To get the height of an element before render in React, we can combine two React hooks: useRef
and useLayoutEffect
.
import React, { useRef, useLayoutEffect, useState } from 'react';
function ExampleComponent() {
const [height, setHeight] = useState(0);
const elementRef = useRef(null);
useLayoutEffect(() => {
setHeight(elementRef.current.offsetHeight);
}, []);
return (
<div ref={elementRef}>
{`The height of this element is: ${height}px`}
</div>
);
}
See also: How to Get the Width of an Element Before Render in React
useLayoutEffect()
works just like useEffect()
, but the key difference is that it fires before the browser repaints the screen – before React renders the component.
We pass an empty dependencies array to useLayoutEffect()
to make sure it only gets called once.
Here we create a ref for the target element with useRef()
, and assign it to its ref
prop, so we can access the HTMLElement
object for this React element.
useRef
returns a mutable ref object that doesn’t change its value when a component is updated. Also, modifying the value of this object’s current
property does not cause a re-render. This is in contrast to the setState
update function returned from useState
.
We do use useState()
though, to create a state that we update when useLayoutEffect()
gets called. So calling setHeight()
will cause the component to be re-rendered.
To get the actual height, we use the offsetHeight
property which includes borders, padding, and any scrollbars when calculating the element’s height.
It’s also possible to get the height of the entire window in React, instead of just one element.
Get height of element with clientHeight
clientHeight
is another way to get the height of an element. Unlike offsetHeight
, it includes padding but excludes borders and scrollbars.
import React, { useRef, useLayoutEffect, useState } from 'react';
function ExampleComponent() {
const [height, setHeight] = useState(0);
const elementRef = useRef(null);
useLayoutEffect(() => {
setHeight(elementRef.current.clientHeight);
}, []);
return (
<div ref={elementRef}>
{`The height of this element is: ${height}px`}
<p>This element's height is measured using clientHeight.</p>
</div>
);
}
Get height of element before render and on resize in React
If you want to get the height of an element before render and also on resize, here’s what you’ll do:
- Combine the
useLayoutEffect()
anduseRef()
hooks to get the element’s height before it renders, like in the previous section. - Track the current height with state.
- Add a
resize
event listener to thewindow
object inuseLayoutEffect()
. - In the event listener, get the new height of the element, and update the state with it.
So it’s similar to what we did previously, but we’re adding a resize
event listener this time.
import React, { useLayoutEffect, useState, useRef } from 'react';
function ExampleComponent() {
const [height, setHeight] = useState(0);
const elementRef = useRef(null);
useLayoutEffect(() => {
const handleResize = () => {
setHeight(elementRef.current.offsetHeight);
};
handleResize();
window.addEventListener('resize', handleResize);
return () => {
window.removeEventListener('resize', handleResize);
}
}, []);
return (
<div ref={elementRef}>
{`The height of this element is: ${height}px`}
</div>
);
}
We call addEventListener()
in useLayoutEffect()
to set the resize
event listener on the element before it renders on the screen.
The resize
event listener is called whenever the user resizes the window. In the listener we update the state that stores the element’s height, and this causes a re-render.
We call removeEventListener()
in useLayoutEffect
‘s clean-up function, to stop listening for resize
events when the component is unmounted or when the dependencies change, to prevent memory leaks and unintended side effects.
Get height of element after render in React
We can also get the height of a React element after it renders in React, by combining the useRef()
and useEffect()
hooks.
import React, { useEffect, useState, useRef } from 'react';
function ExampleComponent() {
const [height, setHeight] = useState(0);
const elementRef = useRef(null);
useEffect(() => {
setHeight(elementRef.current.offsetHeight);
}, []);
return (
<div ref={elementRef}>
{`The height of this element is: ${height}px`}
</div>
);
}
So the difference between “before render” and “after render” is useLayoutEffect()
and useEffect()
.
useEffect()
runs after the component mounts or re-renders, unlike useLayoutEffect()
that runs before. They both have a dependencies array that cause them to fire when any of those dependencies change.
As earlier, we create a ref for the target element with useRef()
, and assign it to its ref
prop, so we can access the HTMLElement
object for this React element.
useRef
returns a mutable ref object that doesn’t change its value when a component is updated. Also, modifying the value of this object’s current
property does not cause a re-render. This is in contrast to the setState
update function returned from useState
.
We do use useState()
to create a state that we update when useEffect()
gets called. This update causes the component to update again from useEffect()
.
Get height of element after render and on resize in React
If you want to get the height of an element after render and also on resize, here’s what you’ll do:
- Combine the
useEffect()
anduseRef()
hooks to get the element’s height before it renders, like in the previous section. - Track the current height with state.
