Getting the current route in a web application is important for managing user sessions, handling authentication, and dynamically displaying UI elements.
Let’s how see how easy it is to get the current route or pathname in our Next.js app.
In this article
- Get current route in Next.js Pages Router component
- Get current route in Next.js App Router component
- Get current route in Next.js getServerSideProps
Get current route in Next.js Pages Router component
We can get the current route in a Next.js component with the useRouter
hook:
import Head from 'next/head';
import { useRouter } from 'next/router';
export default function Page() {
const { pathname } = useRouter();
return (
<>
<Head>
<title>Next.js - Coding Beauty</title>
<meta name="description" content="Next.js Tutorials by Coding Beauty" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<link rel="icon" href="/favicon.ico" />
</Head>
<main>
Welcome to Coding Beauty
<br />
<br />
Pathname: <b>{pathname}</b>
</main>
</>
);
}
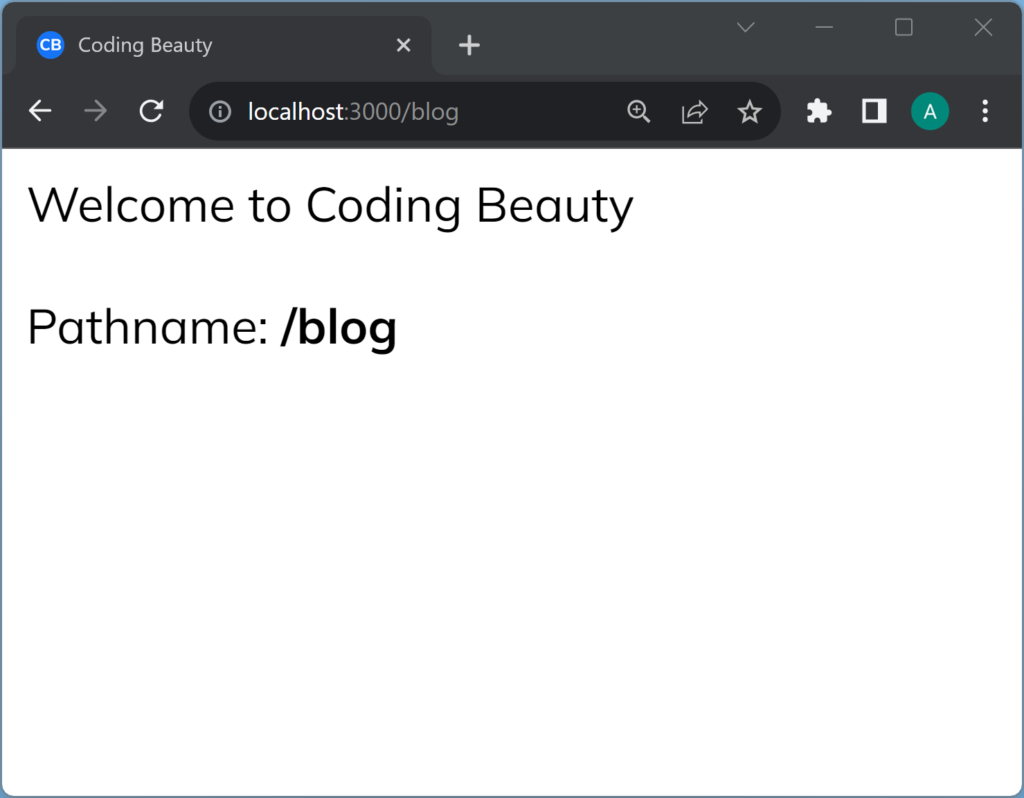
Get current route in Next.js App Router component
