This new UI framework is an absolute game changer😲
The new shadcn/ui
framework completely transforms how we design and build modern web apps.
It solves every major issue we’ve had with ALL those UI frameworks: Material UI, Bootstrap…
Project bloat and customization have always been huge pains.
Imagine, you import thousands of lines of CSS code, all just to create a button — that you end up customizing anyway.
When you install these classic libraries, you get every single one of the components dumped into your codebase. There’s no escape.
ShadCN solves this problem in a radical way.
Zero classes. Zero built-in components.
It’s pure… copy and paste?
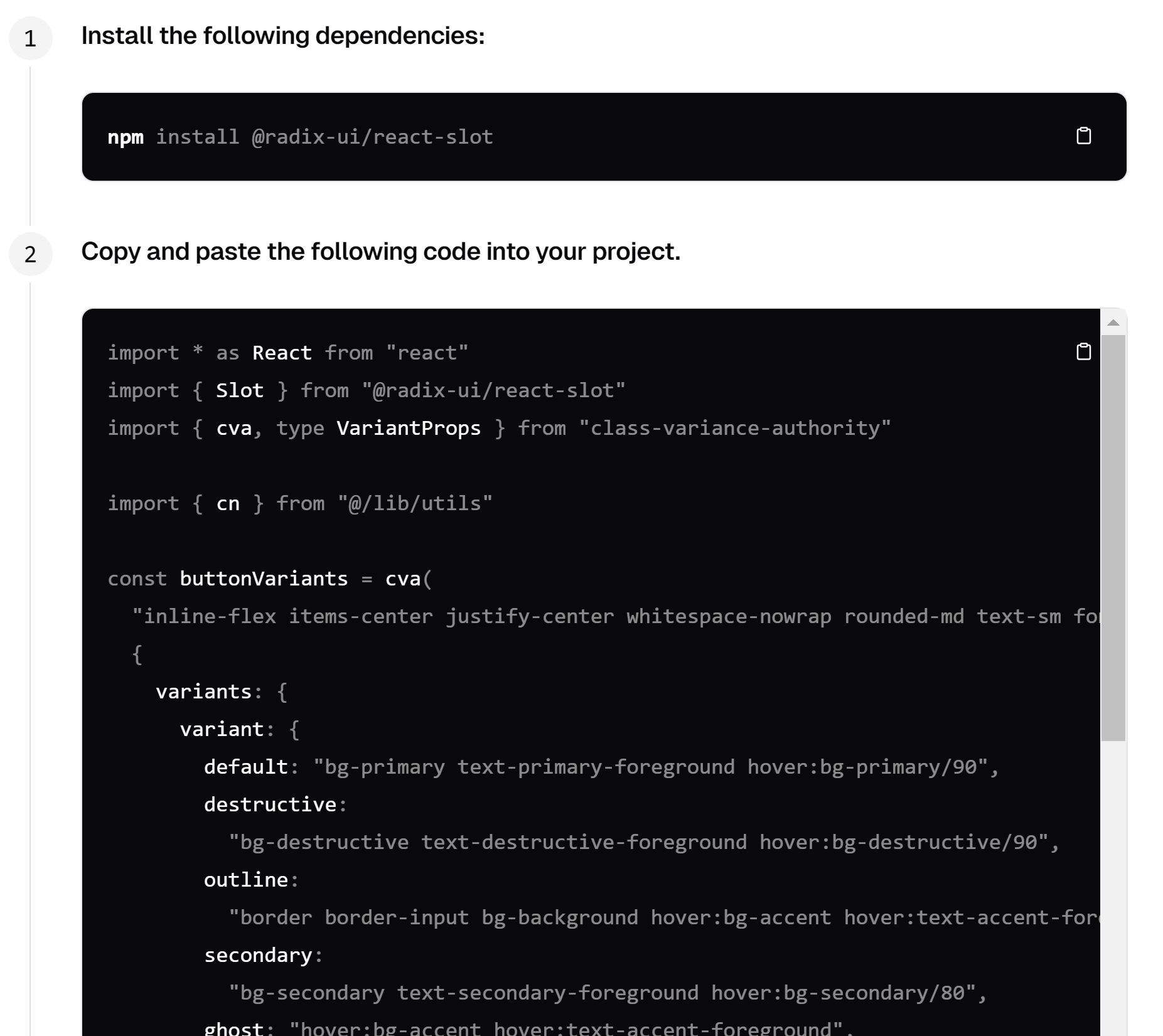
❌Before in Material UI and others:
You couldn’t see the component code directly and could only customize it with external classes.
The worst part is that the component’s appearance can change completely and break your styles when you upgrade to a new version.
✅But now with shadcn/ui:
You have total control over all your component files. You can see everything.
The button code completely belongs to me here. I can make whatever change I want and it’ll stay that way.
