To scroll to the top of a div
element in React, create a ref for the div
, then call scrollTo()
on the ref.
import React, { useRef } from 'react';
function YourComponent() {
const divRef = useRef(null);
const onClick = () => {
divRef.current.scrollTo({ top: 0, behavior: 'smooth' });
};
return (
<div
ref={divRef}
style={{
height: '200px',
width: '400px',
overflow: 'auto',
border: '1px solid #c0c0c0',
}}
>
{[...Array(100)].map((_, index) => (
<div key={index}>Item {index + 1}</div>
))}
<button onClick={onClick}>Go to top</button>
</div>
);
}
export default function App() {
return (
<div>
<YourComponent />
</div>
);
}
Related: How to Scroll to the Bottom of a Div in React
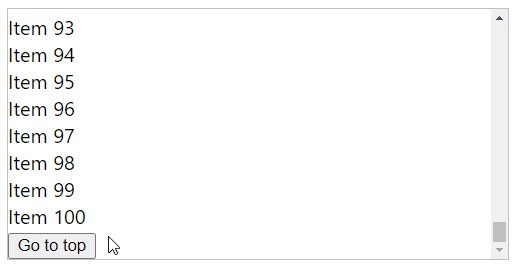
When reading long content, scroll-to-top helps users get back to the start easily.
Think of a lengthy blog post or an extensive list of items.
The useRef
hook from React plays a key role here.
It holds the reference to our scrollable div in divRef
.
The scrollTo
function accepts an object specifying the top
position and scroll behavior
.
This creates the scrollable effect due to the div’s fixed height and width.
The button at the end does the magic.
Clicking it triggers the onClick
function, smoothly scrolling the div to the top.
We enabled smooth scrolling by setting behavior
to smooth
in scrollTo()
.
In smooth scrolling, the scrolling happens gradually – an animation.
By default behavior
is auto
.
In auto
the scroll happens instantly.
A smooth scroll back to the top adds a slick, professional touch to the user experience.
Let’s dive deeper into a slightly different case in the next section.
How to scroll to the top of a div on item add in React
Imagine you have a list in a div.
This list is overflowing the div.
Each time a new item is added, we want to scroll back to the top.
React makes this quite easy:
import React, { useRef, useEffect } from 'react';
const MyComponent = () => {
const divRef = useRef(null);
const initialItems = Array.from({ length: 10 }, (_, i) => `Item ${i + 1}`);
const [items, setItems] = React.useState(initialItems);
const addItem = () => {
setItems([...items, `Item ${items.length + 1}`]);
};
useEffect(() => {
if (divRef.current) {
divRef.current.scrollTo({ top: 0, behavior: 'smooth' });
}
}, [items]);
return (
<div>
<button onClick={addItem}>Add Item</button>
<div
ref={divRef}
style={{
height: '200px',
width: '400px',
overflow: 'auto',
border: '1px solid #c0c0c0',
}}
>
{items.map((item, index) => (
<p key={index}>{item}</p>
))}
</div>
</div>
);
};
const App = () => {
return (
<div>
<MyComponent />
</div>
);
};
export default App;
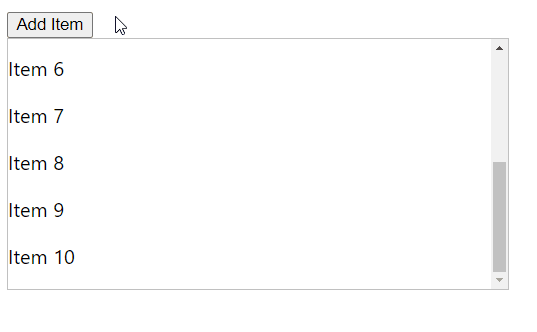
We use useRef
to create a new ref – divRef
.
This ref will be attached to our div.
The addItem
function adds new items to items
.
The useEffect
hook is activated every time items
is updated.
It takes care of scrolling the div to the top.
The component includes a button and the div.
Clicking the button adds a new item.
The div is linked to divRef
.
We display each item in items
as a paragraph.
That’s it.
With each new item added, the div scrolls to the top.
Give it a shot!
Scroll to top of page in React
To scroll to the top of a page in React, call window.scrollTo({ top: 0, left: 0})
.
import { useRef } from 'react';
const allCities = [
'Tokyo',
'New York City',
'Paris',
'London',
'Dubai',
'Sydney',
'Rio de Janeiro',
'Cairo',
'Singapore',
'Mumbai',
];
export default function App() {
const ref = useRef(null);
const scrollToTop = () => {
window.scrollTo({ top: 0, left: 0, behavior: 'smooth' });
};
return (
<div>
<h2>Top of the page</h2>
<div style={{ height: '100rem' }} />
<div ref={ref}>
{allCities.map((fruit) => (
<h2 key={fruit}>{fruit}</h2>
))}
</div>
<button onClick={scrollToTop}>Scroll to top</button>
<div style={{ height: '150rem' }} />
</div>
);
}
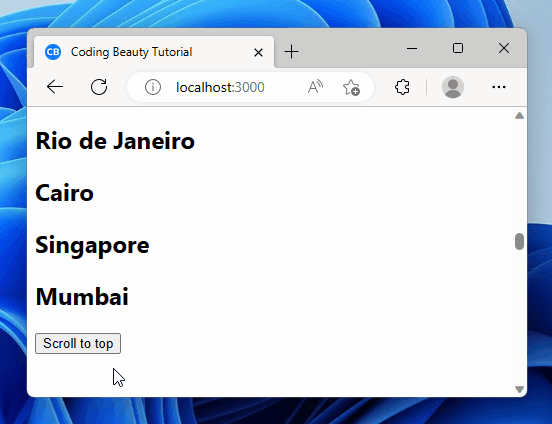
The window.scrollTo()
method scrolls to a particular position on a page.
const scrollToTop = () => {
window.scrollTo({ top: 0, left: 0, behavior: 'smooth' });
};
We could have called it with two arguments: window.scrollTo(0, 0)
.
