A Vue method is a function associated with every Vue instance and created with the methods
property. We can use them to perform certain actions when the user interacts with an element, like clicking on a button, or entering data into a text input. Read on to find out more about how to define and use Vue methods.
Creating a Vue Method
To create a method, we assign an object to the method property of the Vue instance. Each key of the object passed corresponds to a method name.
<script>
export default {
methods: () => ({
handleClick: () => {
alert('You clicked the button.');
},
handleChange: () => {
console.log('Text input changed');
},
}),
};
</script>
This is JavaScript, so we can use the shorter method definition syntax introduced in ES 2015:
<script>
export default {
methods: {
handleClick() {
alert('You clicked the button.');
},
handleInput() {
console.log('Text input changed');
},
},
};
</script>
Calling Methods
To handle an event with a method, we pass it to a v-on
directive attached to an element. For example:
<template>
<div>
<button v-on:click="handleClick">Click me</button>
<input v-on:input="handleInput" />
</div>
</template>
<script>
export default {
methods: {
handleClick() {
alert('You clicked the button.');
},
handleInput() {
console.log('Text input changed');
},
},
};
</script>
We can also use the @
symbol for a shorter syntax:
<template>
<div>
<button @click="handleClick">Click me</button>
<input @input="handleInput" />
</div>
</template>
<script>
export default {
methods: {
handleClick() {
alert('You clicked the button.');
},
handleInput() {
console.log('Text input changed');
},
},
};
</script>
Accessing Data from Vue Methods
We can access a data property with this.{propertyName}
, where propertyName is the name of one of the properties returned from the data()
method. For example:
<template>
<div>Num: {{ num }}</div>
<button @click="addOne">Add 1</button>
</template>
<script>
export default {
data() {
return {
num: 0,
};
},
methods: {
addOne() {
this.num++;
},
},
};
</script>
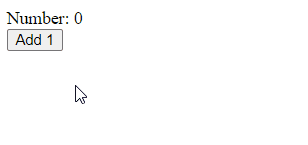
Passing Parameter Values to Vue Methods
Vue methods can accept arguments like normal JavaScript functions. We can pass arguments by calling the method directly in the template with the parameter values:
<template>
<div>Number: {{ num }}</div>
<button @click="add(5)">Add 5</button>
</template>
<script>
export default {
data() {
return {
num: 0,
};
},
methods: {
add(amount) {
this.num += amount;
},
},
};
</script>
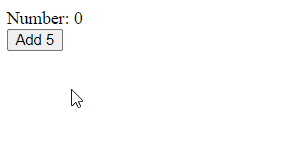
Conclusion
We can define methods on a Vue instance by passing an object to the methods
property. Vue methods are similar to JavaScript functions and allow us to add interactivity to our Vue apps.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