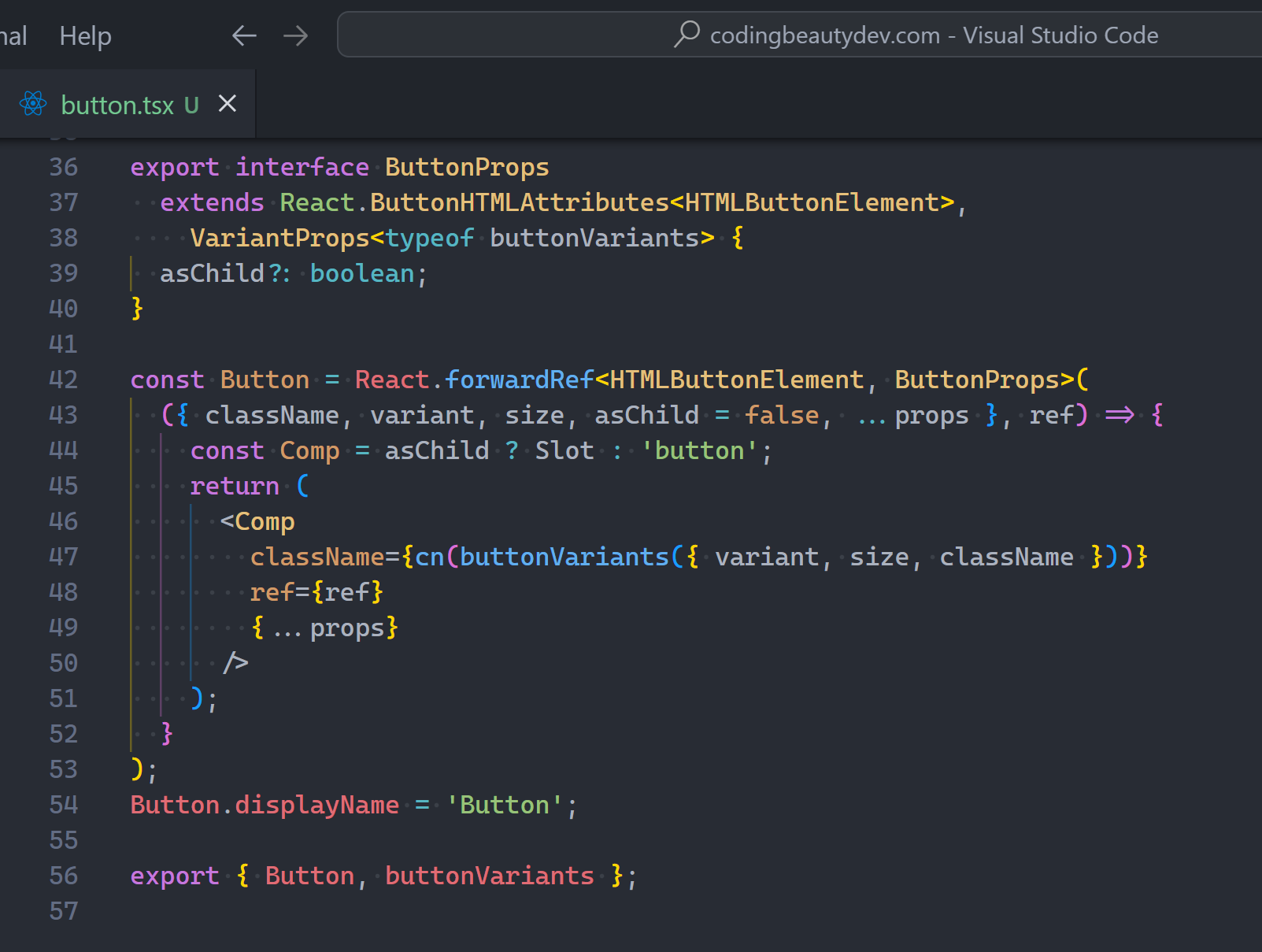
Result in page:
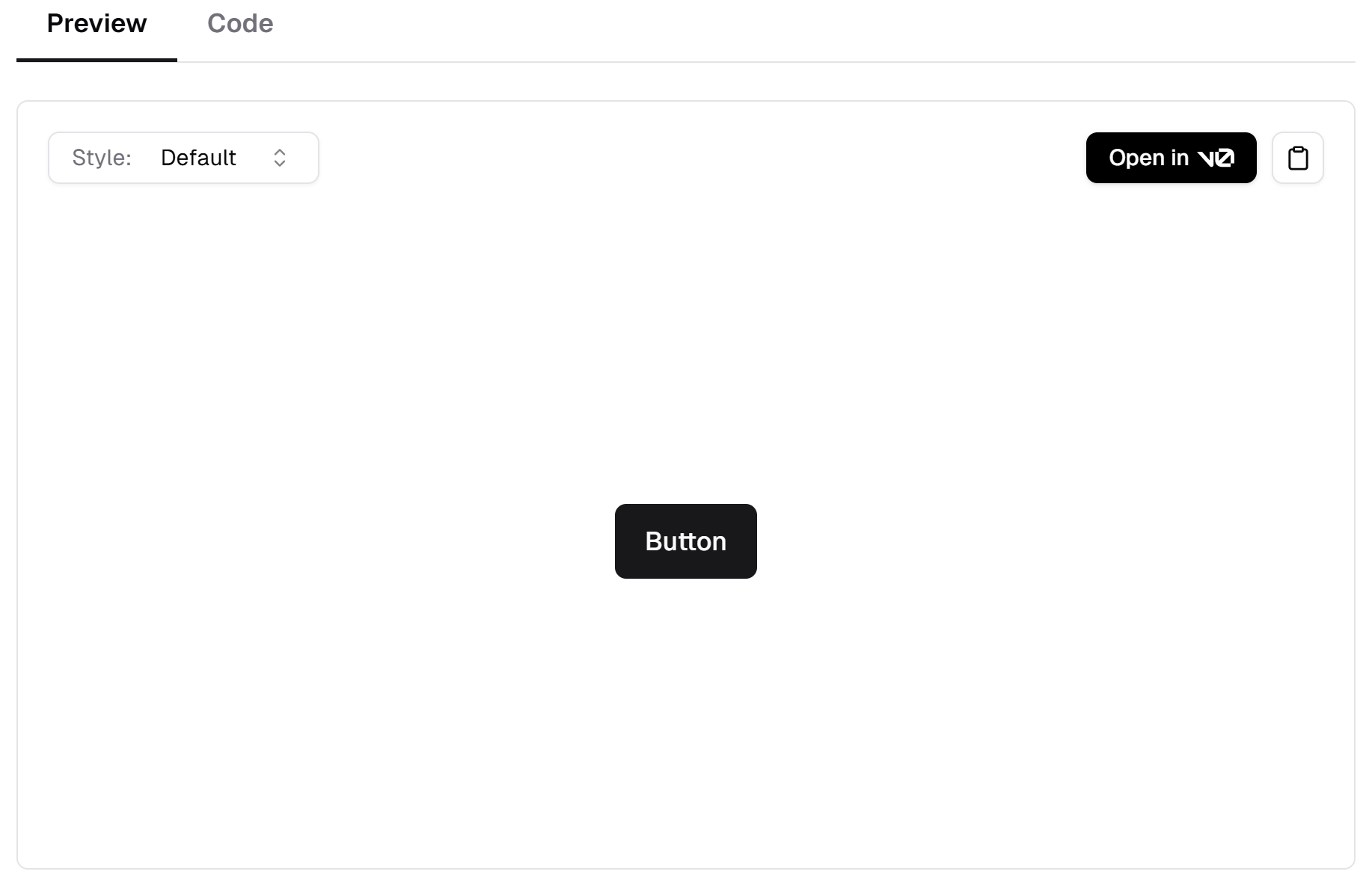
And all the initial styles are done with Tailwind classes for rapid, effortless customization.
