After taking input from users with a text field, there has to be a way to retrieve the data and do something with it. In this article, we’ll learn how to easily get the value of an input field in Vue.
The v-model Directive
To get an input value in Vue, we can use the v-model
directive to set up a two-way binding between the value and a variable.
For example:
<template>
<div id="app">
<input
type="text"
v-model="text"
placeholder="Text"
/>
<br />
You typed: <b>{{ text }}</b>
</div>
</template>
<script>
export default {
data() {
return {
text: '',
};
},
};
</script>
Every time the user changes the text in the input field, the text
variable will be updated automatically. We can then use this variable to perform an action or display information. In this example, we simply display the value of the input below it.
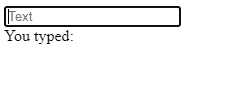
Using Vue Computed Properties with an Input Value
Instead of just displaying the value in the input field as it is, we could use a computed property to show information derived from the value.
For example, we could display the number of characters the value has:
<template>
<div id="app">
<input
type="text"
v-model="text"
placeholder="Text"
/>
<br />
Character count: <b>{{ count }}</b>
</div>
</template>
<script>
export default {
data() {
return {
text: '',
};
},
computed: {
// Computed property named "count", depending on the
// "text" variable
count() {
return this.text.length;
},
},
};
</script>
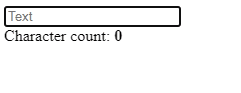
How to Get an Input Value on Change
We can create a handler for the input
event of the input
component to perform an action with the input value when it is changed. We can listen for the event using the v-on
directive (v-on:input
), which can be shortened to the @
symbol (@input
).
In the following example, we use the input
event to log the new input value in the developer console when it changes.
<template>
<div id="app">
<input
type="text"
v-model="text"
placeholder="Text"
@input="handleInput"
/>
<br />
</div>
</template>
<script>
export default {
data() {
return {
text: '',
};
},
methods: {
handleInput(event) {
console.log(event.target.value);
},
},
};
</script>
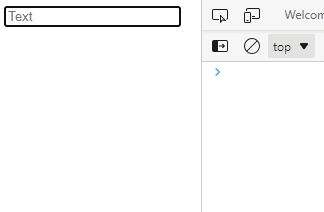
We can also the input
event to implement a custom one-way binding between the input field value and a variable. Instead of v-model
, we can use the value
prop to set the text in the input manually.
In the following example, we use the input
event and the value
prop to space out the digits of the card number entered in the input field and provide a better user experience.
<template>
<div id="app">
<input
type="text"
:value="cardNo"
placeholder="Card number"
@input="handleInput"
@keypress="handleKeyPress"
/>
<br />
</div>
</template>
<script>
export default {
data() {
return {
cardNo: '',
};
},
methods: {
handleInput(event) {
this.cardNo = event.target.value
// Remove spaces from previous value
.replace(/\s/g, '')
// Add a space after every set of 4 digits
.replace(/(.{4})/g, '$1 ')
// Remove the space after the last set of digits
.trim();
},
handleKeyPress(event) {
const num = Number(event.key);
const value = event.target.value;
// Only allow 16 digits
if ((!num && num !== 0) || value.length >= 16 + 3) {
event.preventDefault();
}
},
},
};
</script>
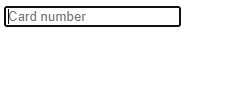
How to Get an Input Value with a Ref
In most scenarios, v-model
and @input
/value
will be sufficient for reading or updating the value of an input field. However, we can also use the ref
attribute to get the input value. We can set this attribute on any DOM element and use the $refs
property of the Vue instance to access the object that represents the element.
