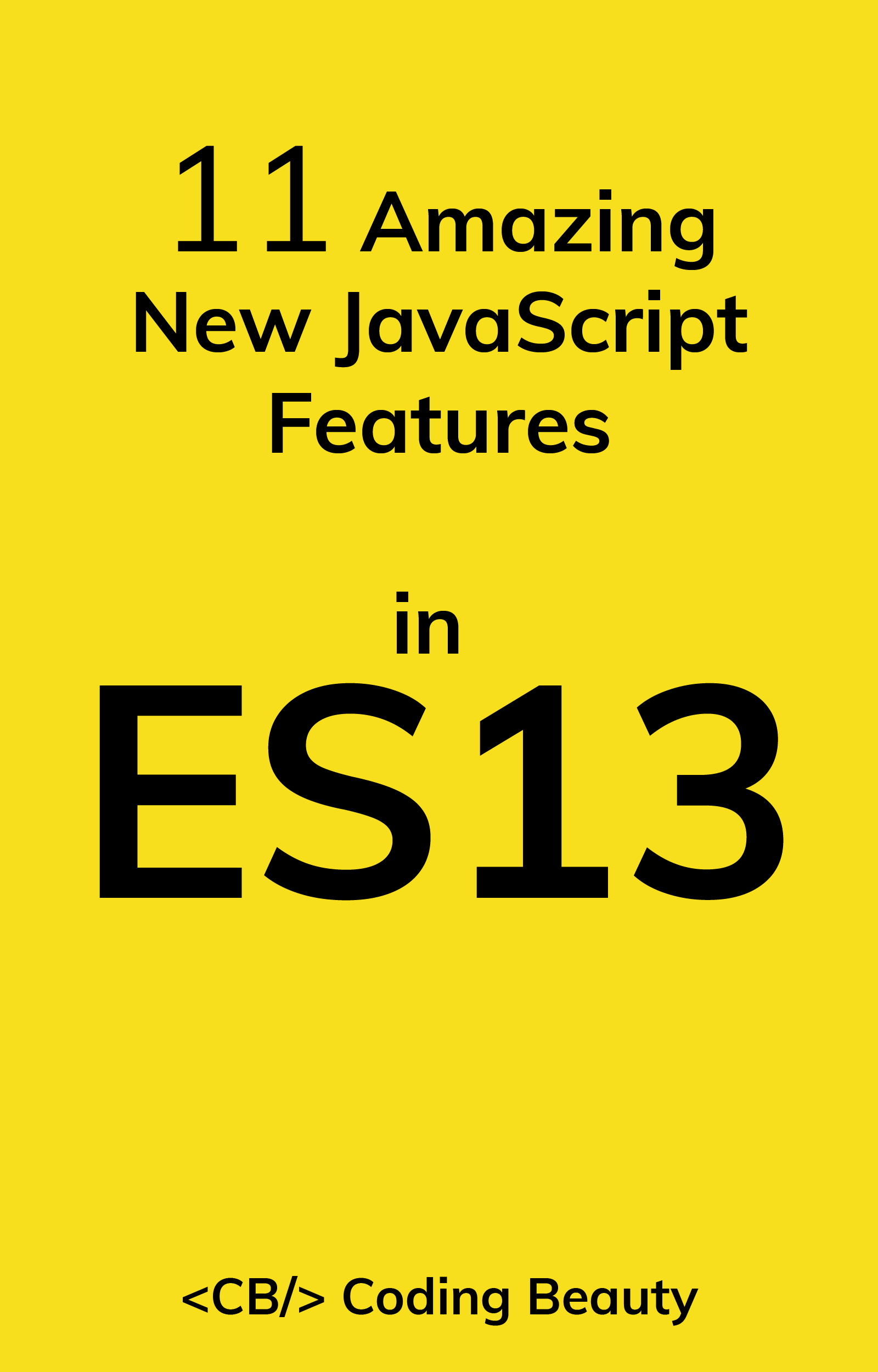