A radio button is useful when you need to obtain user input from a group of options. For example, a user can select a preferred language or country with it. In this article, we’re going to learn how to create and customize a radio button using Vuetify.
The Vuetify Radio Button Component
Use the v-radio
component to create a basic radio button:
<template>
<v-app>
<div class="d-flex justify-center mt-4">
<v-radio></v-radio>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>

Radio Button Labels
To describe a radio button to the user, we can use the label
prop:
<template>
<v-app>
<div class="d-flex justify-center mt-4">
<v-radio label="Radio"></v-radio>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>

Radio buttons are typically used with radio groups in Vuetify. We can do this by wrapping all the v-radio
s in a v-radio-group
. We can then set up a two-way binding with the radio group component through v-model
, which will allow us to access and set the currently selected radio button in the group:
<template>
<v-app>
<div class="d-flex justify-center mt-4">
<v-radio-group v-model="radioGroup">
<v-radio
v-for="n in 3"
:key="n"
:label="`Radio ${n}`"
:value="n"
></v-radio>
</v-radio-group>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
radioGroup: 1,
}),
};
</script>

Clicking on another radio button in the group will update the variable and change the selection:

Radio Button Custom Colors
The radio button component has a color
prop that we can use to customize its color:
<template>
<v-app>
<div class="d-flex justify-center mt-4">
<v-radio-group v-model="radioGroup">
<v-radio
v-for="(color, i) in colors"
:key="i"
:label="color"
:value="color"
:color="color"
></v-radio>
</v-radio-group>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
colors: ['indigo', 'yellow', 'error', 'green', 'primary'],
}),
};
</script>
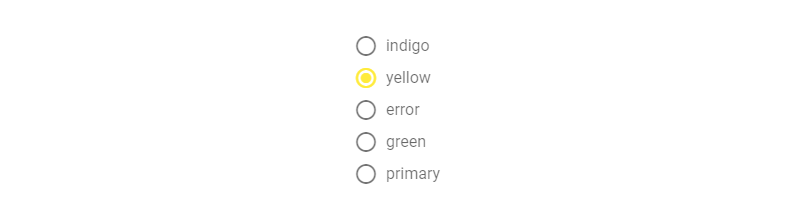
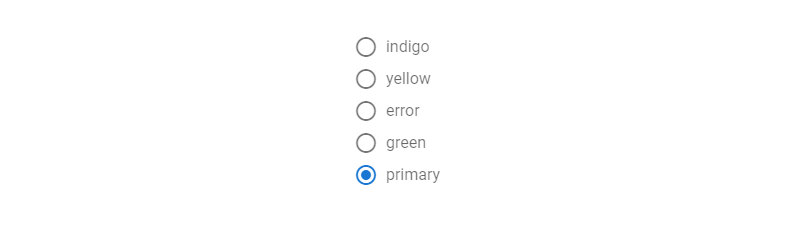
Radio Button Directions
We can use the row
prop to present the radio buttons horizontally:
<template>
<v-app>
<div class="d-flex justify-center mt-4">
<v-radio-group v-model="radioGroup" row>
<v-radio
v-for="i in 3"
:key="i"
:label="`Option ${i}`"
:value="i"
></v-radio>
</v-radio-group>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>

