To get the width and height of the window in React, use window.innerWidth
and window.innerHeight
.
import { useRef } from 'react';
export default function App() {
const windowSize = useRef([window.innerWidth, window.innerHeight]);
return (
<div>
<h2>Width: {windowSize.current[0]}</h2>
<h2>Height: {windowSize.current[1]}</h2>
</div>
);
}
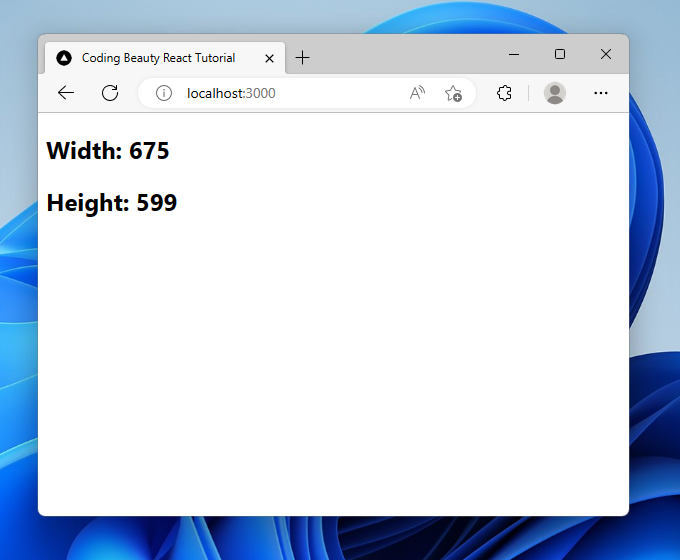
The innerWidth
property returns the interior width of the window in pixels, including the width of the vertical scrollbar, if it’s present.
Similarly, innerHeight
property returns the interior height of the window in pixels, including the height of the horizontal scrollbar, if it is present.
Since we are getting the window width and height only once – when the component mounts – we use the useRef()
hook to store them.
useRef
returns a mutable ref object that doesn’t change its value when a component is updated. Also, modifying the value of this object’s current
property does not cause a re-render. This is in contrast to the setState
update function returned from useState
.
Get window width and height on resize in React
To get the window width and height on resize in React:
- Get the window width and height with
window.innerWidth
andwindow.innerHeight
. - Add an event listener for the
resize
event on thewindow
object. - Update the window width and height in this event listener.
In the previous example, we needed to get the window width and height only once, and we used a ref to store it.
If you instead want to get the window’s width and height when it is resized, you’ll need to add a resize
event listener to the window
object and create a state variable to track changes to the width and height.
import { useState, useEffect } from 'react';
export default function App() {
const [windowSize, setWindowSize] = useState([
window.innerWidth,
window.innerHeight,
]);
useEffect(() => {
const handleWindowResize = () => {
setWindowSize([window.innerWidth, window.innerHeight]);
};
window.addEventListener('resize', handleWindowResize);
return () => {
window.removeEventListener('resize', handleWindowResize);
};
}, []);
return (
<div>
<h2>Width: {windowSize[0]}</h2>
<h2>Height: {windowSize[1]}</h2>
</div>
);
}
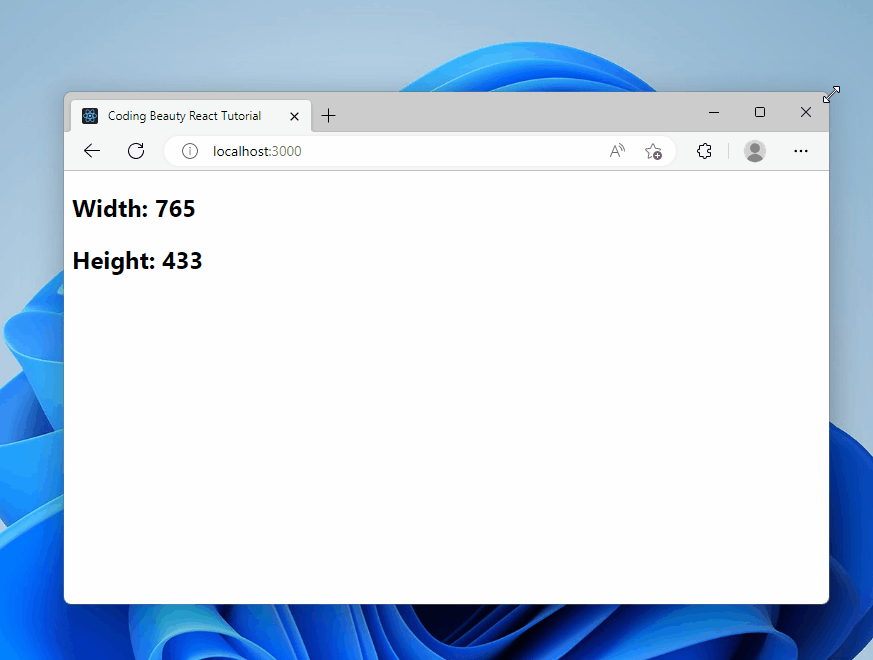
We use the useState
React hook to create a state variable that will be updated whenever the height or width of the window changes.
The useState
hook returns an array of two values. This first is a variable that stores the state, and the second is a function that updates the state when it is called.
const [windowSize, setWindowSize] = useState([
window.innerWidth,
window.innerHeight,
]);
The useEffect
hook is used to perform an action when a component first renders, and when one or more specified dependencies change. In our example, the action is to add the event listener for the resize
hook with the addEventListener()
method.
We pass an empty dependencies array to the useEffect
, so that it gets called only once in the component’s lifetime, and the resize event listener is only registered once – when the component first renders.
useEffect(() => {
const handleWindowResize = () => {
setWindowSize([window.innerWidth, window.innerHeight]);
};
window.addEventListener('resize', handleWindowResize);
return () => {
window.removeEventListener('resize', handleWindowResize);
};
}, []);
In the resize
event listener, we update the state variable with the new height and width of the window.
The function we return in useEffect
is a function that performs clean-up operations in the component. We use the removeEventListener()
method to remove the resize
event listener in this clean-up function and prevent a memory leak.
Note
useEffect
‘s cleanup function runs after every re-render where its dependencies change in addition to when the component unmounts. This prevents memory leaks that happen when an observable prop changes value without the observers in the component unsubscribing from the previous observable value.
Since we pass an empty dependencies array, its dependencies won’t ever change, so in this case, it’ll run only when the component unmounts.