The “Unexpected reserved word ‘enum'” syntax error happens in JavaScript happens because enum
is not a valid keyword in JavaScript, but it is reserved.
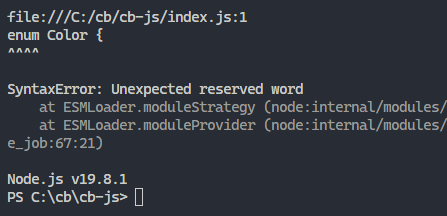
It was reserved in the ECMAScript specification in case it may become valid in the future.
If it wasn’t reserved, devs could start using it as variable names in their code – making future keyword use impossible!
// ❌ SyntaxError: Unexpected reserved word 'enum'
enum Color {
Red,
Blue,
Green
}
const color = Color.Red;
To fix it, represent the enumeration in another way, or change the file to TypeScript.
Fix: Create a JavaScript enum the right way
Enums are useful stuff, but since there’s no enum
keyword in JS, we’ll have to find others way to create and use them.
One powerful way to create an enumeration is with an object with JavaScript symbol properties:
const daysOfWeek = {
Mon: Symbol('Mon'),
Tue: Symbol('Tue'),
Wed: Symbol('Wed'),
Thu: Symbol('Thu'),
Fri: Symbol('Fri'),
Sat: Symbol('Sat'),
Sun: Symbol('Sun'),
};
const dayOfWeek = daysOfWeek.Tue;
With JavaScript symbols, we make sure that the constants are always different values, as every symbol is unique.
No need to worry duplicates – pretty convenient, right?
If we used this:
const daysOfWeek = {
Mon: 'Mon',
Tue: 'Tue',
Wed: 'Wed',
Thu: 'Thu',
Fri: 'Fri',
Sat: 'Sat',
Sun: 'Sun',
};
const dayOfWeek = daysOfWeek.Tue;
Someone could easily reassign the object’s properties to something else, giving us something more to worry about.
Fix: Just use TypeScript
Ah, TypeScript.
Alway there for us. Filled to the top with juicy modern stuff.
I mean enums aren’t so modern, but it’s one thing TS has that JS doesn’t.
Our first JS code runs flawlessly in TypeScript.
// ✅ No error - enums all the way!
enum Color {
Red,
Blue,
Green
}
const color = Color.Red;
So, if you really need that enum
keyword – even though much of the TypeScript community seems to frown on it – change that file extension.
Oh, it could even be that it’s TypeScript you wanted all along. As it happened here.
Here, this person was new to TypeScript on Node (we’ll all been there) and ran his TS file with the node
command directly.
Well, Node didn’t care about the extension and just treated the entire thing like a JavaScript file.
The easy solution was to compile it first with tsc
before running node
on the JS output. Or to use ts-node
on the TypeScript file directly.
Key takeaways
So, the few things to keep in mind on fixing the “Unexpected reserved keyword ‘enum'” error in JavaScript:
enum
is a no-go in JavaScript (for now): JavaScript doesn’t haveenum
. It’s reserved for future use.- Use objects with symbol properties: Want to create enumerations in JavaScript? Use objects with symbol properties.
- Symbols keep your code safe: Symbols are unique. They stop others from messing with your object’s properties.
- TypeScript has
enum
: Needenum
? Switch to TypeScript. It’s got your back. - Remember to compile TypeScript: Using TypeScript for Node.js? Compile your TypeScript files first. Or quickly use
ts-node
.
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
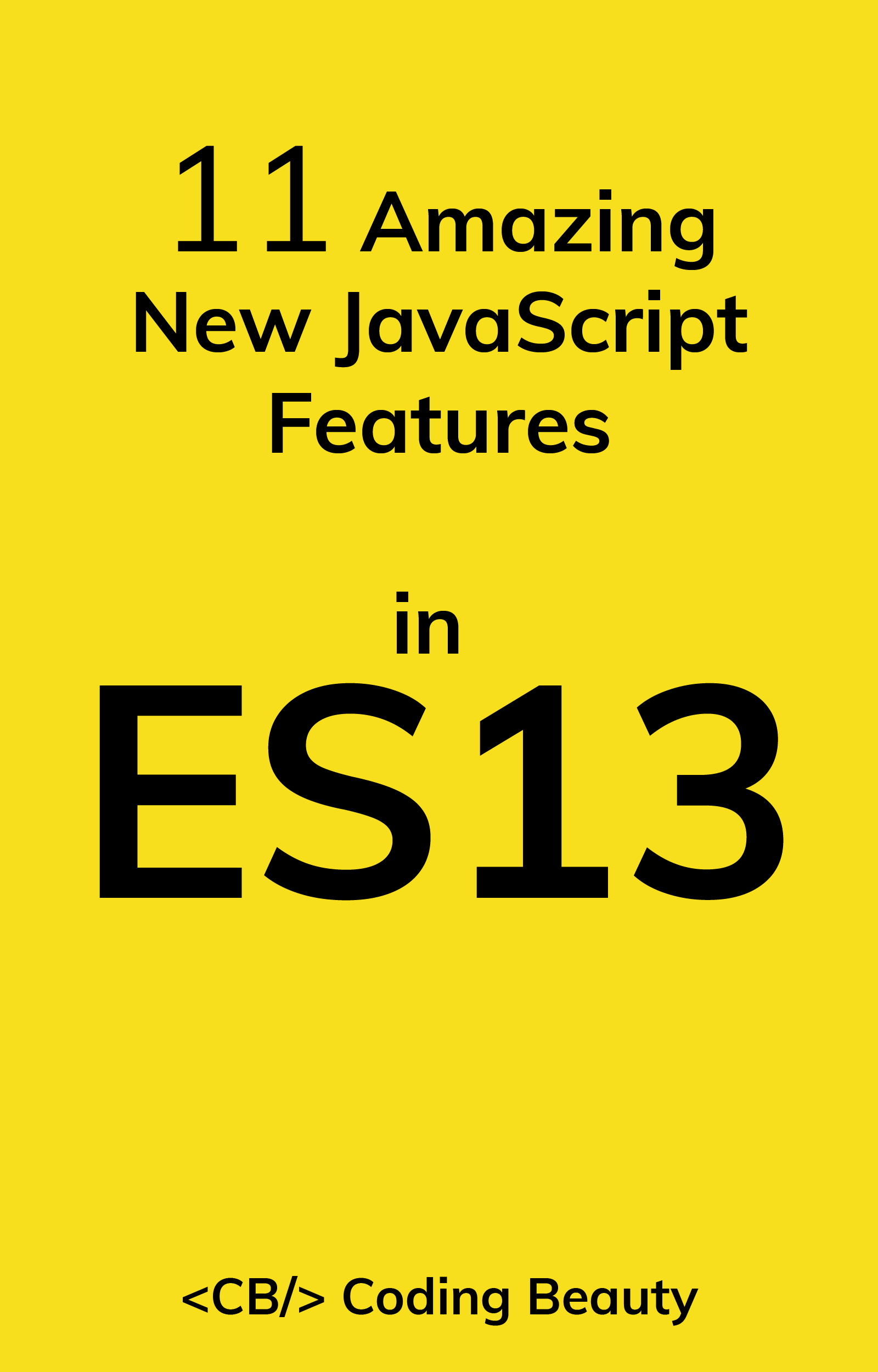