The “Unexpected strict mode reserved word ‘yield'” syntax error happens in JavaScript when you use the yield
keyword outside a generator function.
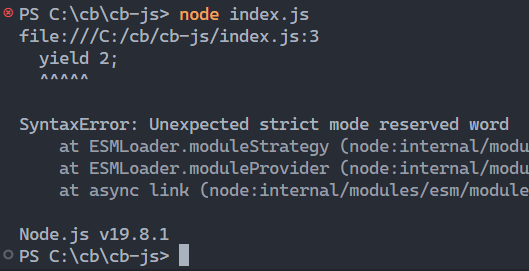
Here’s an example of the error happening:
function* numberGen() {
// ❌ SyntaxError: Unexpected strict mode reserved word 'yield'
yield 2;
yield 4;
}
const gen = numberGen();
console.log(gen.next().value);
console.log(gen.next().value);
Note: As this is a syntax error, we don’t need to call the function for it to happen, and no part of the code runs until we fix it.
To fix the “SyntaxError unexpected strict mode reserved word ‘yield'” error, ensure that the innermost enclosing function containing the yield
keyword is a generator function.
function* numberGen() {
// ✅ Runs successfully - no error
yield 2;
yield 4;
}
const gen = numberGen();
console.log(gen.next().value); // 2
console.log(gen.next().value); // 4
We create generator functions with function*
; the *
must be there.
Make sure innermost function is generator function
A common reason why the “Unexpected strict mode reserved word ‘yield'” happens is using yield
in an inner function that’s in a generator function.
This could be a callback or closure.
function* numberGen() {
return () => {
for (let i = 0; i < 30; i += 10) {
// ❌ SyntaxError: Unexpected strict mode reserved word 'yield'
yield i;
}
}
}
const gen = numberGen()();
console.log(gen.next().value);
console.log(gen.next().value);
You may think the outer function*
will let the yield
work, but nope – it doesn’t!
You have to make the innermost function a generator:
function numberGen() {
return function* () {
for (let i = 0; i < 30; i += 10) {
// ✅ Runs successfully - no error
yield i;
}
};
}
const gen = numberGen()();
console.log(gen.next().value); // 10
console.log(gen.next().value); // 20
Note we removed the *
from the outer one, so it acts like a normal function and returns the generator.
Also we change the arrow function a normal one, because arrow functions can’t be generators.
Here’s another example, seen here:
function* generator() {
const numbers = [1, 2, 3, 4, 5];
// ❌ SyntaxError: Unexpected strict mode reserved word
numbers.map((n) => yield(n + 1));
}
for (const n of generator()) {
console.log(n);
}
Here the goal was to consume the generator in the for loop, printing out each number one by one.
But the callback is the innermost function that has the yield
, and it’s not a generator. Let’s fix this:
function* generator() {
const numbers = [1, 2, 3, 4, 5];
// ✅ Runs successfully - no error
yield* numbers.map((n) => n + 1);
}
for (const n of generator()) {
console.log(n);
}
yield*
keyword
Wondering about the *
in the yield*
? It’s a shorter way of looping through the array and yield
ing each item.
A shorter way of this:
function* generator() {
const numbers = [1, 2, 3, 4, 5];
// ✅ Runs successfully - no error
for (const num of numbers.map((n) => n + 1)) {
yield num;
}
}
for (const n of generator()) {
console.log(n);
}
Key takeaways
The “Unexpected strict mode reserved word ‘yield'” error can happen in JavaScript when you mistakenly use the yield
keyword outside a generator function.
To fix this error, make sure that the innermost enclosing function containing the yield
keyword is actually a generator function.
One common reason for meeting this error is using yield
in an inner function that’s not a generator.
Remember, the yield*
syntax lets us easily loop through an array and yield each item. No need for a traditional for
loop with yield
statements.
By understanding and correctly applying these concepts, you can avoid the “Unexpected reserved word ‘yield'” error and ensure smooth execution of your generator functions in JavaScript. Happy coding!