It’s an open book where you see exactly how everything is styled and all the possible variants.
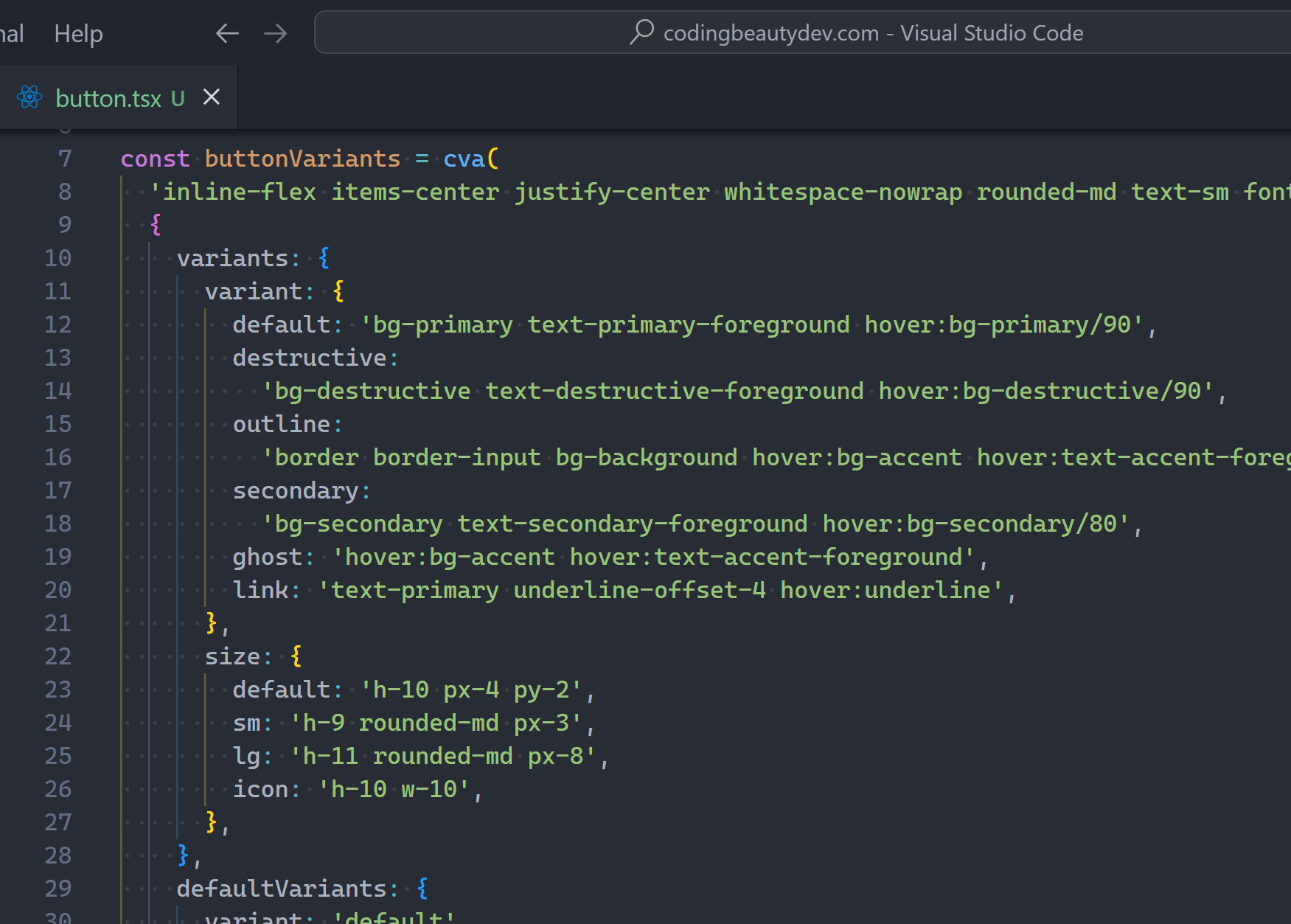
And of course this button is only one of several dozen components in the library.
We’ve got all the classics: Alerts, Cards, Dialogs, and so much more.
And now with the new shadcn/ui
CLI, adding new components to our project is easier than ever.
