AI coding agents are not glorified StackOverflow
It’s a common pushback I hear from the usual AI deniers.
Oh Tari why are you hyping this thing up so much lol, it’s no different from copying and pasting from StackOverflow for goodness sake. Just calm down bro.
They refuse to accept reality.
Like how could you possibly equate those two?
It’s like saying hiring a chef to cook a meal is no different from searching for recipe and doing the cooking yourself.
Just because the same knowledge is used in both cases, or what?
It makes no sense.
AI agents are not “improved Google” or “improved StackOverflow”.
Can StackOverflow build entire features from scratch spanning multiple files in your project?
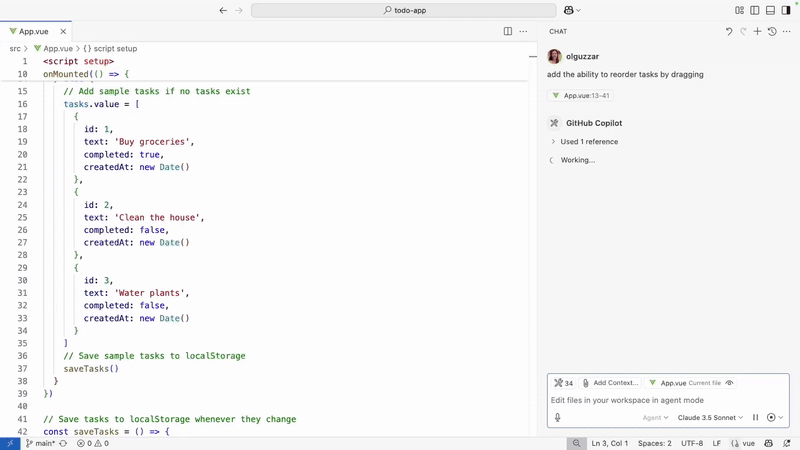
Can Google fix errors in your code with something as simple as “what’s wrong with this code”?
Do they have any deep contextual access to your code to know what you could possibly mean with an instruction as vague as that?
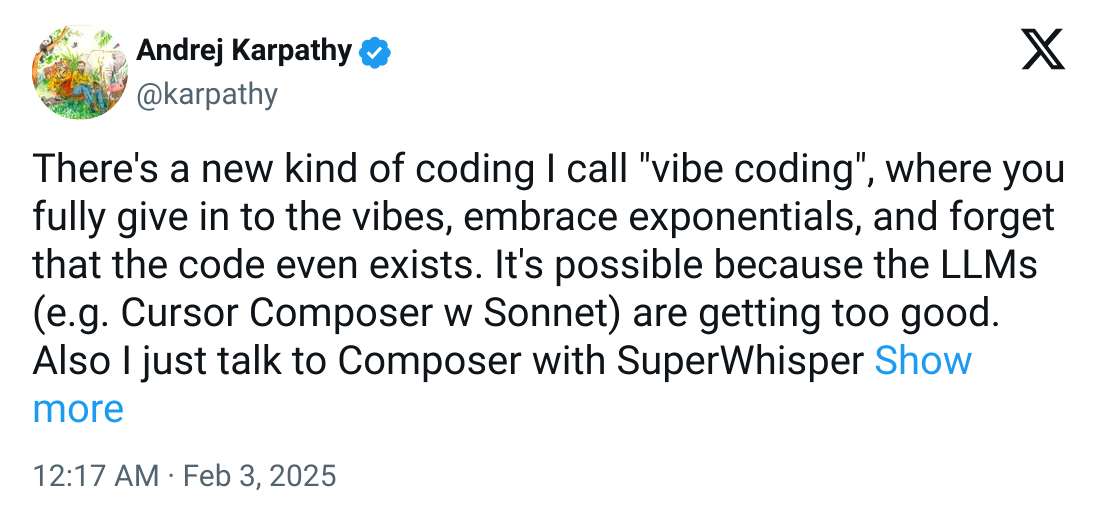
All StackOverflow and Google can do is give you fragments of generic information — you have to do the reasoning yourself.
It‘s up to you to specialize and integrate the information into your project.
And then you still have to do testing and debugging as always.
AI agents are here to do all of these much faster with far greater accuracy.
That’s like the whole point of AI — of automation.
Massive amounts of work done in tiny fractions of effort of the manual alternative.
Faster. Easier.
Predictability. Insane personalization. Smart recommendations.
These are the things that make AI so deadly. It doesn’t matter if they use the same knowledge sources that a human would use.
It’s what would make a hypothetical AI cooking machine a danger to chef careers.
It doesn’t matter if the coding or cooking information and answers are already out there on the Internet.
Even when it comes to accessing knowledge, chatbots are still obviously better at giving it to you in a straightforward cohesive manner, after researching and synthesizing the information from different sources.
Copy and pasting from StackOverflow and Google cannot give you any of these benefits.