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
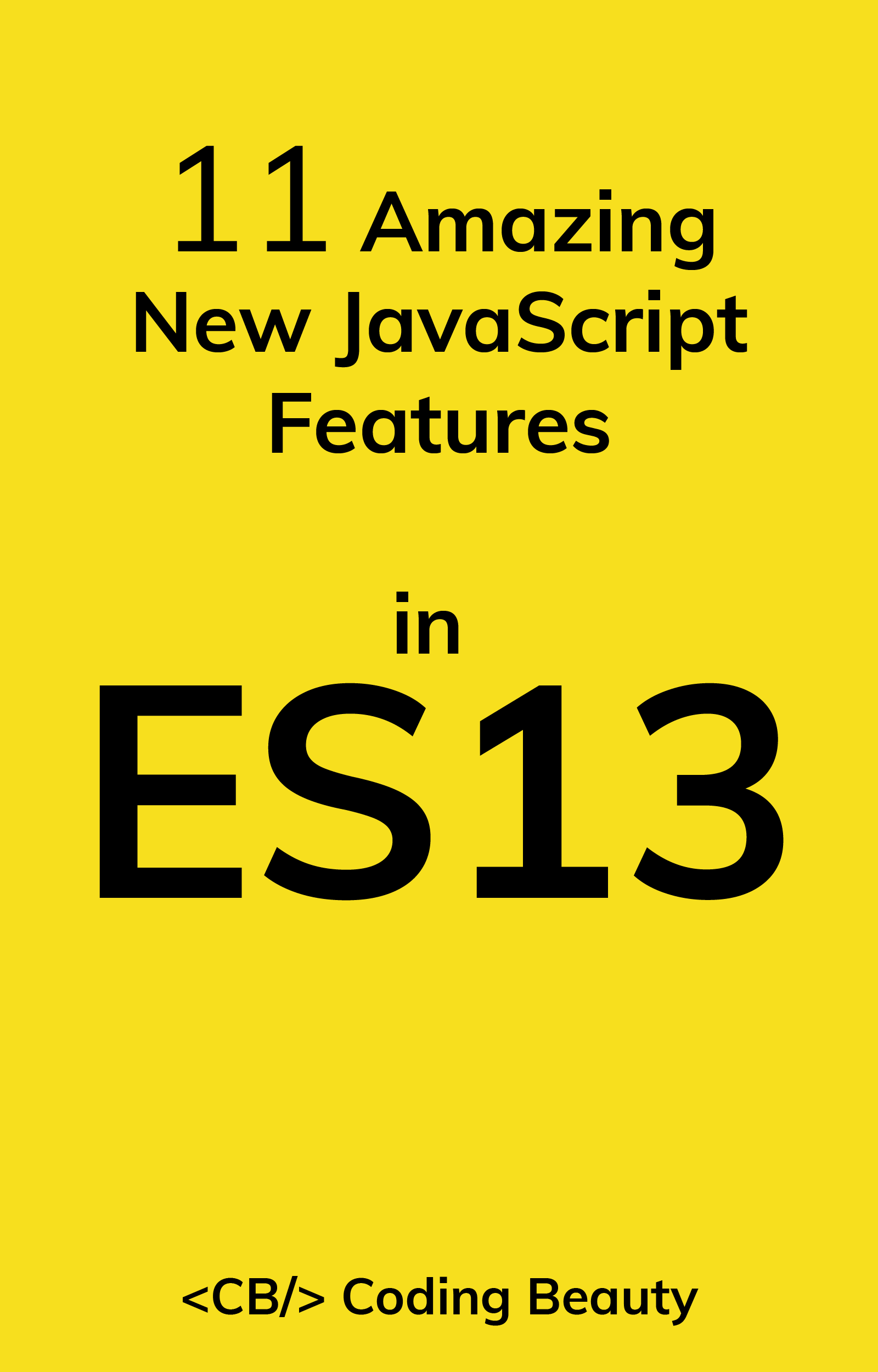