To add a class conditionally to an element in Vue, set the class
prop to a JavaScript object where for each property, the key is the class name, and the value is the boolean condition that must be true for the class to be set on the element.
<p
v-bind:class="{
'class-1': class1,
'class-2': class2,
}"
>
Coding
</p>
Here’s a complete example:
App.vue
<template>
<div id="app">
<input
type="checkbox"
name="class-1"
v-model="class1"
/>
<label for="class-1">Class 1</label>
<br />
<input
type="checkbox"
name="class-2"
v-model="class2"
/>
<label for="class-2">Class 2</label>
<!-- 👇 Add classes conditionally -->
<p
v-bind:class="{
'class-1': class1,
'class-2': class2,
}"
>
Coding
</p>
<p
v-bind:class="{
'class-1': class1,
'class-2': class2,
}"
>
Beauty
</p>
</div>
</template>
<script>
export default {
data() {
return {
class1: false,
class2: false,
};
},
};
</script>
<style>
.class-1 {
font-size: 2em;
font-weight: bold;
}
.class-2 {
color: blue;
text-transform: uppercase;
}
</style>
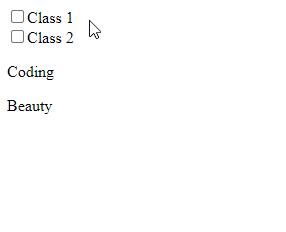
The class-1
class will only present on the element if the class1
variable is true
, and the class-2
class will only be present if the class2
variable is true
. The values of these variables are determined by the current checked state of their respective checkboxes since we use v-model
to set up a two-way binding between the variables and the checkboxes.
Use :class
shorthand
We can use :class
as a shorthand for v-bind:class
.
<p
:class="{
'class-1': class1,
'class-2': class2,
}"
>
Coding
</p>
<p
:class="{
'class-1': class1,
'class-2': class2,
}"
>
Beauty
</p>
Pass object as computed property
The JavaScript object passed doesn’t have to be inline. It can be stored as a computed property in the Vue component instance.
