Let’s learn how to easily subtract any number of minutes from a Date
object in JavaScript.
1. Date
getMinutes()
and setMinutes()
methods
To subtract minutes from a Date
:
- Call the
getMinutes()
method on theDate
to get the minutes. - Subtract the minutes.
- Pass the difference to the
setMinutes()
method.
For example:
function subtractMinutes(date, minutes) {
date.setMinutes(date.getMinutes() - minutes);
return date;
}
// 2:20 pm on May 18, 2022
const date = new Date('2022-05-18T14:20:00.000Z');
const newDate = subtractMinutes(date, 10);
// 2:10 pm on May 18, 2022
console.log(newDate); // 2022-05-18T14:10:00.000Z
Our subtractMinutes()
function takes a Date
object and the number of minutes to subtract as arguments. It returns the same Date
object with the minutes subtracted.
The Date
getMinutes()
method returns a number between 0
and 59
that represent the minutes of a particular Date
.
The Date
setMinutes()
method set the minutes of a Date
to a specified number.
If the minutes subtracted decrease the hour, day, month, or year of the Date
object, setMinutes()
will automatically update the information in the Date
to reflect this.
// 10:10 am on March 13, 2022
const date = new Date('2022-03-13T10:10:00.000Z');
date.setMinutes(date.getMinutes() - 30);
// 9:40 am on March 13, 2022
console.log(date); // 2022-05-18T14:10:00.000Z
In this example, decreasing the minutes of the Date
by 30 rolls the hour back by 1.
Avoiding side effects
The setMinutes()
method mutates the Date
object it is called on. This introduces a side effect into our subtractMinutes()
function. To avoid modifying the passed Date
and create a pure function, make a copy of the Date
and call setMinutes()
on this copy, instead of the original.
function subtractMinutes(date, minutes) {
// 👇 make copy with "Date" constructor
const dateCopy = new Date(date);
dateCopy.setMinutes(date.getMinutes() - minutes);
return dateCopy;
}
const date = new Date('2022-02-20T11:25:00.000Z');
const newDate = subtractMinutes(date, 5);
// 11:20 am on Feb 20, 2022
console.log(newDate); // 2022-02-20T11:20:00.000Z
// 👇 Original not modified
console.log(date); // 2022-02-20T11:25:00.000Z
Tip: Functions that don’t modify external state (i.e., pure functions) tend to be more predictable and easier to reason about, as they always give the same output for a particular input. This makes it a good practice to limit the number of side effects in your code.
2. date-fns subMinutes()
function
Alternatively, we can use the subMinutes()
function from the date-fns NPM package to quickly subtract minutes from a Date
in JavaScript. It works like our pure subtractMinutes()
function.
import { subMinutes } from 'date-fns';
const date = new Date('2022-10-03T20:50:00.000Z');
const newDate = subMinutes(date, 25);
// 8:25 pm on October 3, 2022
console.log(newDate); // 2019-02-20T11:20:00.000Z
// Original not modified
console.log(date); // 2022-02-20T11:25:00.000Z
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
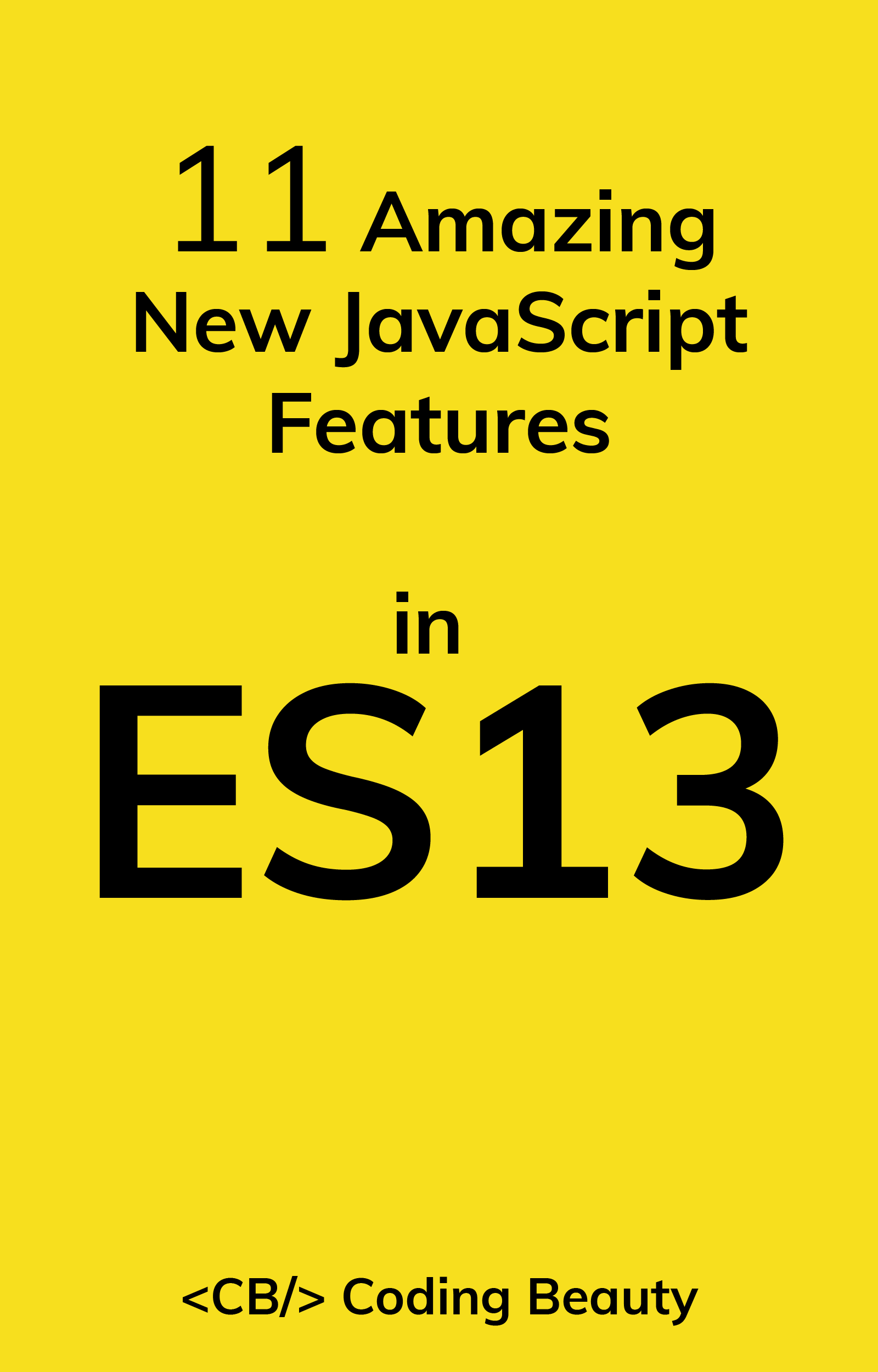