- Add a
resize
event listener to thewindow
object inuseEffect()
. - In the event listener, get the new height of the element, and update the state with it.
import React, { useLayoutEffect, useState, useRef } from 'react';
function ExampleComponent() {
const [height, setHeight] = useState(0);
const elementRef = useRef(null);
useLayoutEffect(() => {
const handleResize = () => {
setHeight(elementRef.current.offsetHeight);
};
handleResize();
window.addEventListener('resize', handleResize);
return () => {
window.removeEventListener('resize', handleResize);
}
}, []);
return (
<div ref={elementRef}>
{`The height of this element is: ${height}px`}
</div>
);
}
We call addEventListener()
in useEffect()
to set the resize
event listener on the element before it renders on the screen.
The resize
event listener is called whenever the user resizes the window. In the listener, we update the state that stores the element’s height, and this causes a re-render.
We call removeEventListener()
in useEffect
‘s clean-up function, to stop listening for resize
events when the component is unmounted or when the dependencies change, to prevent memory leaks and unintended side effects.
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
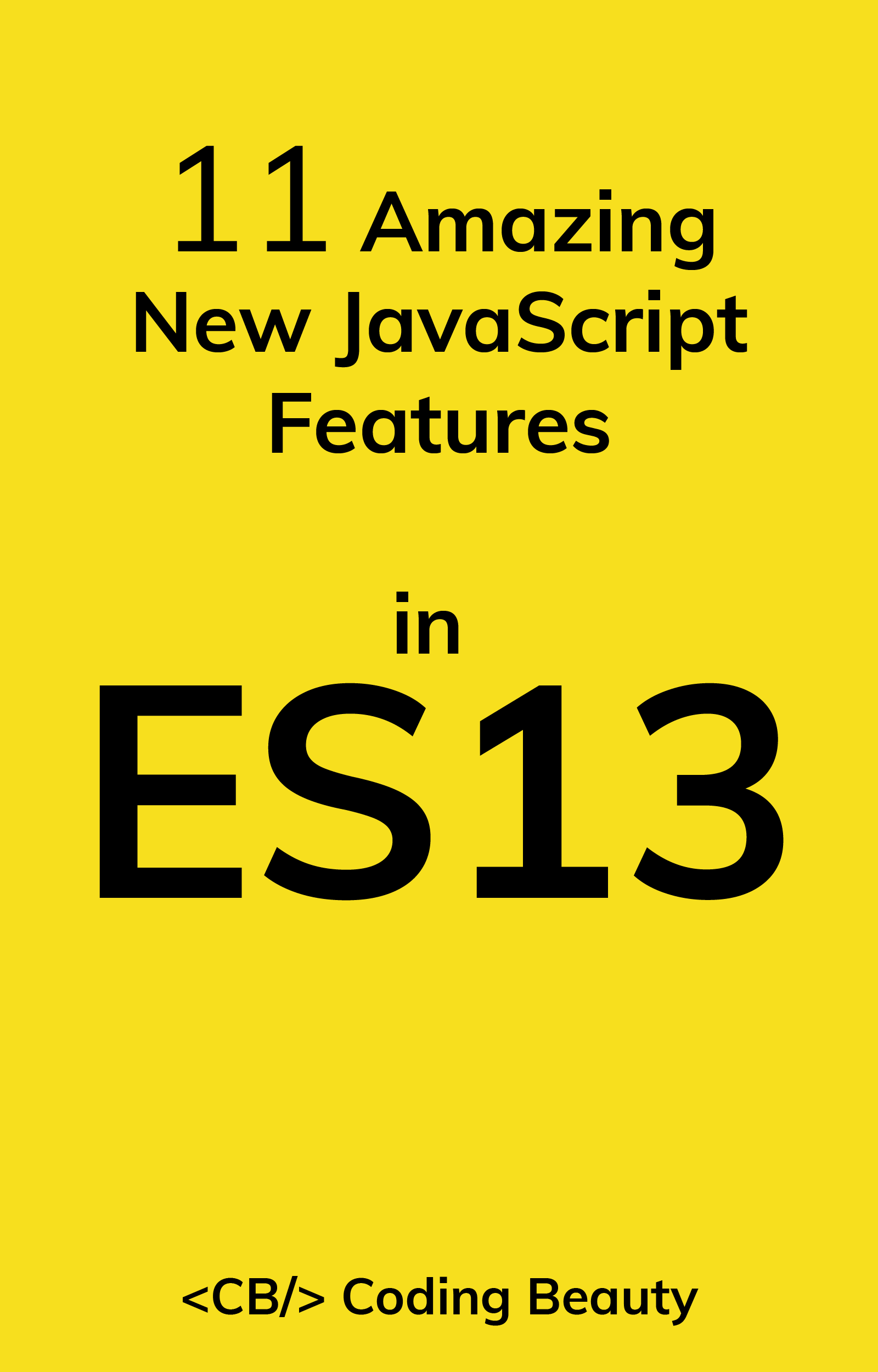