How to Get the Mouse Position in React
To get the position of the mouse in React, add a mousemove
event handler to the window
object and access the clientX
and clientY
properties of the MouseEvent
object to get the X and Y coordinates of the mouse respectively.
For example:
App.js
import { useEffect, useState } from 'react';
export default function App() {
const [mousePos, setMousePos] = useState({});
useEffect(() => {
const handleMouseMove = (event) => {
setMousePos({ x: event.clientX, y: event.clientY });
};
window.addEventListener('mousemove', handleMouseMove);
return () => {
window.removeEventListener(
'mousemove',
handleMouseMove
);
};
}, []);
return (
<div>
The mouse is at position{' '}
<b>
({mousePos.x}, {mousePos.y})
</b>
</div>
);
}
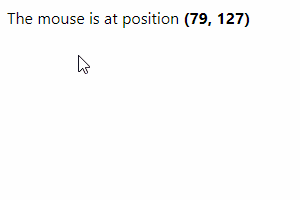
The mousemove
event is triggered on an element when the mouse hovers on it. To be more precise, it is fired when the mouse is moved and the hotspot of the cursor is within the bounds of the element.
We attach the event listener to the window
object to trigger the event whenever the mouse is moved anywhere in the page.
We passed an empty dependencies array to the useEffect hook because we want to add a handler for the mousemove
event only once – when the component mounts.
In the cleanup function, we use the removeEventListener()
method to remove the event listener previously added and prevent a memory leak when the component is unmounted.
The mousemove
event listener receives a MouseEvent object used to access information and perform actions related to the event. We use the clientX
and clientY
properties of this object to get the position of the mouse on the X-coordinate and Y-coordinate respectively in the viewport of the application.
Get mouse position relative to element in React
In the previous example we were able to get the mouse position in global coordinates.
In global coordinates position (0,0) is at the top left of the webpage and position (Xmax, Ymax) is at the bottom right.
We might instead want to get the mouse position within the region of an element.
To get the mouse position relative to an element in React, set an onMouseMove
event handler on the element, then calculate the local X and Y position using properties of the MouseEvent
object passed to the event handler.
For example:
App.js
import { useEffect } from 'react';
import { useState } from 'react';
export default function App() {
const [globalMousePos, setGlobalMousePos] = useState({});
const [localMousePos, setLocalMousePos] = useState({});
const handleMouseMove = (event) => {
// 👇 Get mouse position relative to element
const localX = event.clientX - event.target.offsetLeft;
const localY = event.clientY - event.target.offsetTop;
setLocalMousePos({ x: localX, y: localY });
};
useEffect(() => {
const handleMouseMove = (event) => {
setGlobalMousePos({
x: event.clientX,
y: event.clientY,
});
};
window.addEventListener('mousemove', handleMouseMove);
return () => {
window.removeEventListener(
'mousemove',
handleMouseMove
);
};
}, []);
return (
<div>
<div
style={{
border: '1px solid gray',
display: 'inline-block',
padding: '75px',
textAlign: 'center',
}}
onMouseMove={handleMouseMove}
>
Local
<br />
<b>
({localMousePos.x}, {localMousePos.y})
</b>
</div>
<br />
Global
<br />
<b>
({globalMousePos.x}, {globalMousePos.y})
</b>
</div>
);
}
Now the resulting X and Y coordinates will be relative to the element. For example, position (0,0) will be at the top left of the element, not the page:
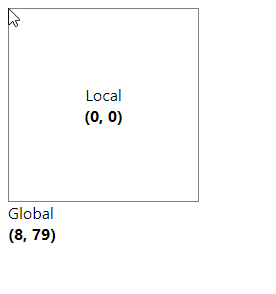
We subtract the offsetLeft
property of the element from the clientX
property of the MouseEvent
object to get the X position relative to the element.
Similarly, to get the Y position, we subtract the offsetTop
property of the element from the clientY
property of the MouseEvent
object.
const handleMouseMove = (event) => {
// Calculate position within bounds of element
const localX = event.clientX - event.target.offsetLeft;
const localY = event.clientY - event.target.offsetTop;
setLocalMousePos({ x: localX, y: localY });
};
The offsetLeft property returns the number of pixels between the left position of an element and that of its parent.
Likewise, the offsetTop property returns the number of pixels between the top position of an element and that of its parent.