Let’s look at some ways to reverse the order of an array without mutating the original in JavaScript.
1. slice() and reverse()
To reverse an array without modifying the original, we can clone the array with the slice()
method and reverse the clone with the reverse()
method.
For example:
const arr = [1, 2, 3];
const reversed = arr.slice().reverse();
console.log(reversed); // [ 3, 2, 1 ]
console.log(arr); // [ 1, 2, 3 ]
The reverse()
method reverses an array in place, so we call the slice()
method on the array with no arguments, to return a copy of it.
2. Spread Operator and reverse()
In ES6+, we could also clone the array by using the spread syntax to unpack its elements into a new array, before calling reverse()
:
const arr = [1, 2, 3];
const reversed = [...arr].reverse();
console.log(reversed); // [ 3, 2, 1 ]
console.log(arr); // [ 1, 2, 3 ]
3. Reverse for loop
We can also reverse an array without modifying it by creating a reverse for
loop, and in each iteration, adding the array element whose index is the current value of the loop counter to a new array. The new array will contain all the elements reversed at the end of the loop.
const arr = [1, 2, 3];
const reversed = [];
for (let i = arr.length - 1; i >= 0; i--) {
reversed.push(arr[i]);
}
console.log(arr); // [ 1, 2, 3 ]
console.log(reversed); // [ 3, 2, 1]
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
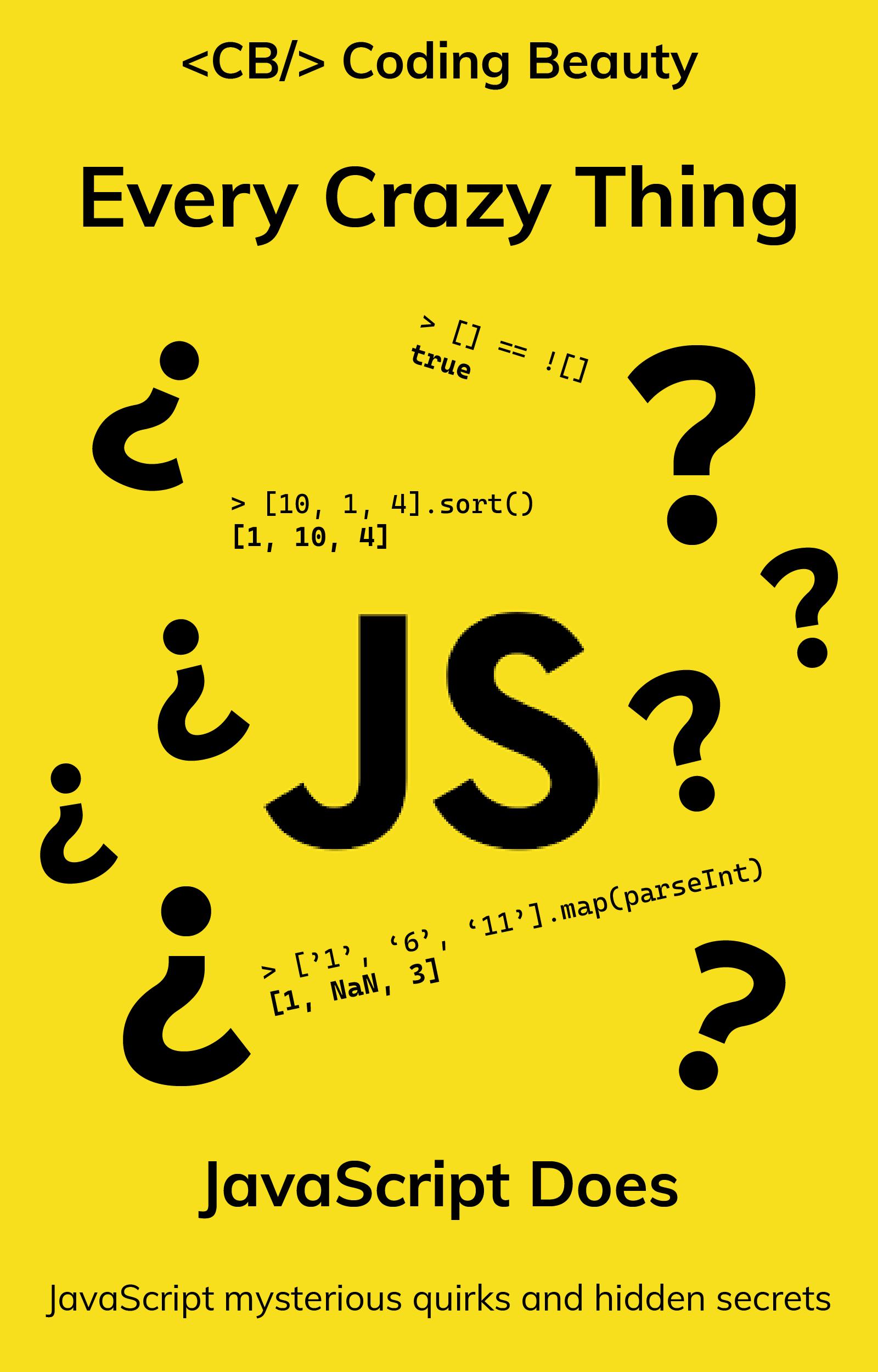