For example:
<template>
<div id="app">
<!-- Create new ref by setting "ref" prop -->
<input
type="text"
placeholder="Text"
ref="inputField"
/>
<button @click="handleShout">Shout</button>
<br />
<b>{{ shout }}</b>
</div>
</template>
<script>
export default {
data() {
return {
shout: '',
};
},
methods: {
handleShout() {
// Access ref with "$refs" property
const message = this.$refs.inputField.value;
this.shout = `${message.toUpperCase()}!!!`;
},
},
};
</script>
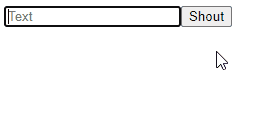
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
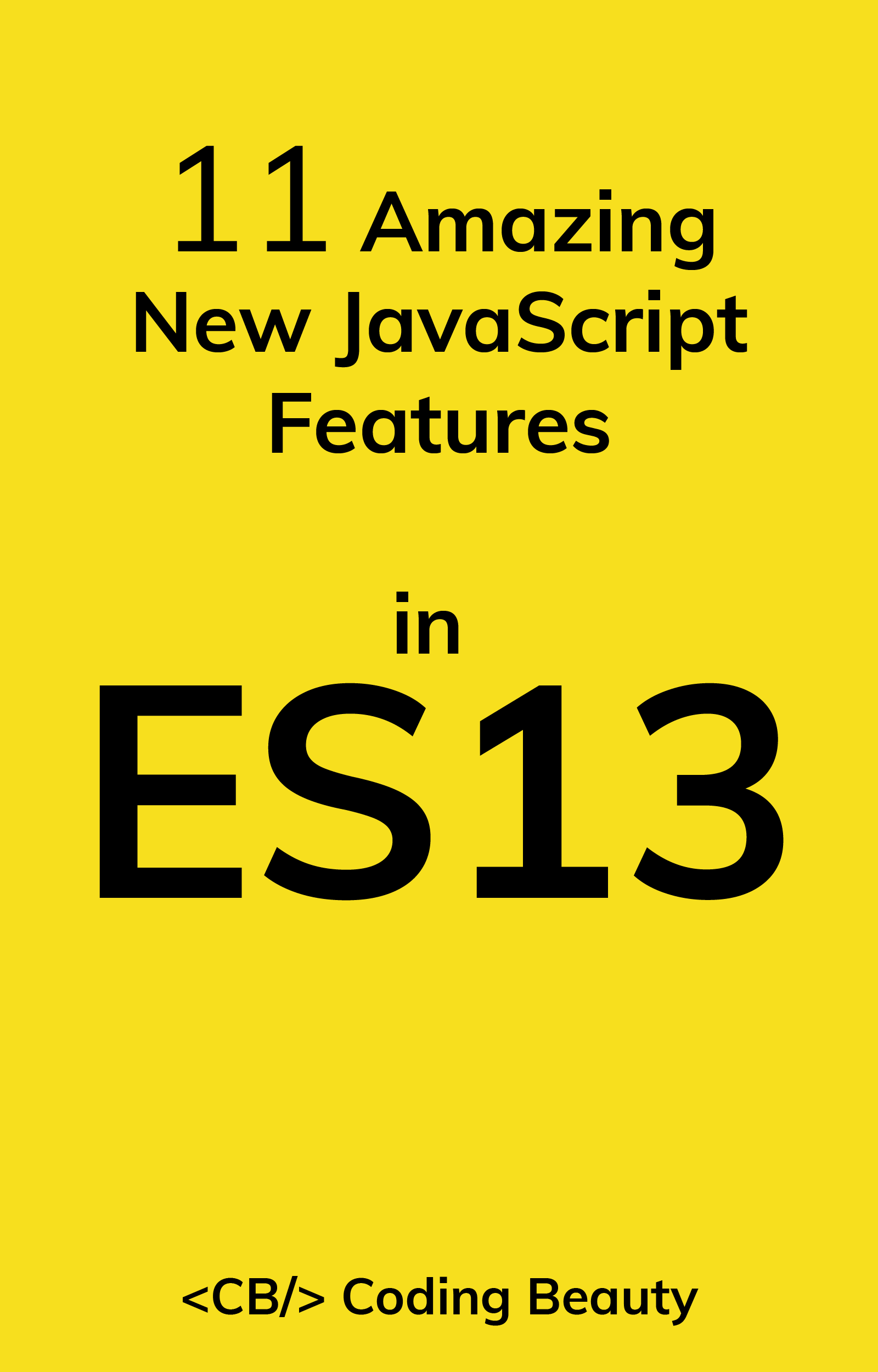