To get the short name of a month in JavaScript, create a Date
object with the given month, then call the toLocaleString()
method on the Date
with a given locale and a set of options. One of the options should specify that the month name should be in a short form.
For example, here’s how we can get the 3-letter month name:
function getMonthShortName(monthNo) {
const date = new Date();
date.setMonth(monthNo - 1);
return date.toLocaleString('en-US', { month: 'short' });
}
console.log(getMonthShortName(1)); // Jan
console.log(getMonthShortName(2)); // Feb
console.log(getMonthShortName(3)); // Mar
We can get the 1-letter month name by setting month
to narrow
:
function getMonthShortName(monthNo) {
const date = new Date();
date.setMonth(monthNo - 1);
return date.toLocaleString('en-US', { month: 'narrow' });
}
console.log(getMonthShortName(1)); // J
console.log(getMonthShortName(2)); // F
console.log(getMonthShortName(3)); // M
Our getMonthShortName()
function takes a position and returns the short name of the month with that position.
The setMonth()
method sets the month of a Date
object to a specified number.
Note
The value passed to setMonth()
is expected to be zero-based. For example, a value of 0
represents January, 1
represents February, 2
represents March, and so on. This why we pass the value of 1
subtracted from the month number (monthNumber - 1
) to setMonth()
.
Date toLocaleString() method
We used the Date toLocaleString() method to get the name of the month of the date. toLocaleString()
returns a string with a language-sensitive representation of a date.
This method has two parameters:
locales
: A string with a BCP 47 language tag, or an array of such strings. There are many locales we can specify, likeen-US
for US English,en-GB
for UK English, anden-CA
for Canadian English.options
: An object used to adjust the output format of the date.
In our examples, we pass en-US
as the language tag to use US English, and we set values of short
and narrow
to the month
property of the options object to display the short name of the month.
We can pass an empty array ([]
) as the first argument to make toLocaleString()
use the browser’s default locale:
function getMonthShortName(monthNo) {
const date = new Date();
date.setMonth(monthNo - 1);
// Use the browser's default locale
return date.toLocaleString([], { month: 'short' });
}
console.log(getMonthShortName(1)); // Jan
console.log(getMonthShortName(2)); // Feb
console.log(getMonthShortName(3)); // Mar
This is good for internationalization, as the output will vary depending on the user’s preferred language.
Intl.DateTimeFormat object
Using the toLocaleString()
means that you have to specify a locale
and options
each time you want a language-sensitive short name of the month. To use the same settings to format multiple dates, we can use an object of the Intl.DateTimeFormat class instead.
For example:
function getTwoConsecutiveMonthNames(monthNumber) {
const date1 = new Date();
date1.setMonth(monthNumber - 1);
const date2 = new Date();
date2.setMonth(monthNumber);
const formatter = new Intl.DateTimeFormat('en-US', { month: 'short' });
// Format both dates with the same locale and options
const firstMonth = formatter.format(date1);
const secondMonth = formatter.format(date2);
return `${firstMonth} & ${secondMonth}`;
}
console.log(getTwoConsecutiveMonthNames(1)); // Jan & Feb
console.log(getTwoConsecutiveMonthNames(2)); // Feb & Mar
console.log(getTwoConsecutiveMonthNames(3)); // Mar & Apr
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
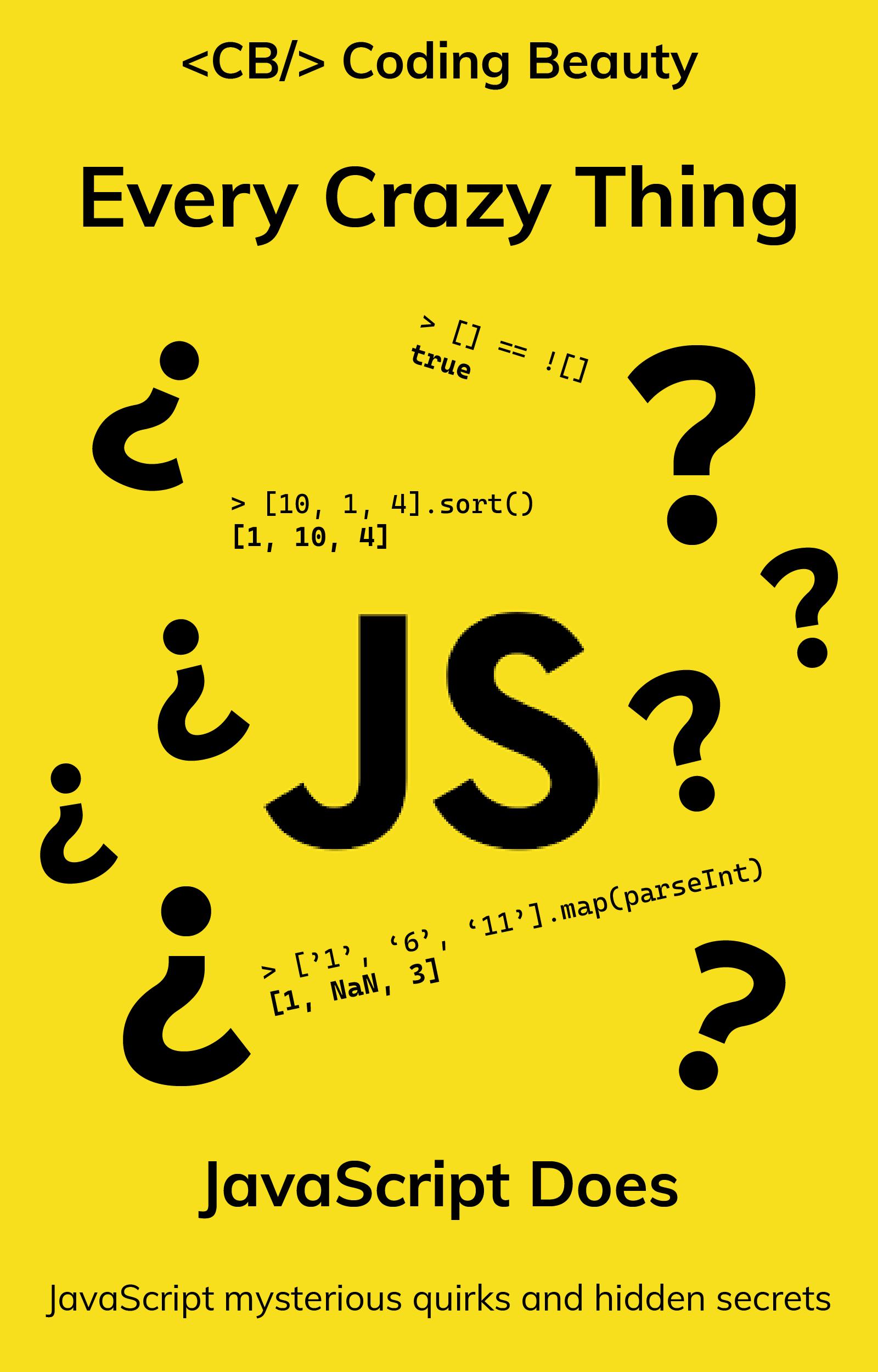