The column
prop will present radio buttons vertically, and is the default direction:
<template>
<v-app>
<div class="d-flex justify-center mt-4">
<v-radio-group v-model="radioGroup" column>
<v-radio
v-for="i in 3"
:key="i"
:label="`Option ${i}`"
:value="i"
></v-radio>
</v-radio-group>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
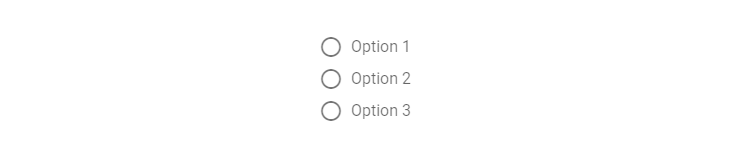
Ensuring a Mandatory Value
To make sure a radio group always has a value, set the mandatory
prop to true
:
<template>
<v-app>
<div class="d-flex justify-center mt-4">
<v-radio-group v-model="radioGroup" mandatory>
<v-radio
v-for="i in 3"
:key="i"
:label="`Option ${i}`"
:value="i"
></v-radio>
</v-radio-group>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
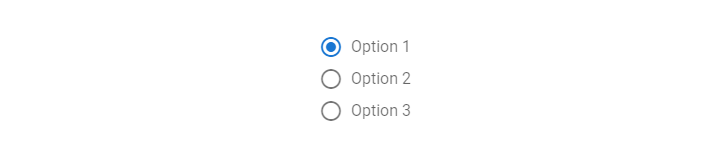
Labels with HTML
If we want to include HTML in the label of a radio group, we can put the content in the label
slot of the v-radio-group
:
<template>
<v-app>
<div class="d-flex justify-center mt-4">
<v-radio-group v-model="radioGroup" mandatory>
<template v-slot:label> Pick an <strong>option</strong> </template>
<v-radio
v-for="i in 3"
:key="i"
:label="`Option ${i}`"
:value="i"
></v-radio>
</v-radio-group>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
};
</script>
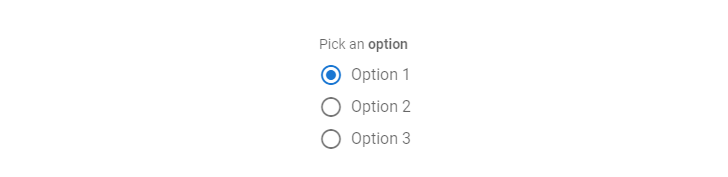
We can also include HTML in the labels of the radio buttons with their own label
slots:
<template>
<v-app>
<div class="d-flex justify-center mt-4">
<v-radio-group v-model="selected">
<template v-slot:label>
Select your default <strong>search engine</strong>
</template>
<v-radio v-for="(url, name) in searchEngines" :key="name" :value="name"
><template v-slot:label
><a :href="url">{{ name }}</a></template
></v-radio
>
</v-radio-group>
</div>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
selected: 'Google',
searchEngines: {
Bing: 'https://bing.com',
Google: 'https://google.com',
Duckduckgo: 'https://duckduckgo.com',
},
}),
};
</script>
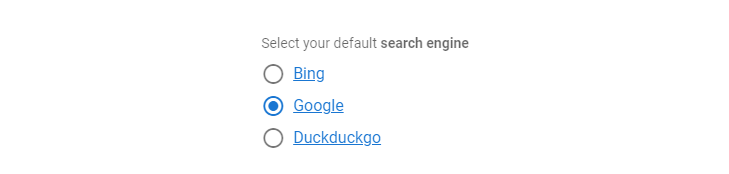
Summary
We can create a group of radio buttons when we want to receive user input from a predefined set of options. Vuetify provides the v-radio
component to create radio buttons and the v-radio-group
component to add groupable functionality to them. These components come with various props for customization.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
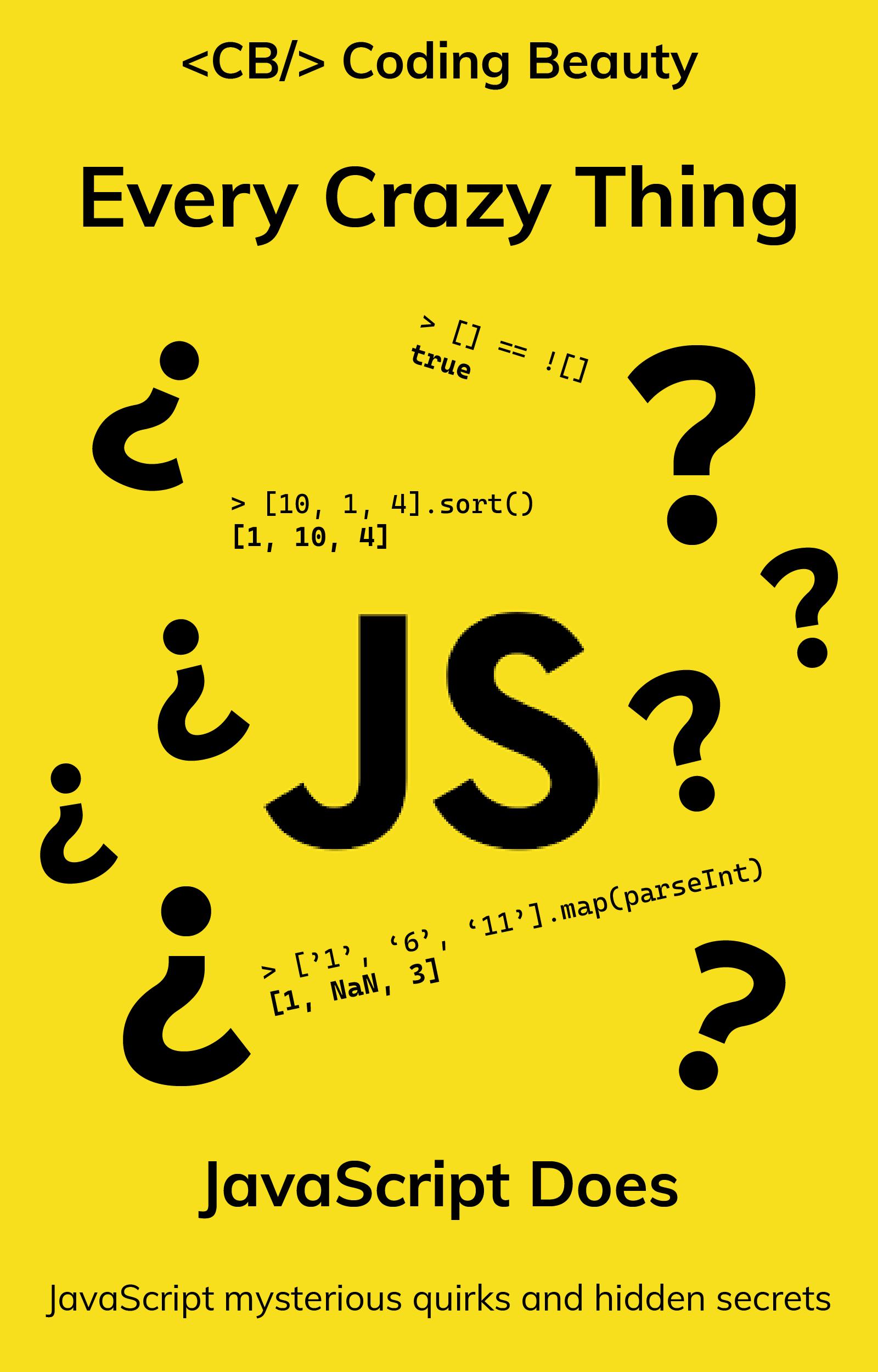