Are you experiencing the “Cannot find module” or MODULE_NOT_FOUND error in your Node.js project?
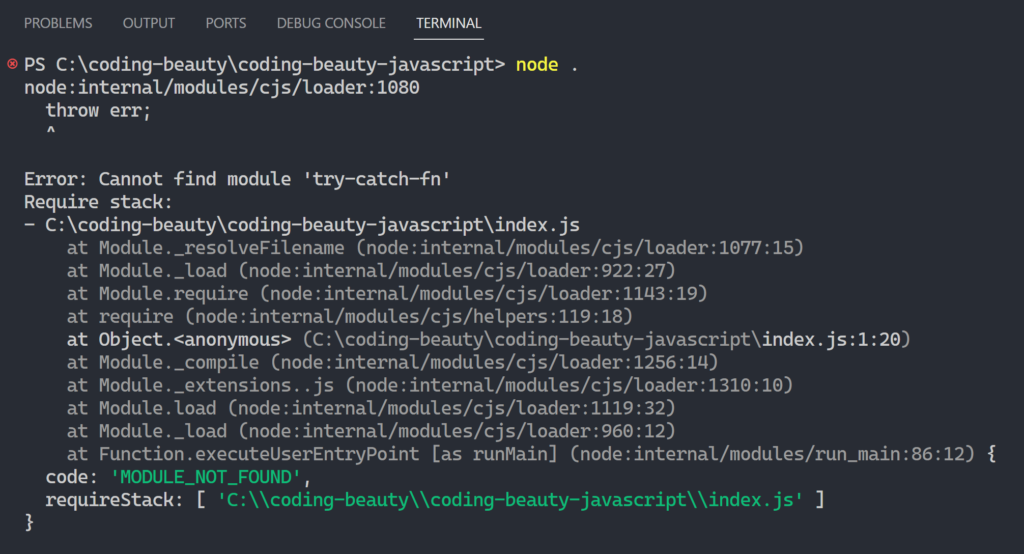
This error happens when your IDE can’t detect the presence of a particular NPM package. Let’s see how easy it is to fix.
In this article
- 1. Ensure NPM package installed
- 2. Install package again
- 3. Reinstall all packages
- 4. TypeScript: Install type definitions
- 5. Ensure package.json main file exists
- 6. npm link package
- 7. Ensure correct NODE_PATH
1. Ensure NPM package installed
To fix the “Cannot find module” error in Node.js, make sure the NPM package is installed and present in your package.json
file.
You can install a package from NPM with the npm i
command, for example:
# NPM
npm i nextjs-current-url
# Yarn
yarn add nextjs-current-url
# PNPM
pnpm add nextjs-current-url
After installation, the package will included under the dependencies
field in package.json
{
...
"dependencies": {
...
"nextjs-current-url": "^1.0.1"
...
}
...
}
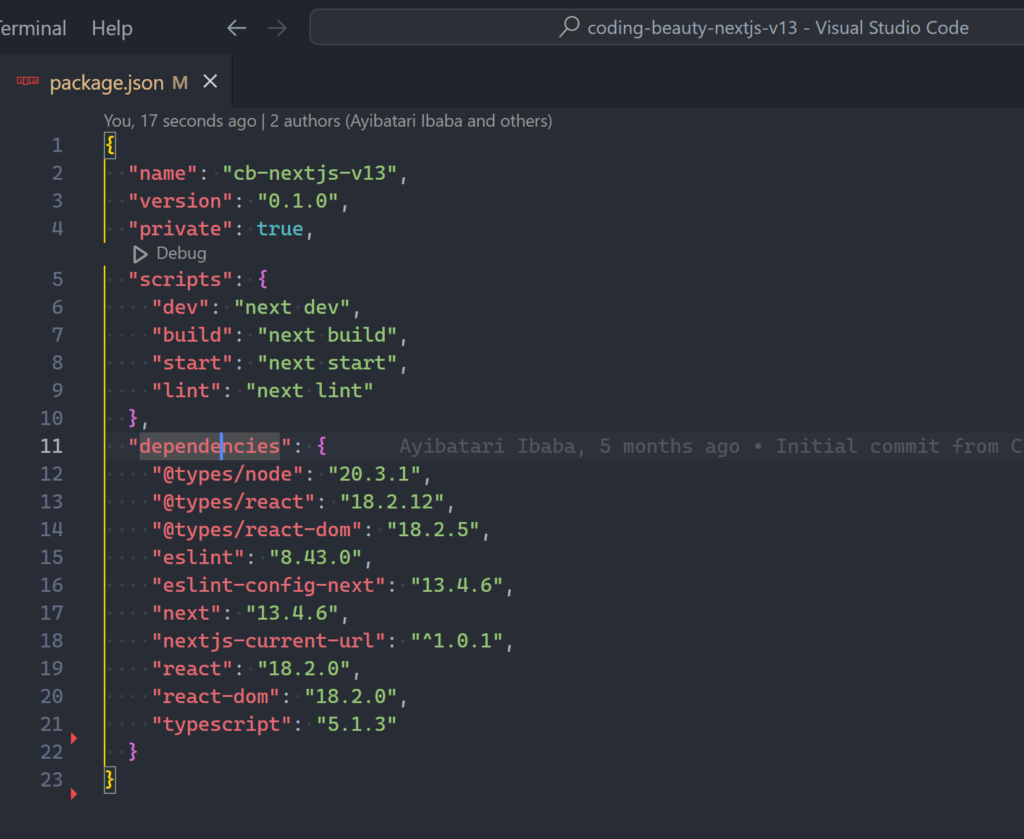
You can also install the package as a development dependency, which indicates that the package is only used for development, and won’t be needed by the app itself. Packages like nodemon
and ts-node
fit this category:
# NPM
npm i -D ts-node
# Yarn
yarn add -D ts-node
# PNPM
pnpm add -D ts-node
It will be part of devDependencies
in package.json after installation:
{
...
