A switch allows a user to choose between two distinct values.
You can use them in place of a checkbox in your UI.
In this article, we’re going to learn about the Vuetify switch component and the different ways of customizing it.
The v-switch
component
v-switch
is the name of the component Vuetify provides for creating a switch:
<template>
<v-app>
<div class="d-flex justify-center mt-2">
<v-switch></v-switch>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>

Clicking the switch turns it on:

Two-way binding with v-model
We can set up a two-way binding between the value of the switch and a variable with v-model
. In the code below, we’ve created a switch and a button below it for turning it off. We added a click handler to the button that will set switch1
to false
, which will turn the switch off due to the v-model
. The two-way binding
also ensures that toggling the switch in the user interface will update switch1
.
<template>
<v-app>
<div class="d-flex justify-center mt-2">
<v-switch v-model="switch1"></v-switch>
</div>
<div class="d-flex justify-center mt-2">
<v-btn color="primary" @click="switch1 = false">Turn off</v-btn>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
switch1: false,
}),
};
</script>

Clicking the button turns off the switch:
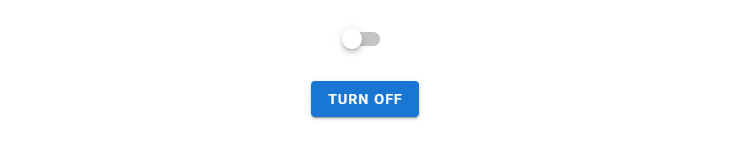
Switch labels
We can explain the function of a switch to users with the label
prop:
<template>
<v-app>
<div class="d-flex justify-center mt-2">
<v-switch
v-model="switch1"
:label="`Switch: ${switch1 ? 'on' : 'off'}`"
></v-switch>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
switch1: false,
}),
};
</script>


Custom HTML labels
To include HTML in the label of a switch, use the label
slot:
<template>
<v-app>
<div class="d-flex justify-center mt-2">
<v-switch v-model="switch1">
<template v-slot:label>
Switch:
<b :class="{ 'primary--text': switch1 }">{{
switch1 ? 'on' : 'off'
}}</b>
</template>
</v-switch>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
switch1: false,
}),
};
</script>


Custom colors
Like many other Vuetify components, v-switch
comes with the color
prop which allows us to set a custom color:
<template>
<v-app>
<div class="d-flex justify-center mt-2">
<v-col sm="4" class="d-flex justify-center"
><v-switch color="red" label="red"></v-switch>
</v-col>
<v-col sm="4" class="d-flex justify-center"
><v-switch color="primary" label="primary"></v-switch>
</v-col>
<v-col sm="4" class="d-flex justify-center"
><v-switch color="green" label="green"></v-switch>
</v-col>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>

Flat switch
We can use the flat prop to remove the elevation on the thumb of a switch.
<template>
<v-app>
<div class="d-flex justify-center mt-2">
<v-switch
v-model="switch1"
:label="`Switch: ${switch1 ? 'on' : 'off'}`"
flat
></v-switch>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
switch1: false,
}),
};
</script>


Switch in inset mode
To render a switch in inset mode, set the inset
prop to true
:
<template>
<v-app>
<div class="d-flex justify-center mt-2">
<v-switch
v-model="switch1"
:label="`Switch: ${switch1 ? 'on' : 'off'}`"
inset
></v-switch>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
switch1: false,
}),
};
</script>


Pass array to v-model
We can pass a shared array variable to the v-model
s of multiple switch components.
<template>
<v-app>
<div class="d-flex justify-center mt-2">
{{ people }}
</div>
<div class="d-flex justify-center mt-2">
<v-switch
v-model="people"
color="primary"
label="Peter"
value="Peter"
></v-switch>
</div>
<div class="d-flex justify-center mt-2">
<v-switch
v-model="people"
color="primary"
label="Daniel"
value="Daniel"
></v-switch>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
people: [],
}),
};
</script>
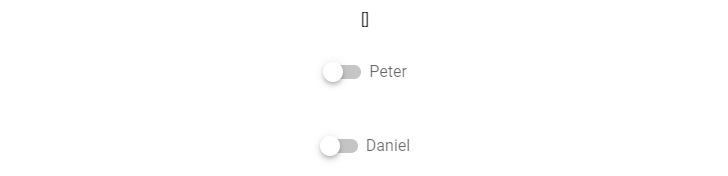
Toggling any one of the switches will update the array:
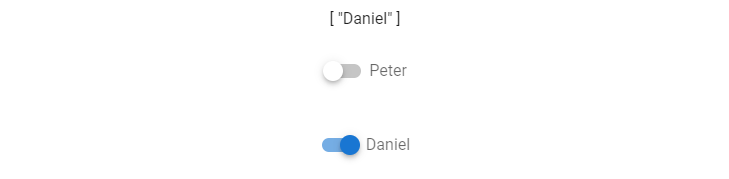
Disabled switch
We can use the disabled
prop to disable a switch:
<template>
<v-app>
<div class="d-flex justify-center mt-2">
<v-switch label="off disabled" :value="false" disabled></v-switch>
</div>
<div class="d-flex justify-center mt-2">
<v-switch label="on disabled" :value="true" disabled></v-switch>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
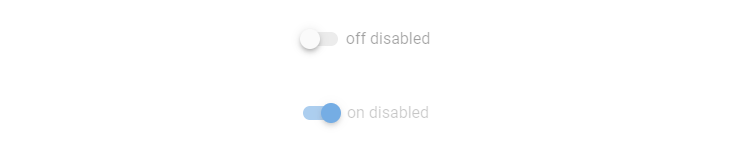
Switch in loading state
Use the loading
prop to set a switch to the loading state:
<template>
<v-app>
<div class="d-flex justify-center mt-2">
<v-switch label="off loading" :value="false" loading="primary"></v-switch>
</div>
<div class="d-flex justify-center mt-2">
<v-switch label="on loading" :value="true" loading="primary"></v-switch>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
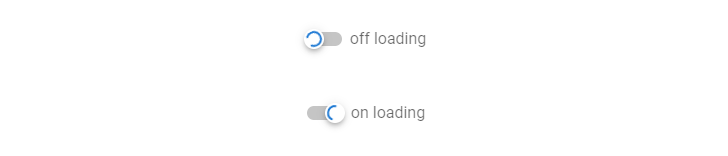
Key takeaways
Switches are useful for taking boolean input from users.
Use the v-switch
component and its various props to create and customize them.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