Instead of going to the site to copy the component I can add it directly from the CLI:
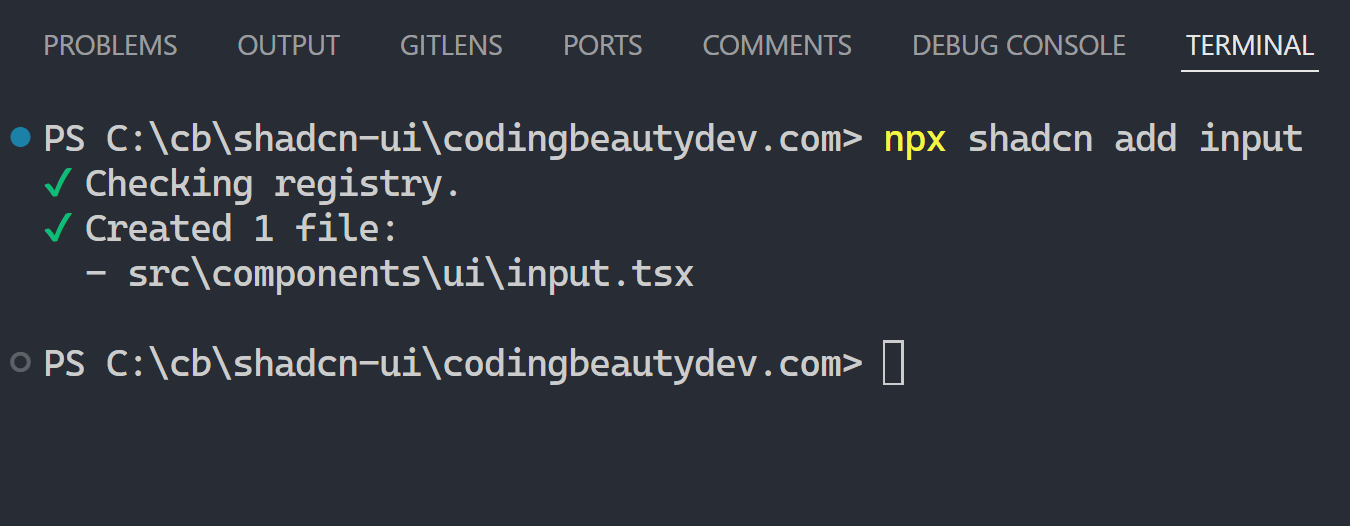
Note: It doesn’t “install” anything in node_modules. It simply automates the copying and pasting for us:
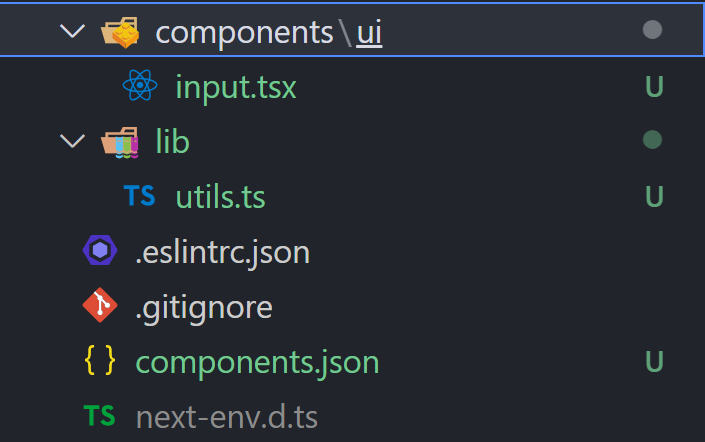
Everything we need:
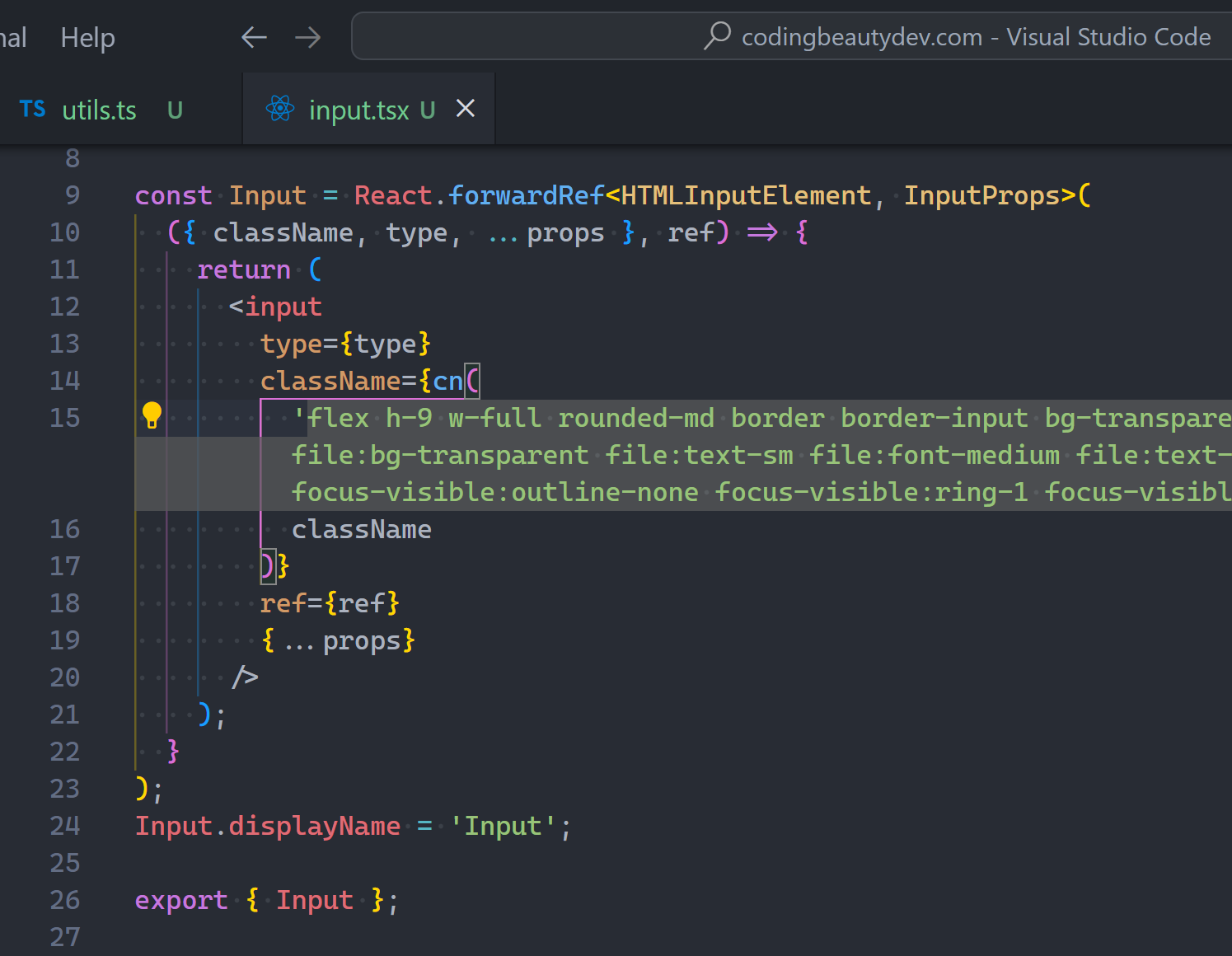
shadcn/ui
‘s reusable nature makes it perfect not just for providing components, but also for even more complex UI element groups we call blocks.
