Are you developing a Visual Studio Code extension and experiencing the “Command not found” error? Let’s learn how to fix it in this article.
In this article
- 1. Register command in package.json contributes
- 2. Register command in package.json activationEvents
- 3. Check console logs for any errors
- 4. Register command in extension.ts registerCommand()
- 5. Compile TypeScript source manually
- 6. Upgrade VS Code to match extension version
1. Register command in package.json
contributes
To fix the “Command not found” error in a VS Code extension, make sure your extension registers the command under the contributed
field in your package.json
file:
{
...
"contributes": {
"commands": [ ... ]
}
...
}
2. Register command in package.json
activationEvents
To fix the “Command not found” error in a VS Code extension, make sure you’re added the command to the activationEvents
array in your package.json
file:
{
...
"activationEvents": [
"onCommand:extension.showMyExtension",
"onCommand:extension.callMyExtension"
]
...
}
3. Check console logs for any errors
One common cause of “Command not found” error in a VS Code extension is an undetected JavaScript error. You may find such errors when you examine the VS Code console logs.
Head to the Help
menu and select Toggle Developer Tools
and inspect the console for potential errors.
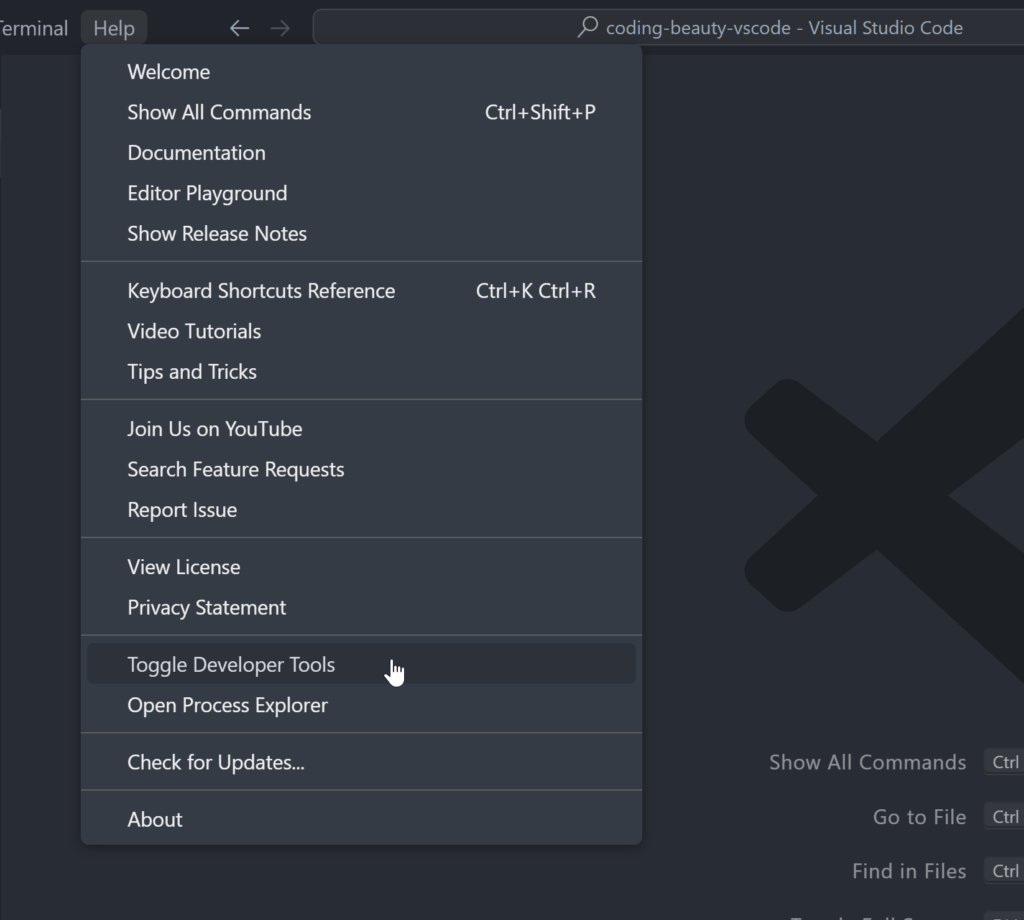
4. Register command in extension.ts
registerCommand()
To fix the “Command not found” error in a VS Code extension, make sure you’re registered the command in extension.ts
using vscode.commands.registerCommand()
:
// ...
export async function activate(context: vscode.ExtensionContext) {
// ...
