The Vuetify rating component is useful for collecting user feedback. Users can use it to indicate their level of satisfaction with certain content. Read on to find out more about this component and the various ways in which we can customize it.
The Vuetify Rating Component (v-rating)
We use v-rating
to create a rating component.
<template>
<v-app>
<div class="d-flex ma-2 justify-center">
<v-rating></v-rating>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>

The user can indicate various levels of satisfaction by clicking on one of the items.

Vuetify Rating Value
We use the value prop to programmatically set the number of selected items on the rating component. The default is 0
.
<template>
<v-app>
<div class="text-center ma-2">
<v-rating></v-rating>
<v-rating :value="3"></v-rating>
<v-rating :value="5"></v-rating>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
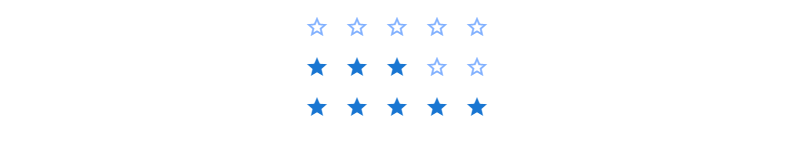
Vuetify Rating v-model
We can use v-model
to set up a two-way binding between the value
of the v-rating
and a variable. In the code example below, we use v-model
to create a rating component with text below it displaying the current value
:
<template>
<v-app>
<div class="d-flex ma-2 justify-center">
<v-rating v-model="rating"></v-rating>
</div>
<div class="d-flex ma-2 justify-center">
Rating: {{ rating }}
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
rating: 0,
}),
};
</script>

We can also use the two-way binding to add a button that changes the rating when clicked:
<template>
<v-app>
<div class="d-flex ma-2 justify-center">
<v-rating v-model="rating"></v-rating>
</div>
<div class="d-flex ma-2 justify-center">
Rating: {{ rating }}
</div>
<div class="d-flex ma-2 justify-center">
<v-btn
@click="rating = 5"
color="primary"
>
5 stars
</v-btn>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
rating: 0,
}),
};
</script>
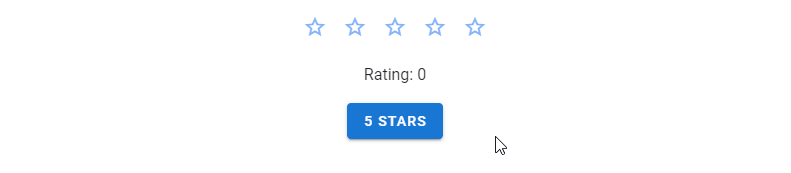
Readonly
We can turn off interactivity with the v-rating
component with the readonly
prop.
<template>
<v-app>
<div class="text-center ma-2">
<v-rating
:value="3"
readonly
></v-rating>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>

Vuetify Rating Colors
v-rating
comes with the color
and background-color
props for customizing its color. We can use any color from the Material design spec.
The color
prop sets the color of the selected items, while the background-color
prop sets the color of the unselected items.
<template>
<v-app>
<div class="text-center">
<v-rating
v-model="rating"
background-color="purple accent-4"
color="purple"
>
</v-rating>
<v-rating
v-model="rating"
background-color="primary"
color="pink"
>
</v-rating>
<v-rating
v-model="rating"
background-color="green"
color="orange"
>
</v-rating>
<v-rating
v-model="rating"
background-color="yellow darken-3"
color="green"
>
</v-rating>
<v-rating
v-model="rating"
background-color="red"
color="red"
>
</v-rating>
<v-rating
v-model="rating"
background-color="indigo"
color="indigo"
></v-rating>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
rating: 3,
}),
};
</script>
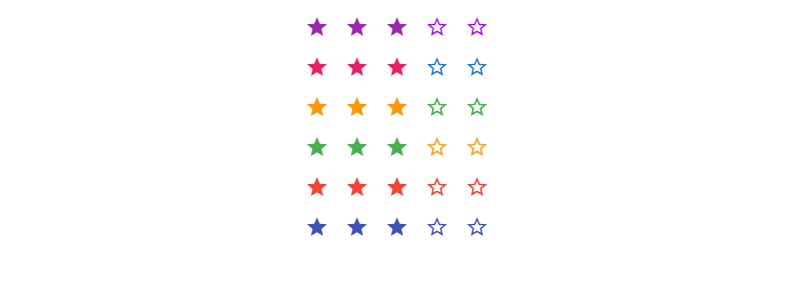
Vuetify Rating Length
We can use the length
prop to set the number of rating levels we want the rating component to have.
<template>
<v-app>
<div class="text-center ma-2">
<v-rating
:value="3"
color="yellow darken-3"
background-color="grey darken-1"
></v-rating>
<v-rating
length="6"
:value="3"
color="yellow darken-3"
background-color="grey darken-1"
></v-rating>
<v-rating
length="7"
:value="4"
color="yellow darken-3"
background-color="grey darken-1"
></v-rating>
<v-rating
length="8"
:value="4"
color="yellow darken-3"
background-color="grey darken-1"
></v-rating>
<v-rating
length="9"
:value="5"
color="yellow darken-3"
background-color="grey darken-1"
></v-rating>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
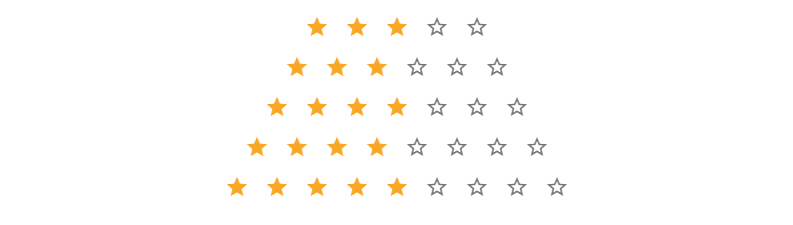
Vuetify Rating Hover
Setting the hover
prop makes the v-rating
display visual feedback when the user hovers over it with the mouse.
<template>
<v-app>
<div class="text-center ma-2">
<v-rating hover></v-rating>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
rating: 3,
}),
};
</script>