Chart blocks:
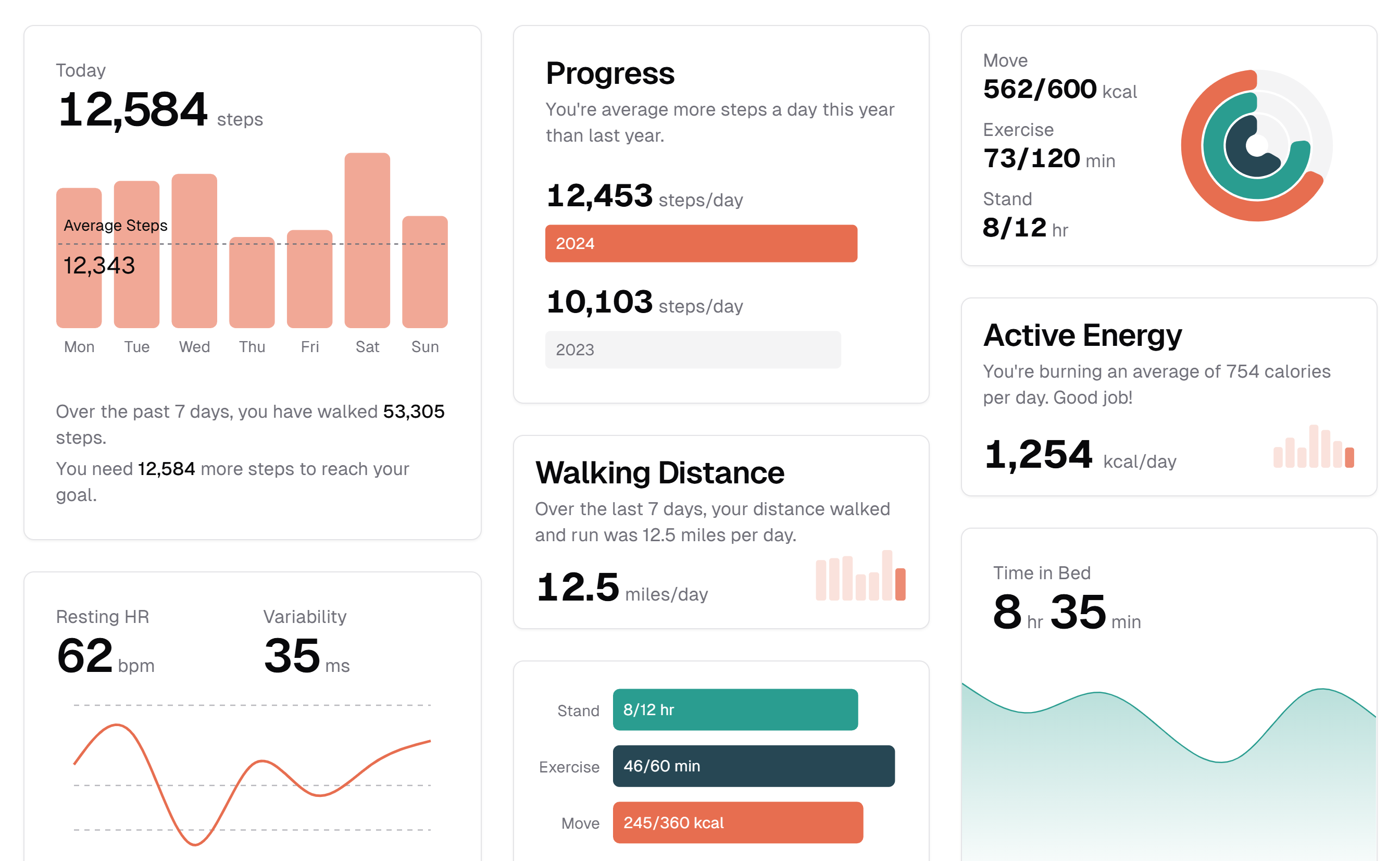
Dashboard element blocks:
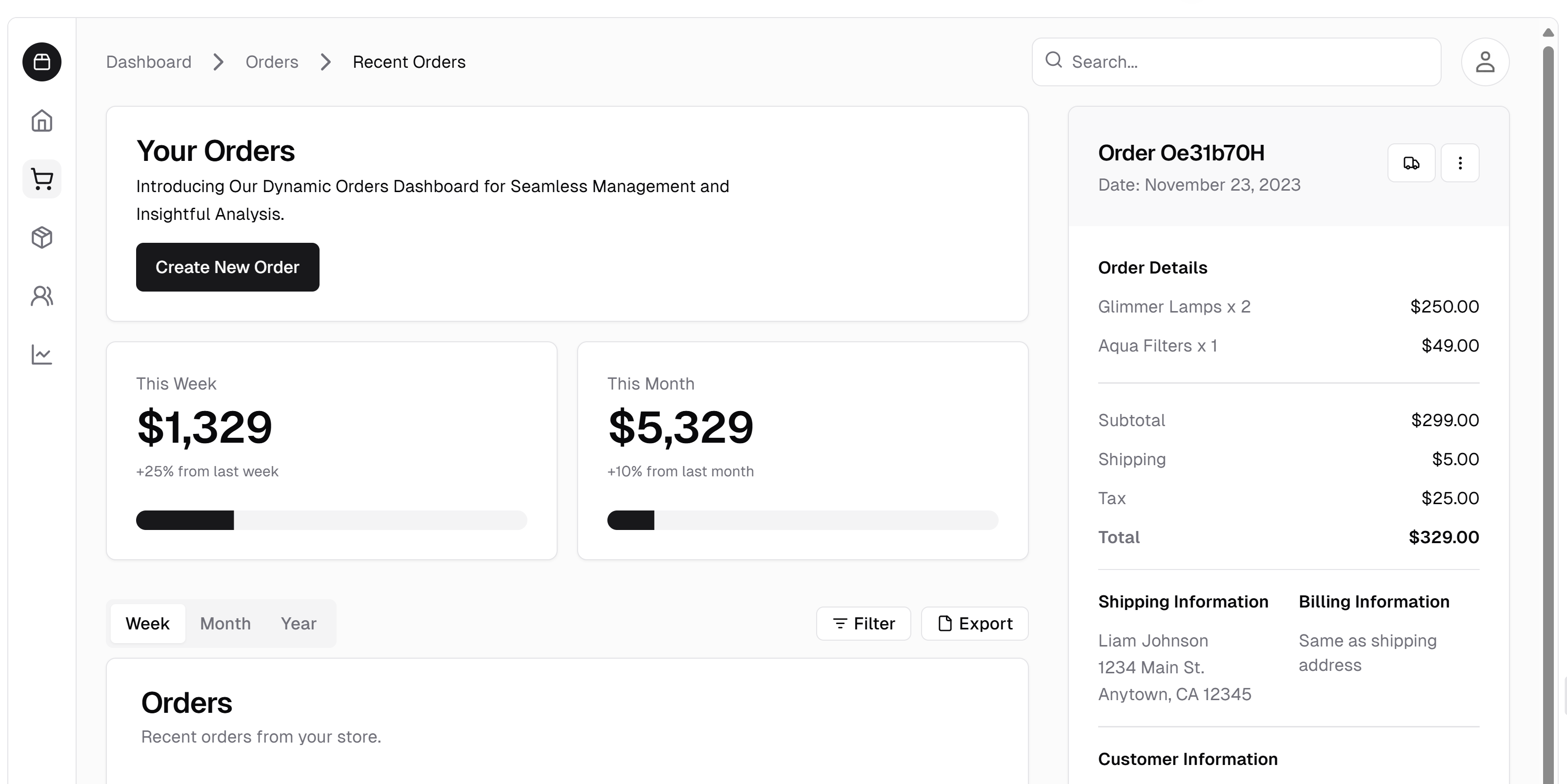
So many amazing blocks that look great by default and are endlessly customizable.
Since it’s just JSX and CSS, you can copy