To get the current route in a Next.js app router component, use the usePathname
hook from next/navigation
:
'use client';
import React from 'react';
import { Metadata } from 'next';
import { usePathname } from 'next/navigation';
export const metadata: Metadata = {
title: 'Next.js - Coding Beauty',
description: 'Next.js Tutorials by Coding Beauty',
};
export default function Page() {
const pathname = usePathname();
return (
<main>
Welcome to Coding Beauty 😄
<br />
<br />
Route: <b>{pathname}</b>
</main>
);
}
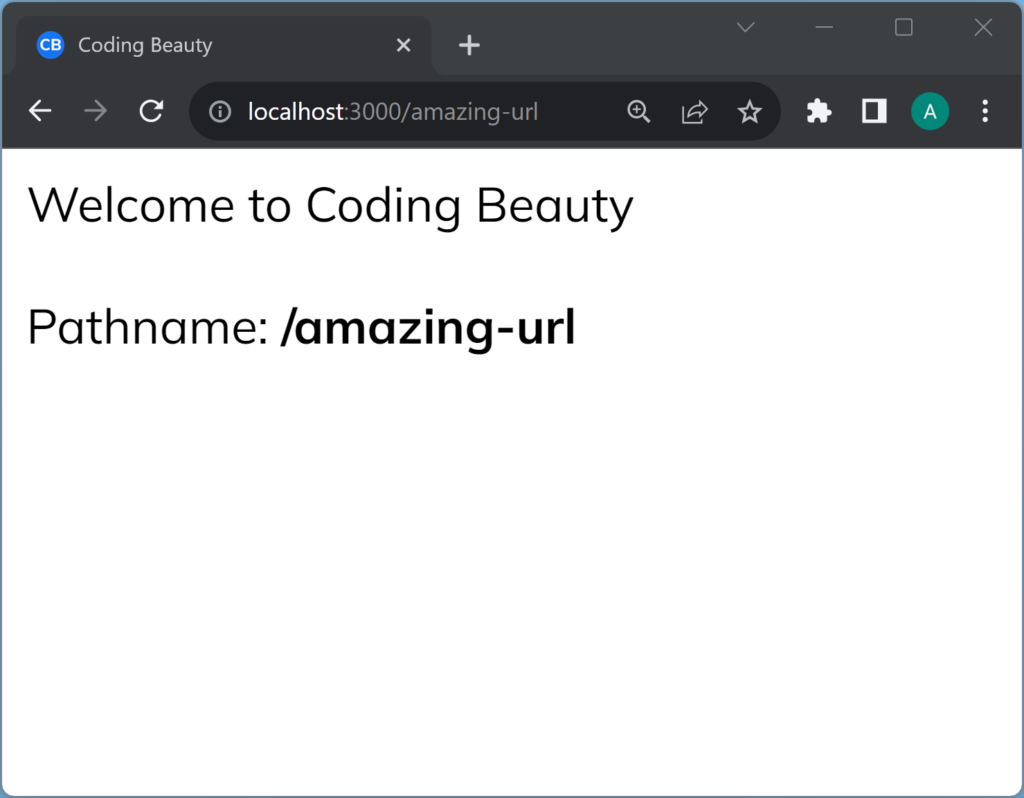
We need 'use client'
in Next.js 13 App Router
Notice the 'use client'
statement at the top.
It’s there because all components in the new app
directory are server components by default, which means we can’t access client-side specific APIs.
We’ll get an error if we try to do anything interactive with useEffect
or other hooks, as it’s a server environment.
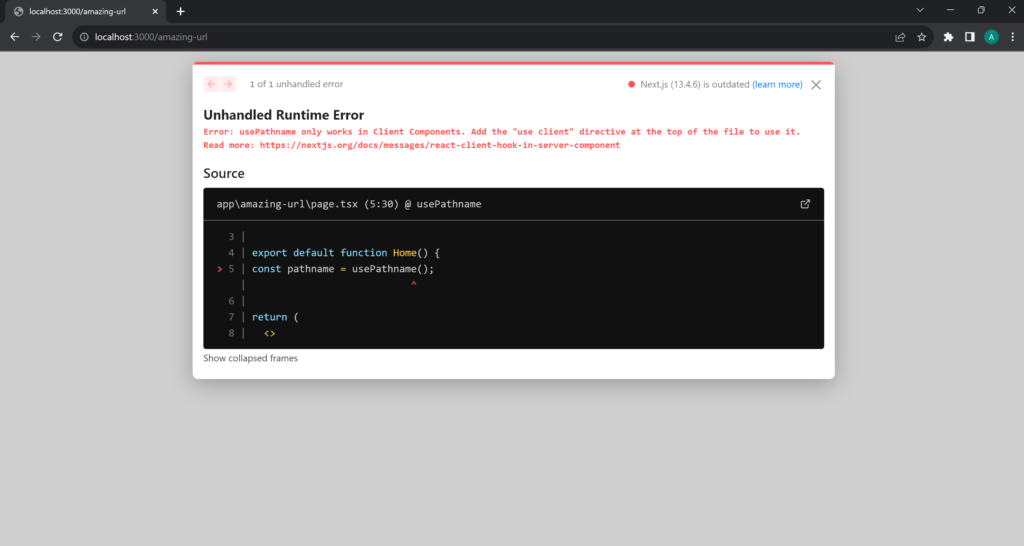
Get current route in Next.js getServerSideProps
To get the current route in getServerSideProps
, use req.url
from the context
argument.
