To use a button as a Vue Router link, wrap the button
element in a router-link
component. When the button is clicked, the browser will navigate to the specified route without refreshing the page.
<template>
<div>
<router-link
to="/posts"
custom
v-slot="{ navigate }"
>
<button
@click="navigate"
role="link"
>
Posts
</button>
</router-link>
</div>
</template>
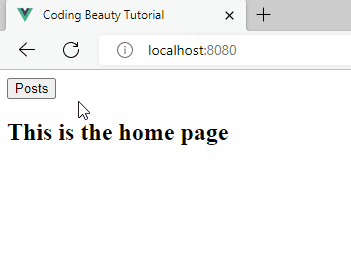
/posts
route without refreshing the page.The router-link
component has a default slot with a navigate
function property.
The to
prop is used to set the route that the browser should navigate to when this navigate
function is called.
We make navigate
an event handler of the button
click
event, so it is called when the button is clicked, making the browser navigate to the route specified with the to
prop.
Setting the custom
prop to true
tells the router-link
not to wrap its content in an <a>
element, and allow us to create a custom router link.
Use button as Vue Router link in Vue 2.x
The above method only works for Vue 3.x though. If working with Vue 2.x, you can use a button as a router link by setting the tag
prop to the name of the button component, and setting the to
prop to the specific route.
<template>
<div>
<router-link to="/posts" tag="button">Posts</router-link>
</div>
</template>
The $router.push() method
Alternatively, we can use a button as a Vue router link by calling the push()
method on the $router
variable made available by Vue Router.
<template>
<div>
<button @click="$router.push('/posts')">Posts</button>
</div>
</template>
The $router
variable represents the router instance and can be used for programmatic navigation.
Use Vuetify button as Vue Router link
When using Vue Router with Vuetify, we can use the button component as a router link by setting the v-btn to
prop.
<template>
<div>
<v-btn
to="/posts"
color="primary"
dark
>
Posts
</v-btn>
</div>
</template>
Use Vue Bootstrap button as Vue Router link
We can set the b-button
to
prop to use the Vue Bootstrap button component as a Vue Router link.
<template>
<div>
<b-button
to="/posts"
variant="success"
>
Posts
</b-button>
</div>
</template>
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
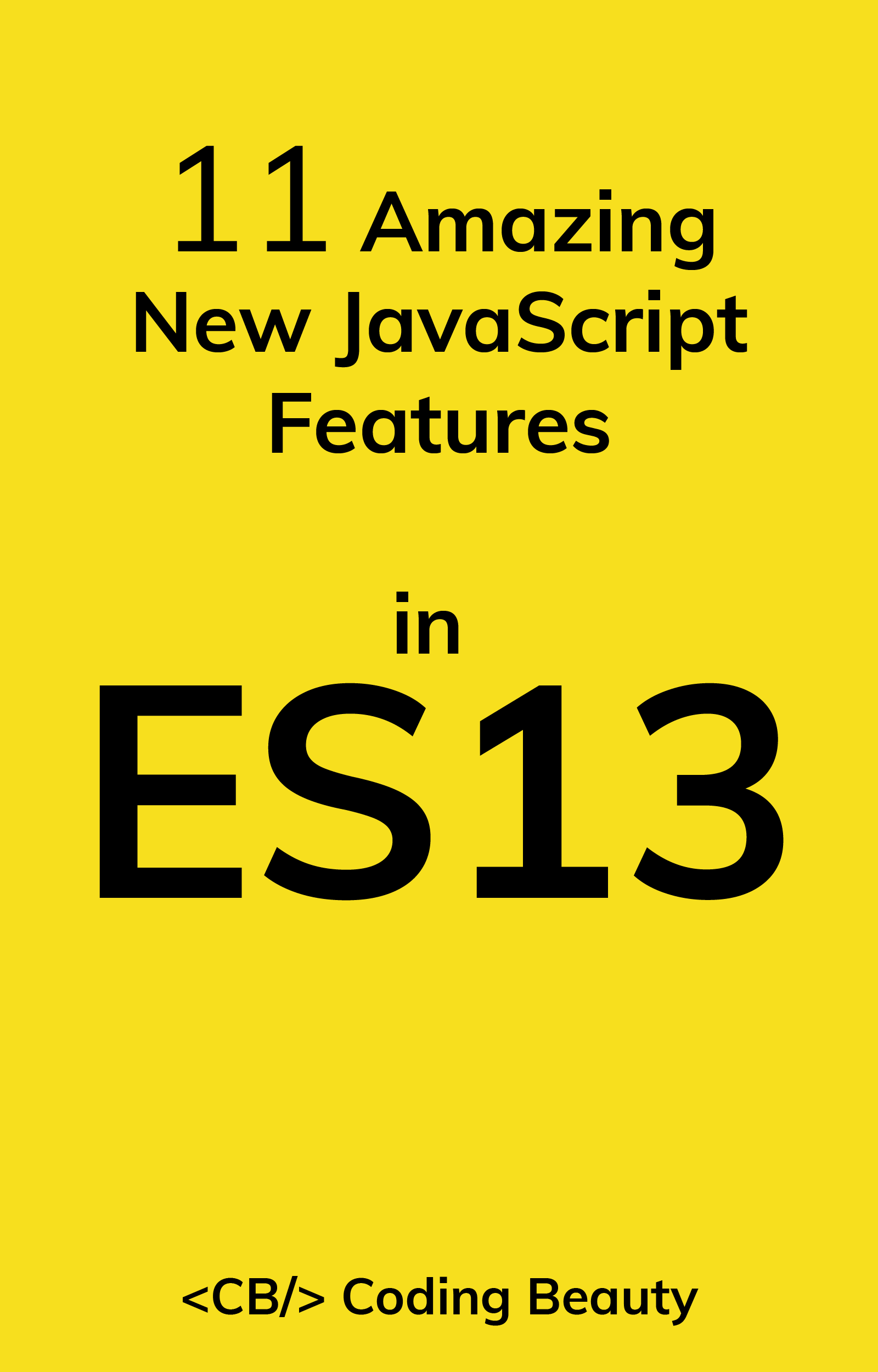