Vuetify Rating Half Increments
Use the half-increments
prop to allow selection of half rating level increments.
<template>
<v-app>
<div class="text-center ma-2">
<v-rating
hover
half-increments
color="yellow darken-3"
background-color="grey darken-1"
:value="0.5"
></v-rating>
<v-rating
hover
half-increments
color="yellow darken-3"
background-color="grey darken-1"
:value="2.5"
></v-rating>
<v-rating
hover
half-increments
color="yellow darken-3"
background-color="grey darken-1"
:value="4.5"
></v-rating>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>

Vuetify Rating Size
We can customize the size of the rating component with the same classes available in the icon component (v-icon
).
<template>
<v-app>
<div class="text-center ma-2">
<v-rating
hover
color="yellow darken-3"
background-color="grey darken-1"
:value="3"
x-small
></v-rating>
<v-rating
hover
color="yellow darken-3"
background-color="grey darken-1"
:value="3"
small
></v-rating>
<v-rating
hover
color="yellow darken-3"
background-color="grey darken-1"
:value="3"
medium
></v-rating>
<v-rating
hover
color="yellow darken-3"
background-color="grey darken-1"
:value="3"
large
></v-rating>
<v-rating
hover
color="yellow darken-3"
background-color="grey darken-1"
:value="3"
x-large
></v-rating>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
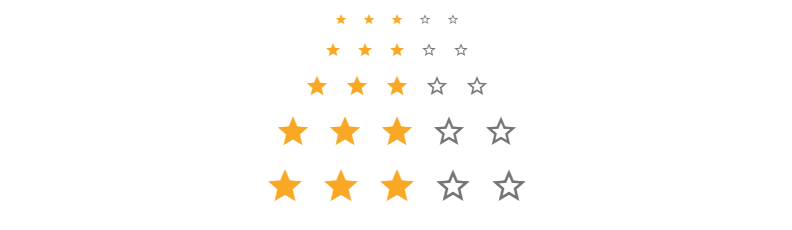
We can also customize the size by setting the size
prop to a numerical value:
<template>
<v-app>
<div class="text-center ma-2">
<v-rating
hover
color="yellow darken-3"
background-color="grey darken-1"
:value="3"
v-for="num in 5"
:key="num"
:size="num * 10"
></v-rating>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
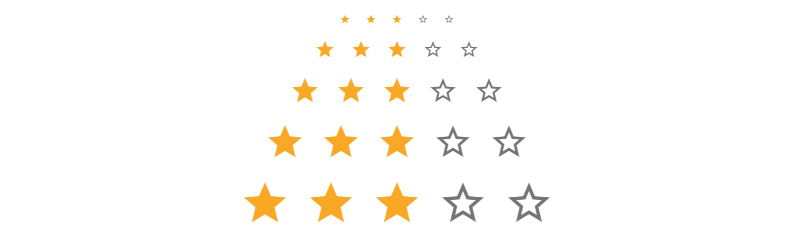
Item Slot
v-rating
comes with an item
slot that we can use to apply a greater amount of customization.
<template>
<v-app>
<div class="text-center ma-2">
<v-rating v-model="rating">
<template v-slot:item="props">
<v-icon
:color="
props.isFilled
? getColor(props.index)
: 'grey lighten-1'
"
large
@click="props.click"
>
{{
props.isFilled
? 'mdi-star-circle'
: 'mdi-star-circle-outline'
}}
</v-icon>
</template>
</v-rating>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
colors: [
'primary',
'yellow darken-3',
'red',
'purple accent-4',
'indigo',
],
rating: 4,
}),
methods: {
getColor(index) {
return this.colors[index];
},
},
};
</script>

Conclusion
We can use the Vuetify rating component (v-rating
) in our application to collect user feedback. This component comes with various props and slots that allow customization.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
