The “structuredClone is not defined” error occurs when you try to use the structuredClone()
/ method in JavaScript, but it’s not defined.
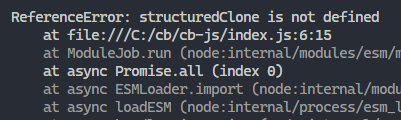
To fix it, install Node.js 17 or a newer version. Once you’ve updated Node.js, you can use structuredClone()
to clone objects with all their properties and methods.
What causes the “structuredClone is not defined” error?
If you try to use the structuredClone()
method in a script that’s running with a Node.js version lower than 17, you will encounter this error.
The structuredClone()
method is used to create a deep copy of an object. It’s a built-in function in JavaScript and is used to clone an object with all its properties and methods.
But when the structuredClone()
method is not defined, it means that the server environment doesn’t recognize the function and cannot perform the action.
Fix: update Node.js
To fix the “structuredClone is not defined” error in JavaScript, you need to install Node.js 17 or a newer version. If you’re using an older version of Node.js, it will throw an error when you try to use structuredClone()
.
Install from website
To download Node.js, visit the official website and opt for the LTS version, as it offers superior stability. As of the time of writing this article, the most recent LTS release of Node.js is v18.15.0.
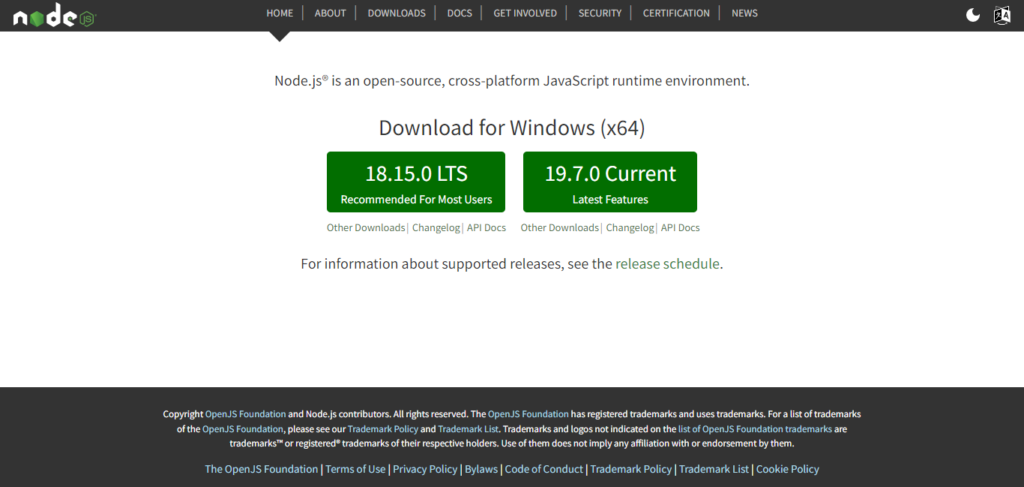
Install with Chocolatey
If you’re using Chocolatey, Node.js is available as the nodejs
package, meaning you can easily install it in a terminal using the following command.
# Use current LTS version
choco install nodejs --version=18.5.0
After installing, you can use the structuredClone
method to clone an object:
const obj = { name: 'Mike', friends: [{ name: 'Sam' }] };
const clonedObj = structuredClone(obj);
console.log(obj.name === clonedObj); // false
console.log(obj.friends === clonedObj.friends); // false
Key takeaways
If you get the “structuredClone
is not defined” error when using the structuredClone()
method in JavaScript, it means that the method is unavailable. To fix the problem, update your Node.js to a version newer than 17. You can get the latest version from the official Node.js website or install the nodejs package using Chocholatey.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
