To capitalize the first letter of each word in a string in React:
- Split the string into an array of words with
.split('')
. - Iterate over the words array with
.map()
. - For each word, return a new word that is an uppercase form of the word’s first letter added to the rest of the word, i.e.,
word.charAt(0).toUpperCase() + word.slice(1)
. - Join the words array into a string with
.join(' ')
.
For example:
export default function App() {
const capitalizeWords = (str) => {
return str
.toLowerCase()
.split(' ')
.map((word) => word.charAt(0).toUpperCase() + word.slice(1))
.join(' ');
};
const str1 = 'coding BEAUTY';
const str2 = 'LEARNING javascript';
return (
<div>
<b>{str1}</b>
<br />
Capitalized: <b>{capitalizeWords(str1)}</b>
<br />
<br />
<b>{str2}</b>
<br />
Capitalized:
<b>{capitalizeWords(str2)}</b>
</div>
);
}
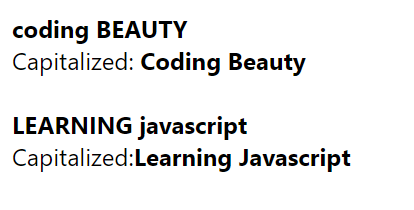
The capitalizedWords()
function takes a string and returns a new string with all the words capitalized.
First, we use the toLowerCase()
method to lowercase the entire string, ensuring that only the first letter of each word is uppercase.
// coding beauty
console.log('coding BEAUTY'.toLowerCase());
Tip: If it’s not necessary for the remaining letters in each word to be lowercase, you can remove the call to the toLowerCase()
method.
Then we call the String
split()
method on the string to split all the words into an array.
// [ 'welcome', 'to', 'coding', 'beauty' ]
console.log('welcome to coding beauty'.split(' '));
After creating the array of words, we call the map()
method on it, with a callback function as an argument. This function is called by map()
and returns a result for each word in the array.
In the function, we get the word’s first character with charAt()
, convert it to uppercase with toUpperCase()
, and concatenate it with the rest of the string.
We use the String
slice()
method to get the remaining part of the string. Passing 1
to slice()
makes it return the portion of the string from the second character to the end.
Note: String (and array) indexing is zero-based JavaScript, so the first character in a string is at index 0, the second at 1, and the last at str.length-1
Lastly, we concatenate the words into a single string, with the Array
join()
method
Passing a space (' '
) to join()
separates the words by a space in the resulting string.
// Welcome To Coding Beauty
console.log(['Welcome', 'To', 'Coding', 'Beauty'].join(' '));
After creating the capitalizeWords()
function, we call it as the component is rendered by wrapping it in curly braces ({ }
) in our JSX code.
return (
<div>
<b>{str1}</b>
<br />
Capitalized: <b>{capitalizeWords(str1)}</b>
<br />
<br />
<b>{str2}</b>
<br />
Capitalized:
<b>{capitalizeWords(str2)}</b>
</div>
);
The function is invoked and its result is rendered at the point where the curly braces are located.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
