To get the current year in Vue.js, create a new Date
object with the Date()
constructor, then use the getFullYear()
method to get the year of the Date
. getFullYear()
will return a number that represents the current year.
For example:
<template>
<div>
{{ new Date().getFullYear() }}
<div>
© {{ new Date().getFullYear() }} Coding Beauty
</div>
</div>
</template>
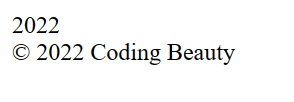
We use the Date() constructor
to create a new Date
object. When Date()
is called with no arguments, the Date
object is created using the current date and time.
The Date
getFullYear()
method returns a number that represents the year of the Date
. Since the Date
object here stores the current date, getFullYear()
returns the current year.
Get current year with data property
We can also put the current year in a data
variable instead of placing it directly in the template with the {{ }}
symbols. This allows us to more easily reuse the value in multiple places in the template
markup.
<template>
<div>
{{ currYear }}
<div>© {{ currYear }} Coding Beauty</div>
</div>
</template>
<script>
export default {
data() {
return {
currYear: new Date().getFullYear(),
};
},
};
</script>
Get current year with Composition API
Of course, this also works when using the Vue 3 Composition API:
<script setup>
const currYear = new Date().getFullYear();
</script>
<template>
<div>
{{ currYear }}
<div>© {{ currYear }} Coding Beauty</div>
</div>
</template>
Get current month
If you also want to get the current month, the getMonth()
method is for you.
getMonth()
returns a zero-based index that represents the month of the Date
. Zero-based here means that 0 = January, 1 = February, 2 = March, etc.
<template>
<div>Month number {{ currMonth }} in {{ currYear }}</div>
</template>
<script>
export default {
data() {
return {
currMonth: new Date().getMonth(),
currYear: new Date().getFullYear(),
};
},
};
</script>
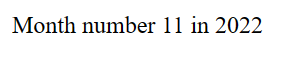
If you want the month name directly (the more likely case), the Date
toLocaleString()
method will do the job.
<template>
<div>{{ currMonth }} in {{ currYear }}</div>
</template>
<script>
export default {
data() {
return {
currMonth: new Date().toLocaleString([], {
month: 'long',
}),
currYear: new Date().getFullYear(),
};
},
};
</script>
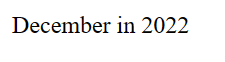
Check out this article for a full guide on how to convert a month number to its equivalent month name in JavaScript.
Get current day of month
Similarly, to get the current day in the month, you’d use the Date
getDate()
method:
<template>
<div>{{ currMonth }} {{ currDay }}, {{ currYear }}</div>
</template>
<script>
export default {
data() {
return {
currDay: new Date().getDate(),
currMonth: new Date().toLocaleString([], {
month: 'long',
}),
currYear: new Date().getFullYear(),
};
},
};
</script>
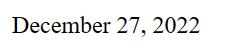
Get current year, month, day, week…
While you could get each component of the date using different functions, a much more flexible and easy way to do this is by formatting the date in the given format with a format specifier.
We can carry out this formatting with the format()
function from the date-fns
library.
In the following example, we use date-fns
format()
to get the multiple individual parts of the date.
<template>
<div>{{ dateString }}</div>
</template>
<script>
import { format } from 'date-fns';
export default {
data() {
return {
dateString: format(
new Date(),
"EEEE, 'the' do 'of' LLLL, yyyy"
),
};
},
computed() {
return {};
},
};
</script>

The format()
function takes a pattern and returns a formatted date string in the format specified by the pattern. You can find a list of the patterns format()
accepts here.
For our example, we use the following patterns:
-
EEEE
: to get the full name of the day of the week. do
: to get the ordinal day of the month, i.e., 1st, 2nd, 3rd, etc.LLLL
: to get the full name of the month of the year.yyyy
: to get the full year.
We also use single quotes to escape strings (the
and of
) that are not patterns but should be included in the result of the formatting.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
