This new AI tool from Google just destroyed web & UI designers
Wow this is absolutely massive.
The new Stitch tool from Google may have just completely ruined the careers of millions of web & UI designers — and it’s only just get started.
Just check out these stunning designs:
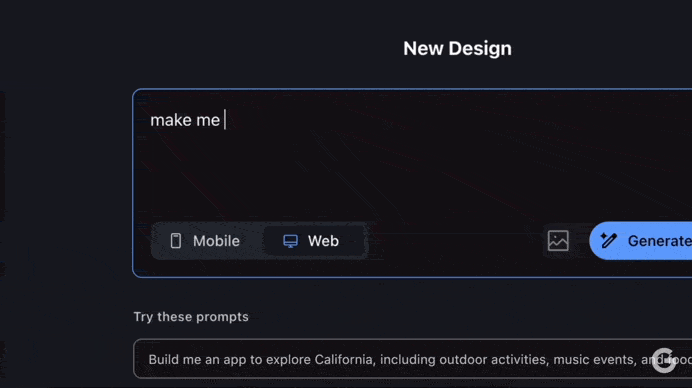
This is an absolute game changer for anyone who’s ever dreamed of building an app but felt intimidated by the whole design thing.
It’s a huge huge deal.
Just imagine you have a classic app idea — photo sharing app, workout app, todo-list whatever…
❌ Before:
You either hire a designer or spend hours wrestling with design software trying to create a pixel perfect UI.
Or maybe you even just try to wing it and hope for the best, making crucial design decisions on the fly as you develop the app.
✅ Now:
Just tell Stitch whatever the hell you’re thinking.
Literally just describe your app in plain English.
“A blue-themed photo-sharing app”:

Look how Stitch let me easily the design — adding likes for every photo:

Or, if you’ve got a rough sketch on a napkin, snap a pic and upload it. Stitch takes your input, whatever it is, and then — BOOM — it generates a visual design for your app’s user interface. It’s like having a personal UI designer at your fingertips.

But it doesn’t stop there. This is where it gets really cool. Stitch doesn’t just give you a pretty picture. It also spits out the actual HTML and CSS code that brings that design to life. Suddenly, your app concept isn’t just an idea; it’s a working prototype. How amazing is that?
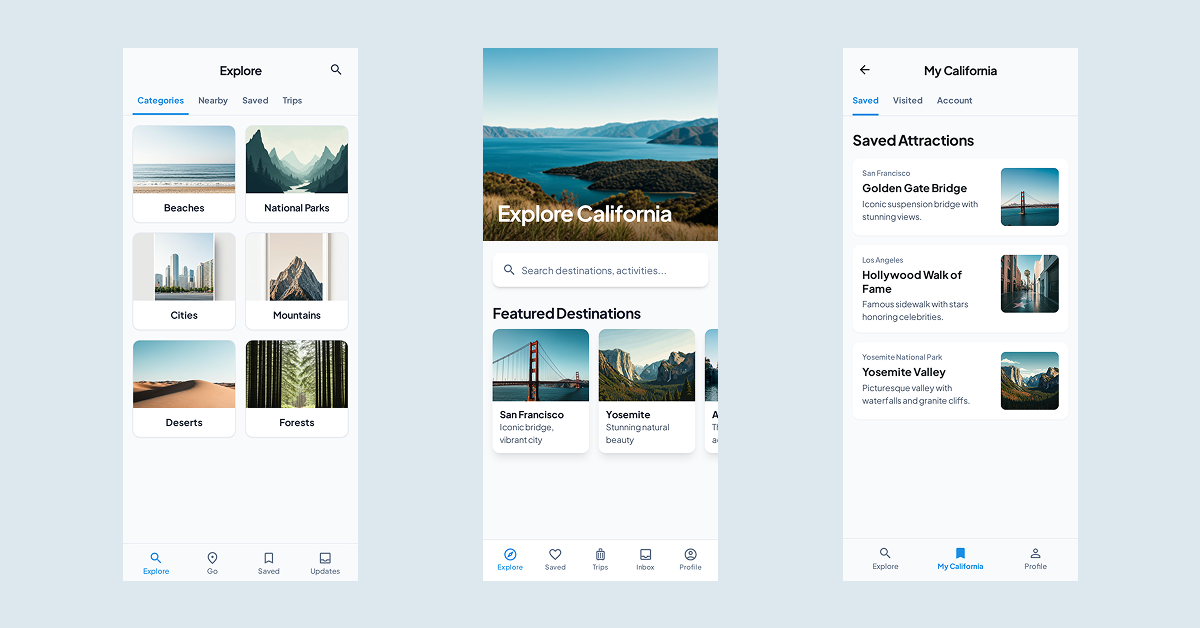
Stitch is pretty smart too. It can give you different versions of your design, so you can pick the one you like best. You can also tweak things – change the colors, switch up the fonts, adjust the layout. It’s incredibly flexible. And if you want to make changes, just chat with Stitch. Tell it what you want to adjust, and it’ll make it happen. It’s a conversation, not a command line.
Behind all this magic are Google’s powerful AI models, Gemini 2.5 Pro and Gemini 2.5 Flash. These are the brains making sense of your ideas and turning them into designs and code. The whole process is surprisingly fast.
Who is this for, you ask? Well, it’s for everyone. If you’re a complete beginner with zero design or coding experience, Stitch is your new best friend. You can create professional-looking apps without breaking a sweat.
It’s a fantastic way to rapidly prototype ideas and get a head start on coding for seasoned developers.
Right now, Stitch is in public beta, available in 212 countries, though it only speaks English for now. And yes, you can use it for free, with a monthly limit on how many designs you can generate.
It’s a super-powered starting gun for your app development journey. It streamlines the early stages to get you from a raw idea to a tangible design and code much faster.
And if you still want more fine-grained control, you can always export your design to Figma.
So, if you’ve got an app idea bubbling in your mind, Google Stitch might just be the tool you’ve been waiting for to bring it to life.