How to Fix the “Unknown file extension .ts” Error in ts-node
The “Unknown file extension .ts” error occurs in ts-node
occurs when "type": "module"
is set in your package.json
file. To fix it, run the TypeScript file with ts-node --esm my-file.ts
, or remove "type": "module"
from your package.json
file.
For example, in a project with this package.json
file:
{
"name": "cb-js",
"version": "1.0.0",
"main": "index.js",
"type": "module",
"license": "MIT",
"devDependencies": {
"prettier": "^2.8.1"
}
}
If you have a TypeScript file in your project, e.g., my-file.ts
:
const num: number = 10;
console.log(num ** 2);
Running the ts-node index.ts
command will result in the ERR_UNKNOWN_FILE_EXTENSION TypeError
in ts-node:
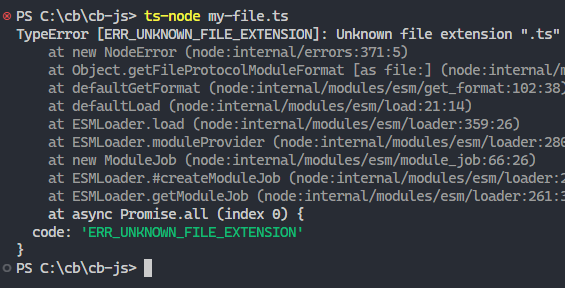
To fix the “Unknown file extension ‘.ts'” error in ts-node
, run ts-node
with the --esm
option set:
ts-node --esm my-file.ts
# Or
ts-node-esm my-file.ts
# Or
node --loader ts-node/esm my-file.ts
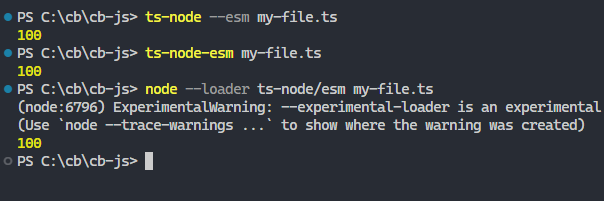
ts-node
in esm
mode.To avoid using the --esm
flag, add the following to your tsconfig.json
file:
{
// other settings...
"ts-node": {
"esm": true,
"experimentalSpecifierResolution": "node"
}
}
After doing this, you’ll be able to run the TypeScript file with only ts-node
:
ts-node my-file.ts
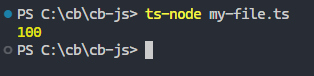
ts-node
.You may also need to add one or more of the following options to your tsconfig.json
file:
{
// other settings..
"compilerOptions": {
// other compiler options...
"esModuleInterop": true,
"module": "ESNext", // "module": "CommonJS" should work too
"moduleResolution": "Node"
},
"include": ["/**/*.ts"],
"exclude": ["node_modules"]
}
Remove "type": "module"
from tsconfig.json
file
Alternatively, instead of doing all of the above, you can simply remove the "type": "module"
field from your package.json
file to fix the ERR_UNKNOWN_FILE_EXTENSION error in ts-node.
{
"name": "cb-js",
"version": "1.0.0",
"main": "index.js",
// removed: "type": "module",
"license": "MIT",
"devDependencies": {
"prettier": "^2.8.1"
}
}
And you’ll be able to run the TypeScript file with ts-node
successfully.
Compile TypeScript files into JavaScript
Another way to avoid ERR_UNKNOWN_FILE_EXTENSION is to stop using ts-node
altogether. Instead, you can compile your TypeScript files with tsc
and run the JavaScript output with node
– the classic way.
# Compile with tsc
npx tsc --outDir dist my-file.ts
# Run with node
node dist/my-file.ts
Basic TypeScript compilation setup
Here’s a great way to set up your project for easily compiling and debugging TypeScript files.
First, install the TypeScript compiler:
npm i tsc
Then specify the src dir and out dir in tsconfig.json
, and enable source maps for seamless Typescript debugging:
{
"compilerOptions": {
// ... other options
"rootDir": "src", // Location of TypeScript files
"outDir": "dist", // Location of compiled JavaScript files
"sourceMap": true // Generate sourcemaps
}
}
Finally, create a start
NPM script that automatically runs the tsc
and node
commands one after the other:
{
// ... other options
"scripts": {
"start": "tsc && node index.js"
}
}
Now you can run the script easily with npm start
.
And you can debug TypeScript in VSCode too.