How to Simulate a KeyPress in JavaScript
To simulate a keypress in JavaScript:
- Get the text field element with a method like
document.querySelector()
. - Create a new
KeyboardEvent
object. - Call the
dispatchEvent()
method on the text field, with theKeyboardEvent
object.
i.e.:
function simulateKeyPress(key) {
const event = new KeyboardEvent('keydown', { key });
textField.dispatchEvent(event);
}
“Text field” here might be an input
element, textarea
element, or even a content editable div.
A KeyboardEvent
object describes a user’s interaction with the keyboard. Its constructor takes two arguments:
type
– a string with the name of the event. We pass'keydown'
to indicate that it’s the event of a key press.options
– an object used to set various properties of the event. We used the object’skey
to set the keyboard key name associated with our key press.
For example:
<input
type="text"
name="text-field"
id="text-field"
placeholder="Text field"
/>
<br />
Key presses: <span id="key-presses">0</span> <br /><br />
<button id="simulate">Simulate</button>
const textField = document.querySelector('#text-field');
const keyPresses = document.getElementById('key-presses');
const keyPressed = document.getElementById('key-pressed');
const simulate = document.getElementById('simulate');
// Set 'keydown' event listener, for testing purposes
let keyPressCount = 0;
textField.addEventListener('keydown', (event) => {
keyPressCount++;
keyPresses.textContent = keyPressCount;
keyPressed.textContent = event.key;
});
simulate.addEventListener('click', () => {
simulateKeyPress('a');
});
function simulateKeyPress(key) {
const event = new KeyboardEvent('keydown', { key });
textField.dispatchEvent(event);
}
First, we use selector methods like document.querySelector()
and document.getElementById()
to get an object representing the various elements to be used in the JavaScript code.
Then, we call the addEventListener()
method on the text field object to set an event listener for the keydown
event. When the user types a key or input is simulated from a script, the event listener will be fired, incrementing the key press count and displaying the most recently pressed key.
We also call the addEventListener()
method on the simulate button object for the click
event to simulate the key press when the user clicks the button.
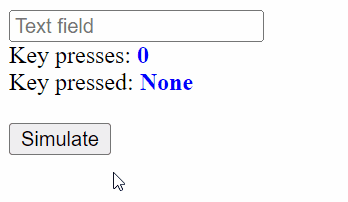
Notice that the key is not entered in the text field. This is because of the isTrusted property, which indicates whether a user action or script generated an event. When isTrusted
is false
, the event is not considered trusted and does not reflect visually in the text field. Browsers do this for security reasons.
const event = new KeyboardEvent('keydown', { key, ctrlKey: true });
console.log(event.isTrusted); // false
isTrusted
is a readonly property and will only be true
when an actual user’s action caused the event; in this case: typing on the keyboard.
Insert value into input field or textarea
If you want to enter a key into the text field programmatically, you’ll have to use other approaches. For input
and textarea
elements, here’s a method you can use:
function insertTextAtCursor(element, text) {
element.focus();
const startPos = element.selectionStart;
const endPos = element.selectionEnd;
element.value =
element.value.substring(0, startPos) +
text +
element.value.substring(endPos, element.value.length);
element.selectionStart = element.selectionEnd = startPos + text.length;
}
This function takes the text field element and some text as arguments and inserts the text into the text field, replacing any highlighted text before the insertion.
You can also use the insert-text-at-cursor
NPM package to insert text into an input field or textarea. Its default export function works like the one above.
import insertTextAtCursor from 'insert-text-at-cursor';
Either function can be called like this:
insertTextAtCursor(textField, 'Coding Beauty');
Simulate keypress in input field with key modifier
Sometimes, you might want to simulate a key combination that includes the modifier keys, e.g., Ctrl + A
, Ctrl + Shift + C
, etc. There are option properties you can set to do this.
For instance, setting the ctrlKey
property to true
simulates the Ctrl + {key}
combination, where {key}
is the value assigned to the key
property.
// Ctrl + c, Ctrl + o, Ctrl + d, Ctrl + e
const keysToSend = 'code';
let keyIndex = 0;
simulate.addEventListener('click', () => {
simulateKeyPress(keysToSend.at(keyIndex++) || keysToSend.at(-1));
});
function simulateKeyPress(key) {
const event = new KeyboardEvent('keydown', { key, ctrlKey: true });
textField.dispatchEvent(event);
}
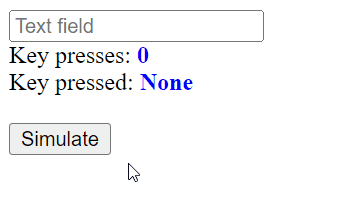
Ctrl
modifier.Some other important key modifier option properties are:
altKey
: adds theAlt
key to the key combination, e.g.,Alt + Z
,Ctrl + Alt + N
, etc.ctrlKey
: adds theCtrl
key to the key combination, e.g.,Ctrl + A
,Ctrl + Shift + T
, etc.shiftKey
: adds theShift
key to the key combination.metaKey
: adds the ⌘ key on MacOS and the Windows key on Windows to the key combination.
Key takeaways
We can easily simulate keypresses in JavaScript on a text field by calling the dispatchEvent()
method on the element object, passing a custom-created KeyboardEvent
object as an argument. While this will trigger the appropriate key events set on the element, it would not visually reflect on the text field since programmatically created events are not trusted for security reasons.