To scroll to an element in React, set a ref on the target element, then call scrollIntoView()
on the target ref.
We can use this approach to scroll to an element when another trigger element is clicked.
We’ll first need to set a click
event listener on the trigger element.
Then we’ll call scrollIntoView()
in the listener.
import { useRef } from 'react';
export default function App() {
const ref = useRef(null);
const handleClick = () => {
ref.current?.scrollIntoView({ behavior: 'smooth' });
};
return (
<div>
<button onClick={handleClick}>Scroll to element</button>
<div style={{ height: '150rem' }} />
<div ref={ref} style={{ backgroundColor: 'lightblue' }}>
Coding Beauty
</div>
<div style={{ height: '150rem' }} />
</div>
);
}
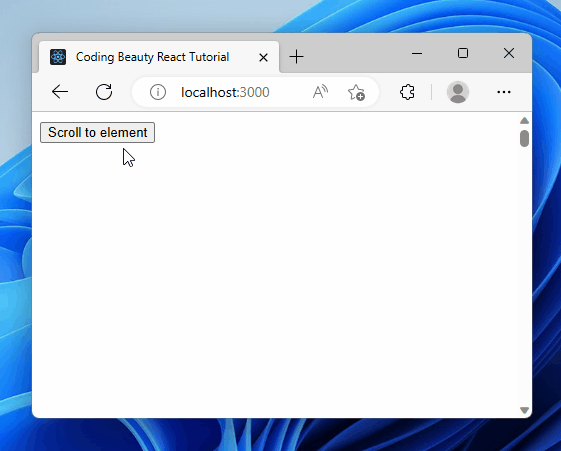
Scrolling on click is great for quick navigation.
It improves the user experience.
It’s great for long lists and pages with many sections.
It makes apps more interactive with smooth-scrolling animations.
We create a ref object with the useRef
hook and assign it to the ref
prop of the target div
element.
Doing this sets the current
property of the ref object to the DOM object that represents the element.
useRef
returns a mutable object that maintains its value when a component updates.
Also, modifying the value of this object’s current
property doesn’t cause a re-render.
This is unlike the setState
update function return from the useState
hook.
We use the onClick
on the button to a click listener to it. So, this listener will be called when the user clicks the button.
const handleClick = () => {
ref.current?.scrollIntoView({ behavior: 'smooth' });
};
In the handleClick
listener, we call the scrollIntoView()
method on the ref of the target div
element to scroll down to it and display it to the user.
We set the behavior
option to smooth
to make the element scroll into view in an animated way, instead of jumping straight to the element in the next frame – auto
.
auto
is the default.
Scroll to dynamic element on click in React
We can do something similar to scroll to a dynamically created element in React:
import { useRef, useState } from 'react';
const allFruits = [
'Apples',
'Bananas',
'Oranges',
'Grapes',
'Strawberries',
'Blueberries',
'Pineapples',
'Mangoes',
'Kiwis',
'Watermelons',
];
export default function App() {
const ref = useRef(null);
const [fruits, setFruits] = useState([...allFruits.slice(0, 3)]);
const addFruit = () => {
setFruits((prevFruits) => [...prevFruits, allFruits[prevFruits.length]]);
};
const scrollToLastFruit = () => {
const lastChildElement = ref.current?.lastElementChild;
lastChildElement?.scrollIntoView({ behavior: 'smooth' });
};
return (
<div>
<div
style={{
position: 'fixed',
backgroundColor: 'white',
bottom: 0,
marginBottom: 10,
}}
>
<button onClick={addFruit}>Add fruit</button>
<button onClick={scrollToLastFruit} style={{ marginLeft: '8px' }}>
Scroll to last
</button>
</div>
<div style={{ height: '5rem' }} />
<div ref={ref}>
{fruits.map((fruit) => (
<h2 key={fruit}>{fruit}</h2>
))}
</div>
<div style={{ height: '150rem' }} />
</div>
);
}
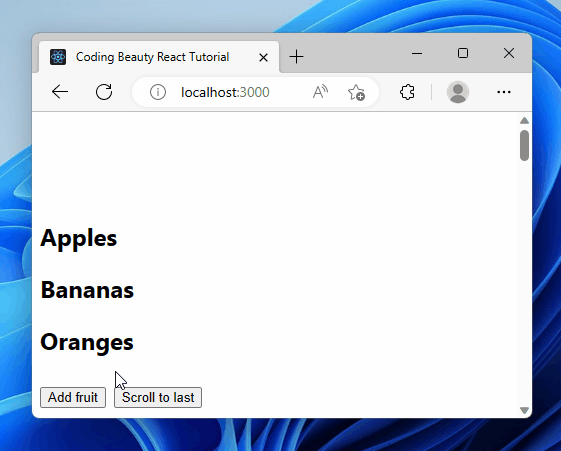
Here we have a list of fruits displayed.