"devDependencies": {
...
"ts-node": "^10.9.1"
...
}
}
2. Install package again
To fix the MODULE_NOT_FOUND error in Node.js, trying installing the package in your project once again, even if you did so earlier:
# NPM
npm i -D try-catch-fn
# Yarn
yarn add try-catch-fn
# NPM
yarn add try-catch-fn
3. Reinstall all packages
Try removing the NPM packages installed in your project and reinstalling them again, to fix the “Cannot find module” error.
You can do this with the following command sequence:
# NPM
rm package-lock.json
rm node_modules -r
npm cache clear
npm install
# Yarn
rm yarn.lock
rm node_modules -r
yarn cache clean
yarn install
# PNPM
rm pnpm-lock.yaml
rm node_modules -r
pnpm store prune
pnpm install
npm cache clear
helps to rid the package manager cache of any corrupted module files.
4. TypeScript: Install type definitions
The MODULE_NOT_FOUND error will occur when you import a package that doesn’t have any detected type definitions into a TypeScript file.
If it’s a core Node.js module like http
or fs
, you may need to add "node"
to compilerOptions.types
in your tsconfig.json
file:
{
// ...
"compilerOptions": {
// ...
"types": [
"node"
]
// ...
},
// ...
}
If it’s a third-party module, installing the type definitions from NPM should help. For example:
npm i @types/express
5. Ensure package.json
main
file exists
You may encounter the “Cannot find module” error in Node.js if the main
field of your package.json
file doesn’t exist.
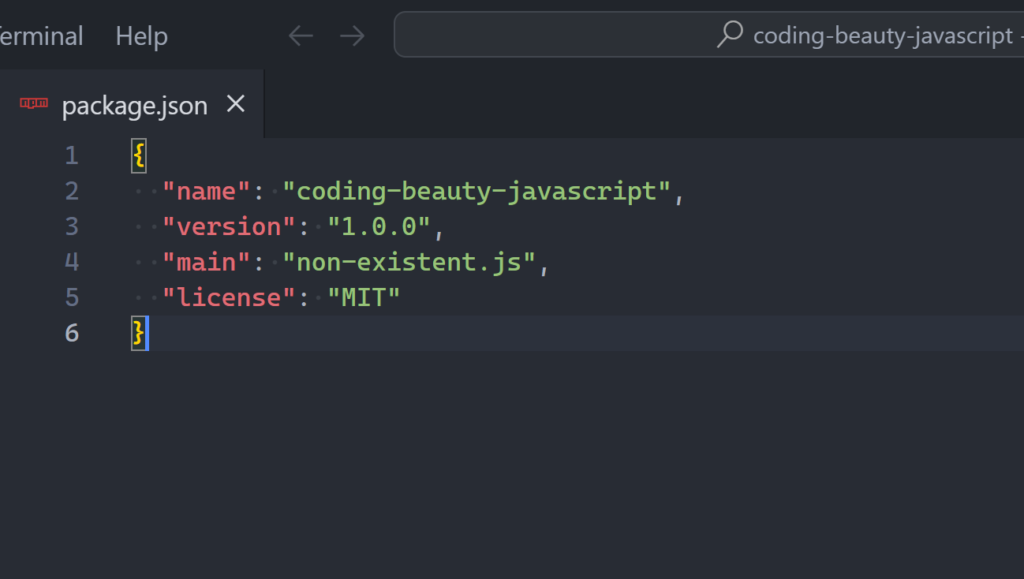
6. npm link
package
You can also try the npm link
command on the package to fix the MODULE_NOT_FOUND error, for example:
npm link create-react-app
npm link webpack
npm link
is a command that connects a globally installed package with a local project using a symbolic link.