But this overload doesn’t let us set a smooth
scroll behavior
.
When behavior
is smooth
, the browser scrolls to the top of the page in a gradual animation.
But when it’s auto
, the scroll happens instantly. We immediately jump to the top of the page.
We use the onClick
prop of the button to set a click
listener.
This listener will get called when the user clicks the button.
Key takeaways
- The
useRef
hook in React is essential for managing scrolling operations. This allows you to create a reference for your scrollable div. - With the
scrollTo()
function, you can easily scroll within your referenced div. - Adding new items to your list and wish to scroll back to the top of your div? React has got you covered!
- React’s
useEffect
hook comes to the rescue by triggering thescrollTo()
function each time your list of items is updated. - You can choose how you want your scrolling to behave – smoothly and gradually, or instantly for quick results.
- The
onClick
prop is perfect for activating your button click listener. A simple click, and you’re back at the top!
So, there you have it. Try implementing these in your upcoming React projects. Happy coding!
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
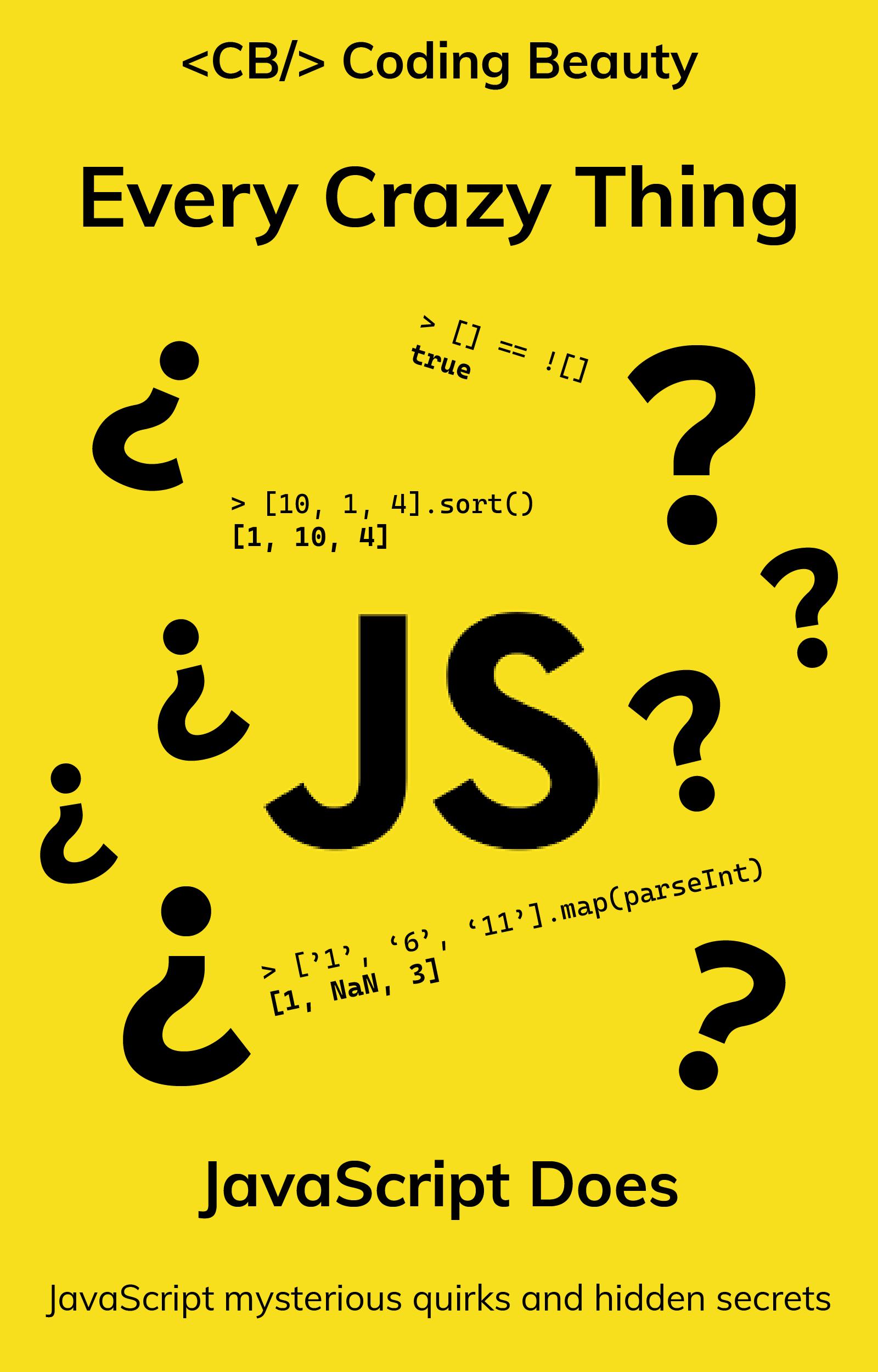