<template>
<div id="app">
...
<!-- 👇 Add classes conditionally -->
<p :class="classObject">Coding</p>
<p :class="classObject">Beauty</p>
</div>
</template>
<script>
export default {
data() {
return {
class1: false,
class2: false,
};
},
computed: {
// 👇 Computed object property
classObject() {
return {
'class-1': this.class1,
'class-2': this.class2,
};
},
},
};
</script>
...
Add both static and dynamic classes
We can set the class
prop on the same element twice, once to add static classes, and once to add the dynamic classes that will be present based on certain conditions.
For example:
<template>
<div id="app">
<input
type="checkbox"
name="class-1"
v-model="class1"
/>
<label for="class-1">Class 1</label>
<br />
<input
type="checkbox"
name="class-2"
v-model="class2"
/>
<label for="class-2">Class 2</label>
<!-- 👇 Add classes conditionally and statically -->
<p
class="static-1 static-2"
:class="{ 'class-1': class1, 'class-2': class2 }"
>
Coding
</p>
<p
class="static-1 static-2"
:class="{ 'class-1': class1, 'class-2': class2 }"
>
Beauty
</p>
</div>
</template>
<script>
export default {
data() {
return {
class1: false,
class2: false,
};
},
};
</script>
<style>
.class-1 {
font-size: 2em;
font-weight: bold;
}
.class-2 {
color: blue;
text-transform: uppercase;
}
/* 👇 Classes to add statically */
.static-1 {
font-family: 'Segoe UI';
}
.static-2 {
font-style: italic;
}
</style>
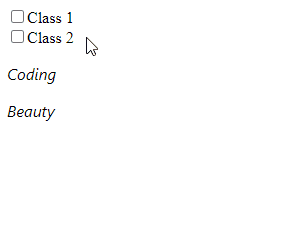
The static-1
and static-2
classes are always applied to the texts, making them italic and changing the font.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
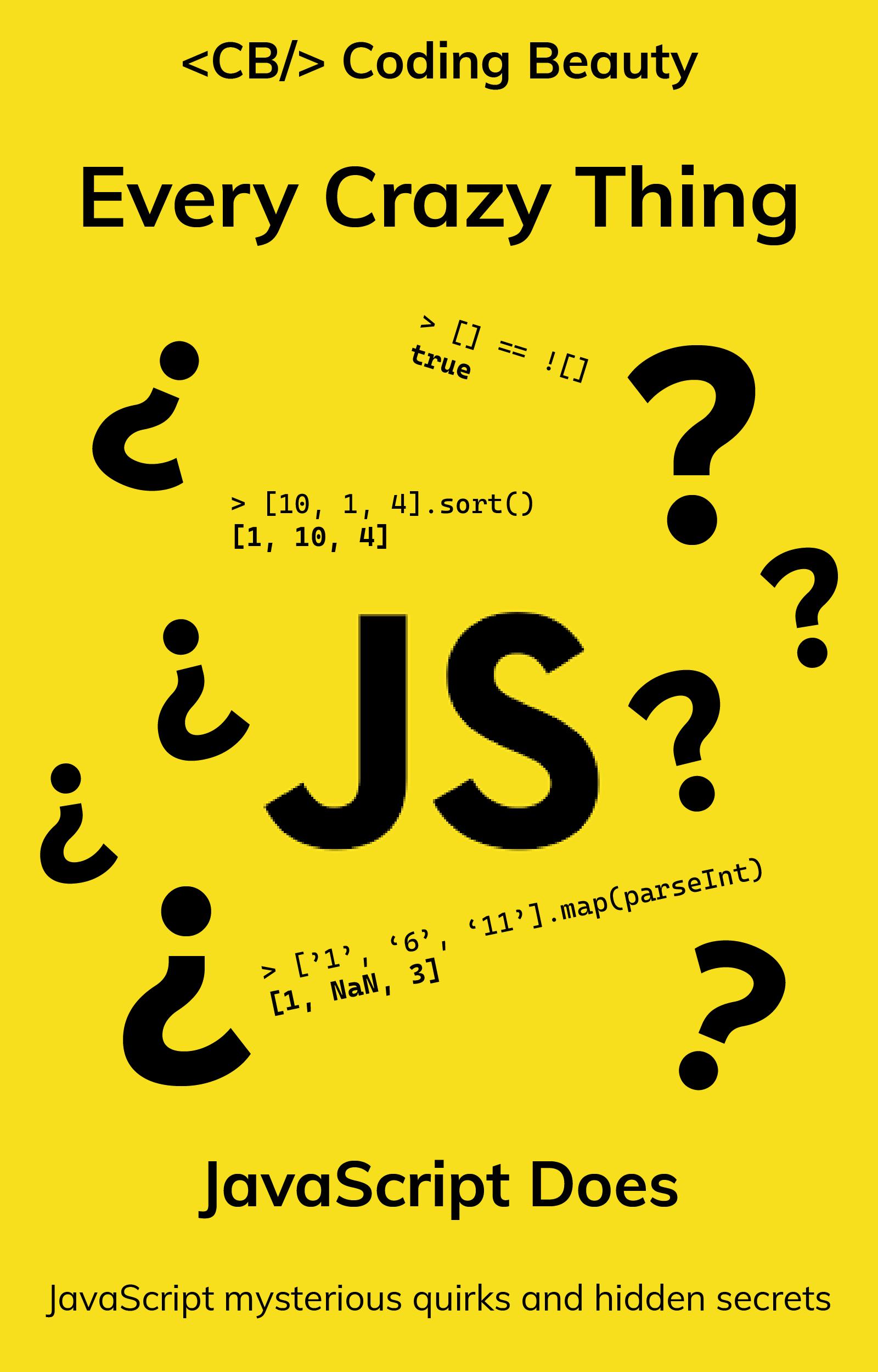