The Add fruit
button dynamically adds an item to the fruit list on click.
Then the Scroll to last
button scrolls to this item on click, as it’s the last in the list.
Like before, we use the onClick
prop to set a click event listener on the button.
This time we set the ref on the list instead of the items since the items are created dynamically, and the last item will not be constant.
Doing this sets the current
property of the ref object to the DOM object that represents the list element.
In this listener, we use the lastElementChild
property of the list element to get its last item element. Then we call scrollIntoView()
on this last item to scroll down to it.
const scrollToLastFruit = () => {
const lastChildElement = ref.current?.lastElementChild;
lastChildElement?.scrollIntoView({ behavior: 'smooth' });
};
Scroll to element after render in React
To scroll to an element after render on React:
- Set a ref on the element.
- Create a
useEffect
hook to run code just after the component mounts. - Call
scrollIntoView()
on the ref object inuseEffect
.
import { useEffect, useRef } from 'react';
export default function App() {
const ref = useRef(null);
const scrollToElement = () => {
ref.current?.scrollIntoView({ behavior: 'smooth' });
};
useEffect(() => {
scrollToElement();
}, []);
return (
<div>
<div style={{ height: '150rem' }} />
<div ref={ref} style={{ backgroundColor: 'blue', color: 'white' }}>
Coding Beauty
</div>
<div style={{ height: '150rem' }} />
</div>
);
}
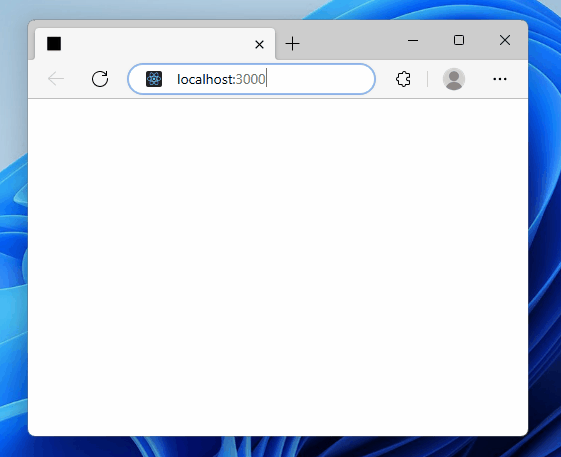
Scrolling after page loads help users find info.
It also helps fix errors.
It’s great for those who use special software.
It aids web navigation.
Like before, we create a ref object with the useRef
hook and assign it to the ref
prop of the target div
element.
Doing this sets the current
property of the ref object to the DOM object that represents the element.
useRef
returns a mutable object that maintains its value when a component updates.
Also, modifying the value of this object’s current
property doesn’t cause a re-render.
This is unlike the setState
update function return from the useState
hooks.
The useEffect
hook performs an action after the component renders, and when one or more of its dependencies change.
We passed an empty dependencies array ([]
) to make sure that action only runs when the component mounts.
In useEffect
, we call the scrollIntoView()
method on the ref of the target div
element to scroll down to it and display it to the user.
We set the behavior
option to smooth
to make the element scroll into view in an animated way, instead of jumping straight to the element in the next frame (auto
).
auto
is the default.
Key takeaways
In React, we can use refs to scroll to a specific element on the page.
We can set a click event listener on a trigger element and call scrollIntoView()
on the target ref to scroll to the desired element.
useRef()
returns a mutable object that maintains its value when a component updates, allowing us to reference DOM elements.
Setting a ref on a list container allows us to dynamically scroll to the last item in the list.
We can use useEffect()
to scroll to an element after the component mounts, improving the user experience and aiding web navigation.
Setting the behavior
option to smooth
in scrollIntoView()
produces a smooth scrolling animation instead of an abrupt jump to the element.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