// commandAction is a callback function
context.subscriptions.push(
vscode.commands.registerCommand('command_name', commandAction)
);
// ...
}
5. Compile TypeScript source manually
The “Command not found” error happens in a VS Code extension if the TypeScript source wasn’t compiled to the out
folder before launch or build.
This typically indicates a problem with your VS Code debug configuration, for example, preLaunchTask
may be missing from launch.json
:
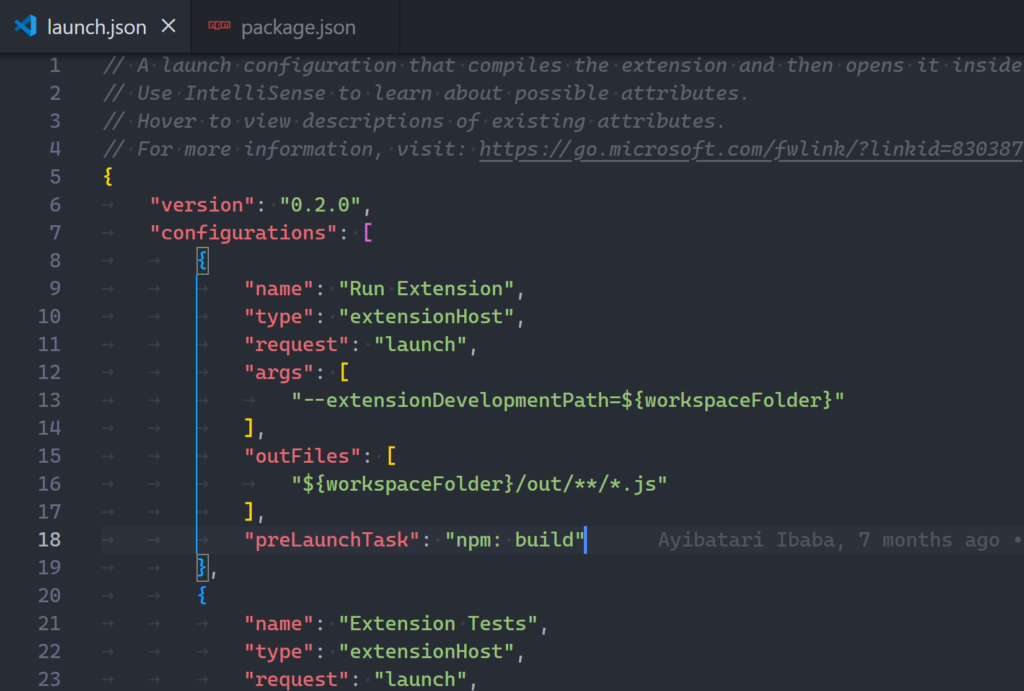
launch.json
should have a preLaunchTask
which builds the TypeScript files automatically.Or there may be a problem with the build
script in package.json
.
{
...
"scripts": {
...
"vscode:prepublish": "npm run esbuild-base -- --minify && npm run build",
"esbuild-base": "rimraf out && esbuild ./ext-src/extension.ts --bundle --outfile=out/extension.js --external:vscode --format=cjs --platform=node",
"build": "webpack && tsc -p tsconfig.extension.json",
...
}
...
}
While you figure out what’s preventing the automatic TypeScript compilation, you can do it yourself with the tsc
command:
# NPM
npx tsc -p .
# Yarn
yarn tsc -p .
# PNPM
pnpm tsc -p .
6. Upgrade VS Code to match extension version
To fix the “Command not found” error in an extension, update VS Code to a version higher that what is specified in the engines.vscode
field of your package.json
file.
Or, downgrade engines.vscode
to a version equal to or lower than the VS Code version you’re using to run the extension.
{
...
"engines": {
"vscode": "^1.80.0"
},
...
}