just the few chunks you want and drop them into your project.
Every great UI framework has extensive theme support and ShadCN is no exception.
You can pick from a broad range of color and border-radius to create the theme.
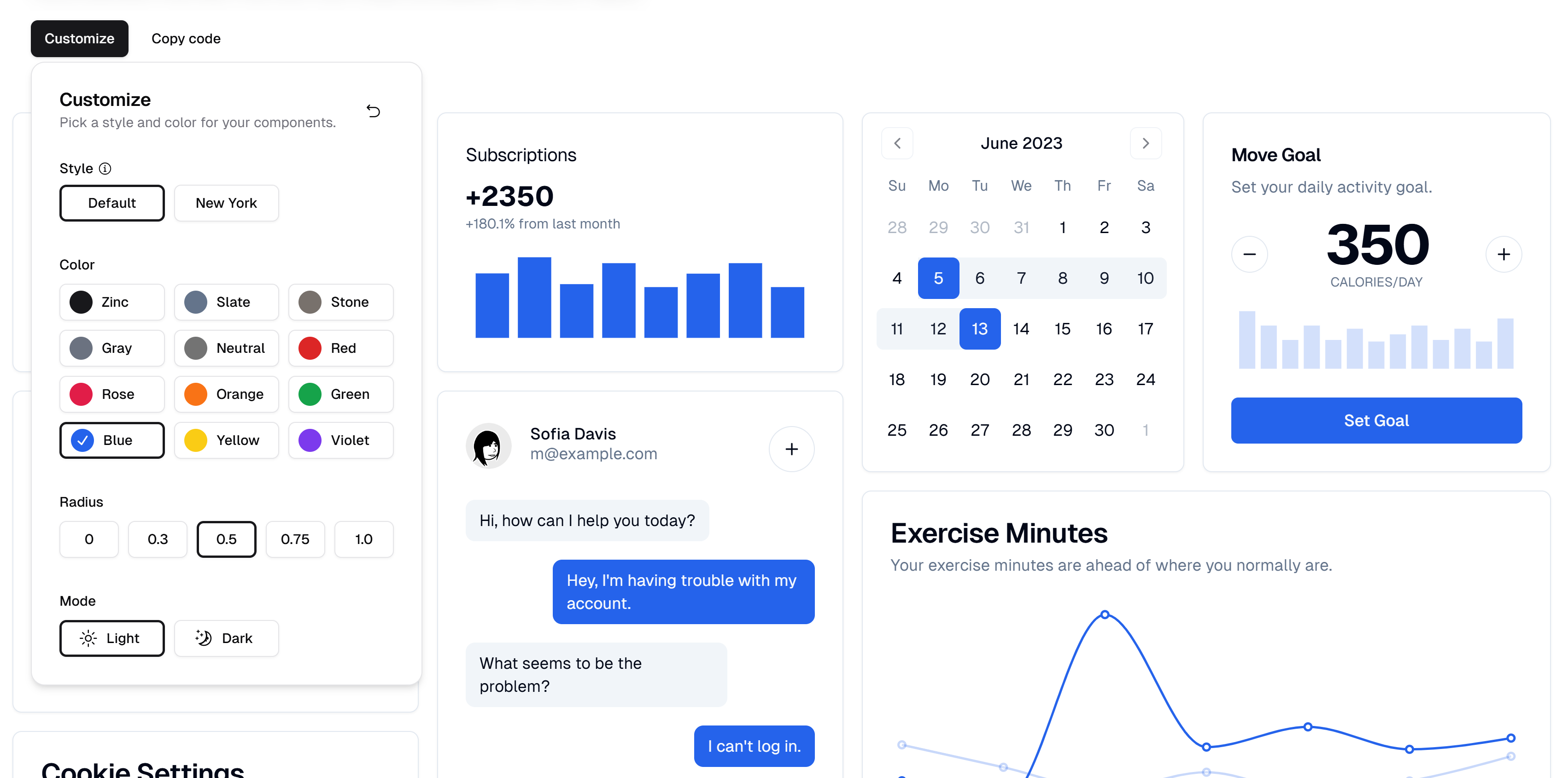
Stunning dark mode:
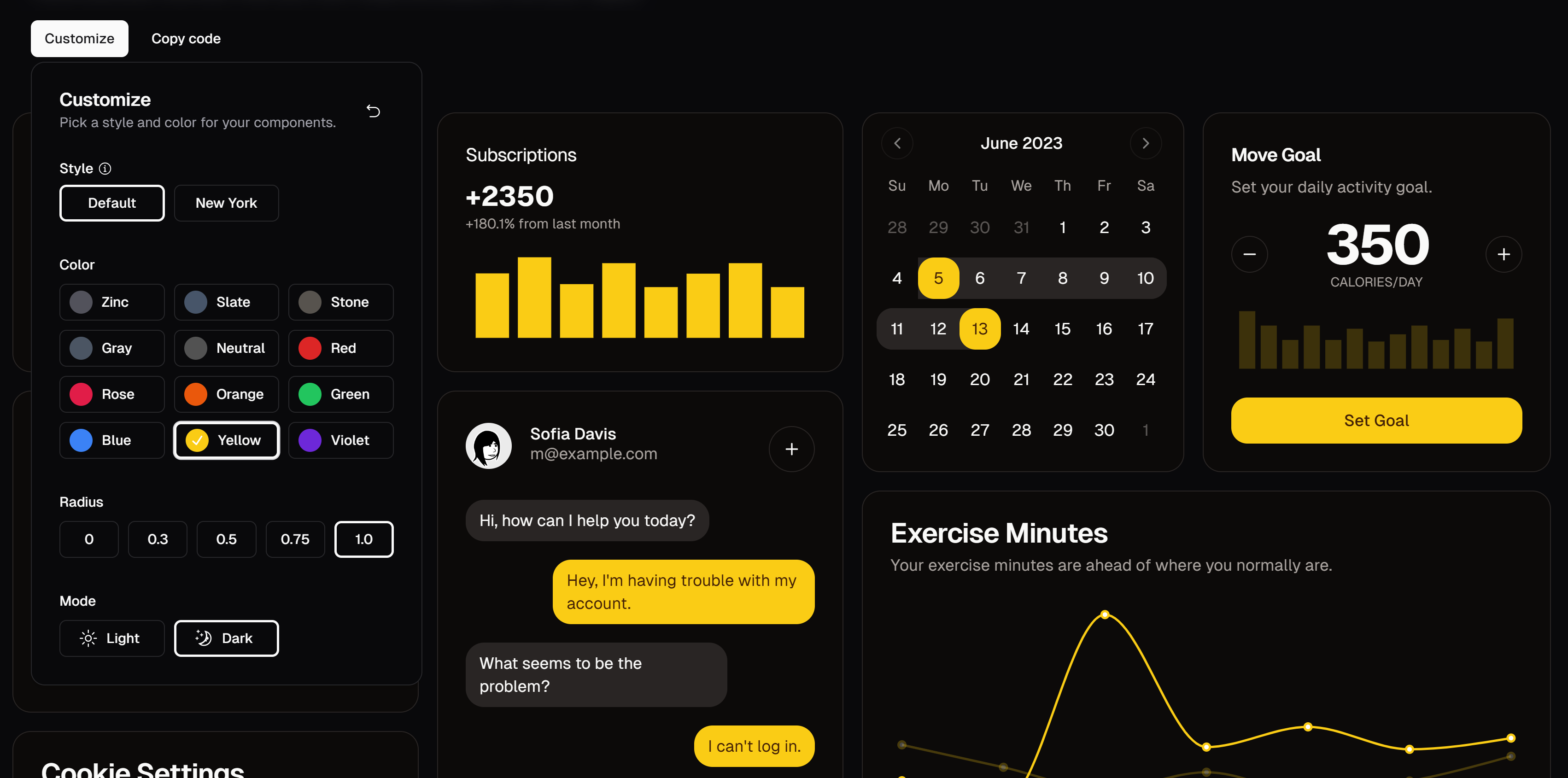
And when you’re done, you simply copy and paste the CSS as variables in your Tailwind layer:
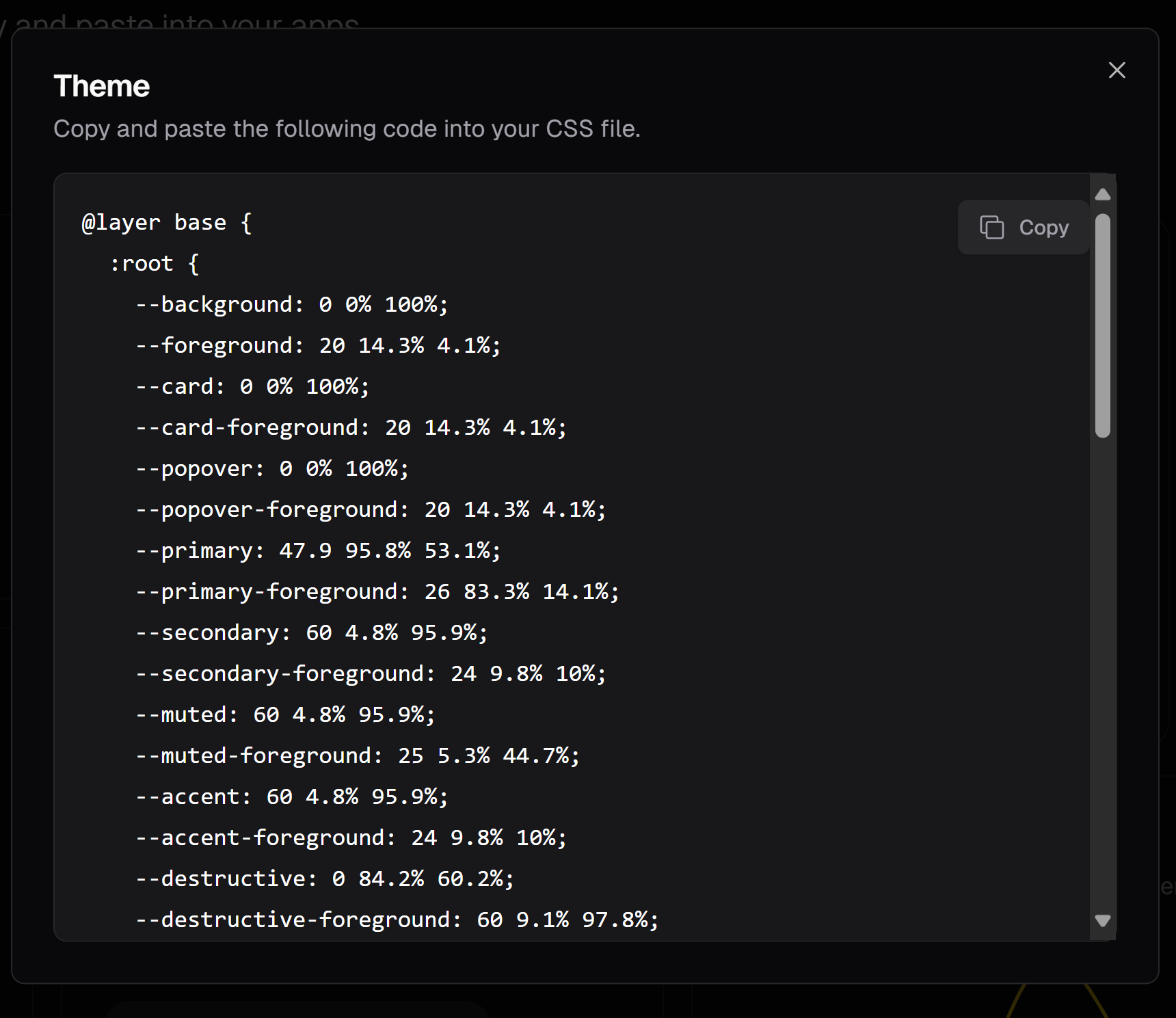