import { NextPageContext } from 'next';
import Head from 'next/head';
export function getServerSideProps(context: NextPageContext) {
const route = context.req!.url;
console.log(`The route is: ${route}`);
return {
props: {
route,
},
};
}
export default function Page({ route }: { route: string }) {
return (
<>
<Head>
<title>Next.js - Coding Beauty</title>
<meta name="description" content="Generated by create next app" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<link rel="icon" href="/favicon.png" />
</Head>
<main>
Welcome to Coding Beauty 😃
<br />
Route: <b>{route}</b>
</main>
</>
);
}
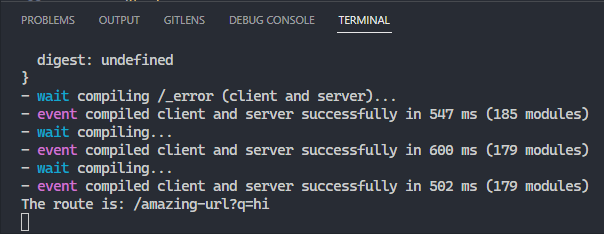
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
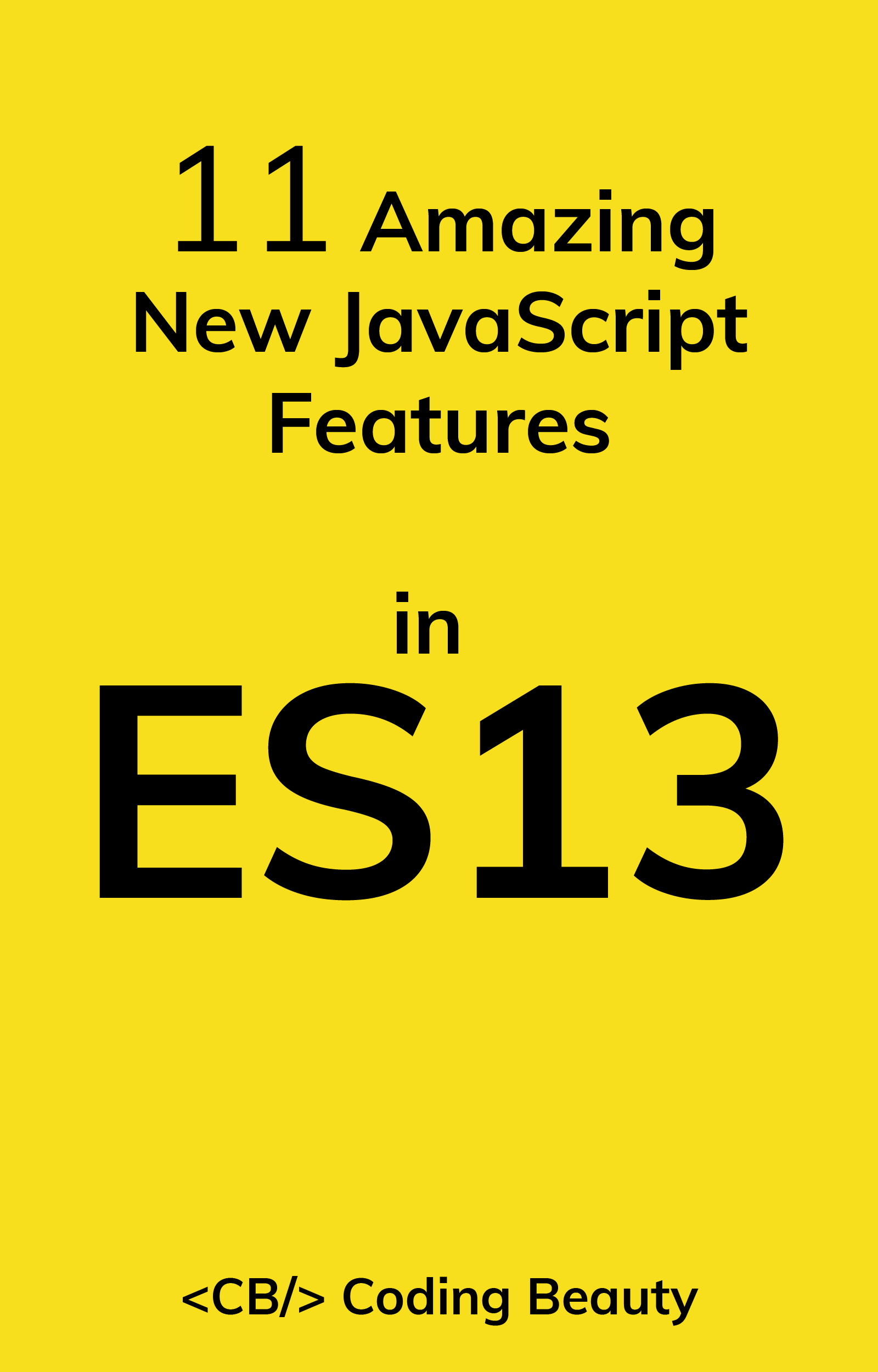