In this article, we’re going to learn how to easily remove an item from a state array in React.
The Array filter() Method
To remove an item from a state array in React, call the filter() method on the array, specifying a test that every item in the array apart from the one to be removed will pass, then update the state with the result of filter()
with setState
.
import { useState } from 'react';
export default function App() {
const initialState = [
{ id: 1, name: 'Banana', amount: 5 },
{ id: 2, name: 'Apple', amount: 6 },
];
const removeSecond = () => {
setFruits((current) =>
current.filter((fruit) => fruit.id !== 2)
);
};
const [fruits, setFruits] = useState(initialState);
return (
<div style={{ margin: '16px' }}>
<button onClick={removeSecond}>Remove second</button>
{fruits.map((fruit) => (
<div key={fruit.id}>
<h2>Name: {fruit.name}</h2>
<h2>Amount: {fruit.amount}</h2>
<hr />
</div>
))}
</div>
);
}
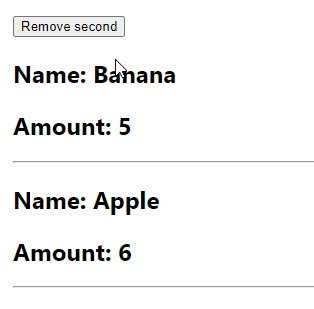
We remove the fruit object with the id
2
by returning a condition from the filter()
callback that is true
only for the items in the array that don’t have an id
of 2. Doing this excludes the target item from the array returned from filter()
.
const initialState = [
{ id: 1, name: 'Banana', amount: 5 },
{ id: 2, name: 'Apple', amount: 6 },
];
const secondRemoved = initialState.filter((fruit) => fruit.id !== 2);
// [ { id: 1, name: 'Banana', amount: 5 } ]
console.log(secondRemoved);
Since App
here is a functional component, we use the useState()
React hook to create the initial state array. The first value useState()
returns lets us access the state data. The second value is a function used to update the state (generically called setState
). We pass a function to setState
(named setFruits
here) to ensure that we get the current/latest state.
const removeSecond = () => {
// "current" contains the latest state array
setFruits((current) =>
current.filter((fruit) => fruit.id !== 2)
);
};
Tip: Always pass a function to setState
when the new state is computed from the current state data.
Don’t Modify State Directly in React
Note that trying to remove the item from the array by modifying it directly using a function like splice()
will not work:
const removeSecond = () => {
// Find the index of the object to be removed
const secondIndex = fruits.findIndex((fruit) => fruit.id === 2);
// Mutating the array like this will not update the view
fruits.splice(secondIndex, 1);
};
State is meant to be immutable in React, so we can’t update the array by mutating it. It has to be replaced with a new array returned from filter()
for the view to update.
Remove Items from a State Array Based on Multiple Conditions
If you need to remove an item from the state array based on multiple conditions, you can use the logical AND (&&) or logical OR (&&) operator.
Using the Logical OR (||) Operator
Here is an example of using the logical OR (||
) operator to remove an item from a state array.
const initialState = [
{ id: 1, name: 'Banana', amount: 5 },
{ id: 2, name: 'Apple', amount: 6 },
{ id: 3, name: 'Orange', amount: 10 },
{ id: 4, name: 'Watermelon', amount: 1 },
];
const [fruits, setFruits] = useState(initialState);
const remove = () => {
setFruits((current) =>
current.filter(
(fruit) =>
fruit.name === 'Orange' || fruit.name === 'Apple'
)
);
};
Combining a set of operands with the ||
operator will result in true
if at least one of the operands evaluates to true
. By using this operator with the filter()
method, we return a new array containing only the fruit objects with a name
equal to 'Orange'
or 'Apple'
.
Using the Logical AND (&&) Operator
Here is an example of using the logical AND (&&
) operator to remove an item from a state array:
const initialState = [
{ id: 1, name: 'Banana', amount: 5 },
{ id: 2, name: 'Apple', amount: 6 },
{ id: 3, name: 'Orange', amount: 10 },
{ id: 4, name: 'Watermelon', amount: 1 },
];
const [fruits, setFruits] = useState(initialState);
const remove = () => {
setFruits((current) =>
current.filter(
(fruit) => fruit.id !== 2 && fruit.id !== 4
)
);
};
Combining operands with the &&
operator will result in true
only if all the operands are true
.
By combining the filter()
method with the &&
operator, we are able to specify a compound boolean expression that removes the fruit objects with id
s of 2
and 4
.
Simplifying Compound Boolean Expressions with De Morgan’s Laws
We can use one of De Morgan’s laws to convert the AND condition from our previous example to an OR condition and reduce the number of negations.
const remove = () => {
setFruits((current) =>
current.filter(
(fruit) => !(fruit.id === 2 || fruit.id === 4)
)
);
};
This transformation could make the expression easier to read and understand.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.

Great post 👈
Thanks, I’m glad you liked it.