You can even set a theme when you first add the shadcn/ui
config files to your project.
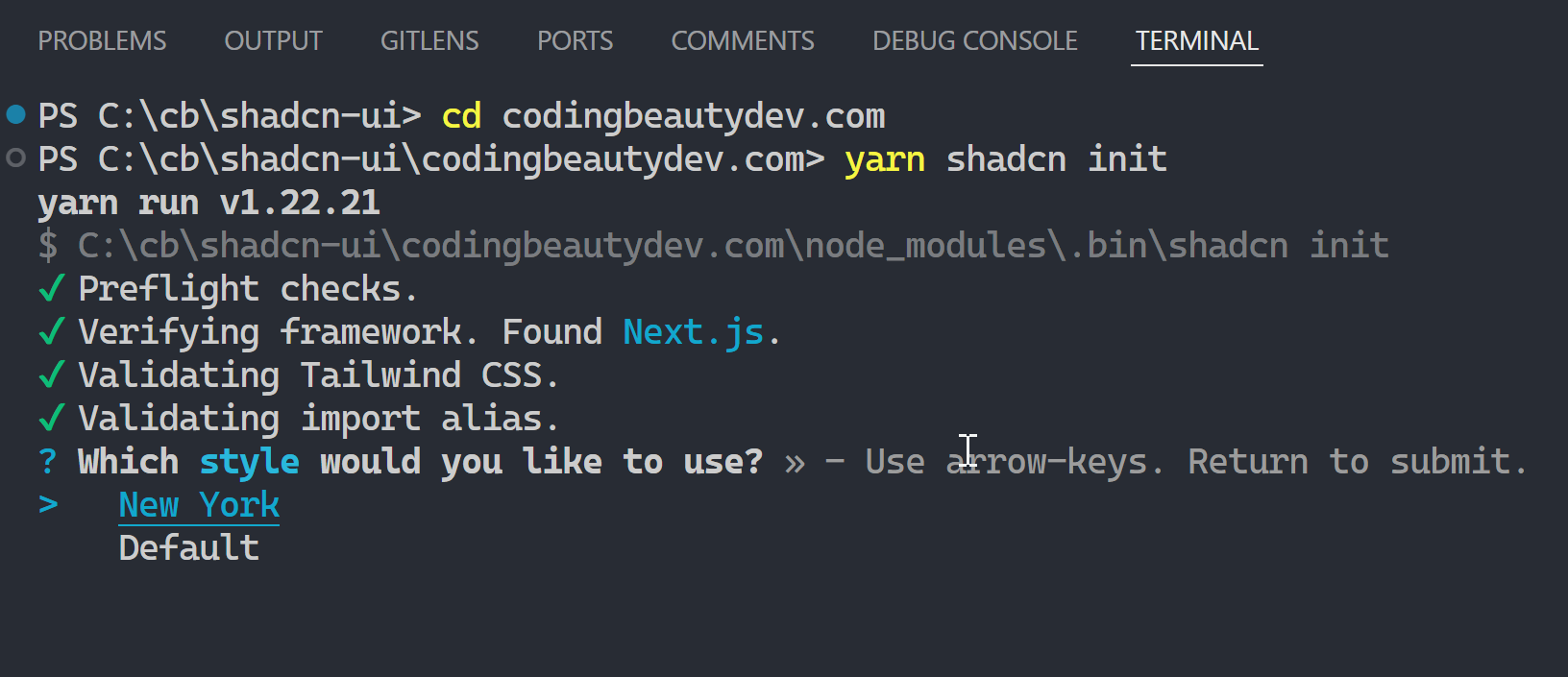
Final thoughts
shadcn/ui
changes everything for web development, helping us design and build breathtaking web apps easier and faster than ever.