It enables working on a package locally without publishing it to the npm registry or reinstalling it for every change. Executing npm link
in the package directory establishes a symbolic link in the global node_modules
directory, directing to the local package.
Afterwards, npm link <package-name>
can be used in the project to link the global package with your local project.
7. Ensure correct NODE_PATH
In older Node.js versions, you may be able to fix the “Cannot find module” error by setting the NODE_PATH
environment variable to correct node_modules
installation folder.
NODE_PATH
is a string of absolute paths separated by colons used by Node.js to locate modules when they can’t be found elsewhere.
It was initially created to enable the loading of modules from different paths when there was no defined module resolution algorithm.
And it’s still supported, but it’s not as important anymore since the we’ve established a convention for finding dependent modules in Node.js community.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
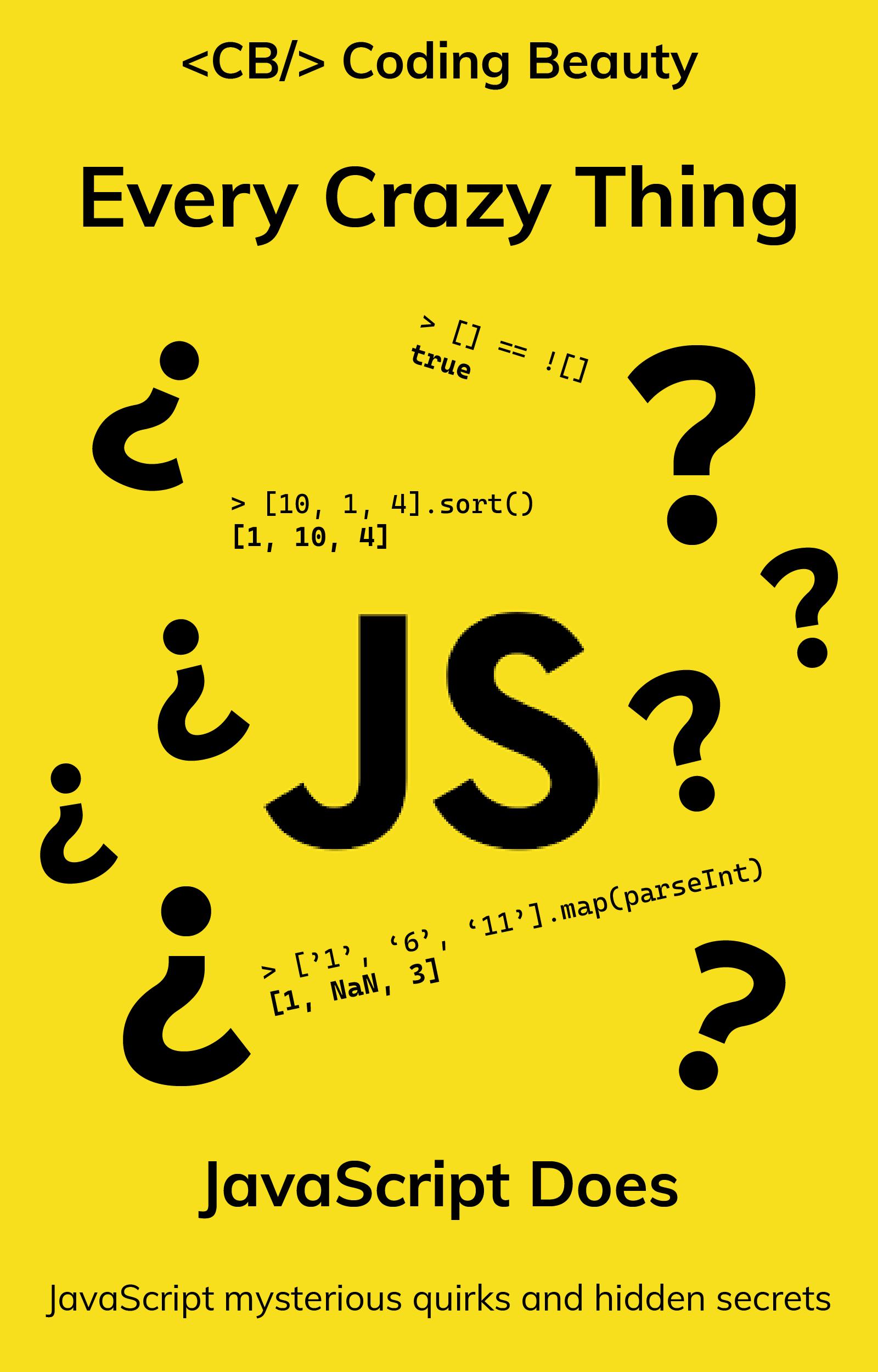