To rename an object key in JavaScript, assign the value of the old key property to a new property with the new key, then delete the old key property.
const obj = { oldKey: 'value' };
obj['newKey'] = obj['oldKey'];
delete obj['oldKey'];
console.log(obj); // { newKey: 'value' }
In this example, we create the new property by direct assignment to the object indexed with the new key. We delete the old key property with the delete
operator.
Note: We can also use the Object.assign()
method to create a new property in the object:
const obj = { oldKey: 'value' };
Object.assign(obj, { newKey: obj.oldKey })['oldKey'];
delete obj['oldKey'];
console.log(obj); // { newKey: 'value' }
This allows us to rename the object key in a single statement:
const obj = { oldKey: 'value' };
delete Object.assign(obj, { newKey: obj.oldKey })['oldKey'];
console.log(obj); // { newKey: 'value' }
The first argument passed to Object.assign()
is the target object that the properties of the sources are applied to. The rest arguments are one or more source objects that contain the properties to apply to the target.
Note: To ensure that the new key property behaves identically to the old key property, use Object.defineProperty()
and Object.getOwnPropertyDescriptor()
to create the new property with the same descriptor as the old key property before deleting it. For example:
const obj = { oldKey: 'value' };
Object.defineProperty(
obj,
'newKey',
Object.getOwnPropertyDescriptor(obj, 'oldKey')
);
delete obj['oldKey'];
console.log(obj); // { newKey: 'value' }
Now the new key property will retain certain characteristics of the old key property, such as enumerability and writability.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
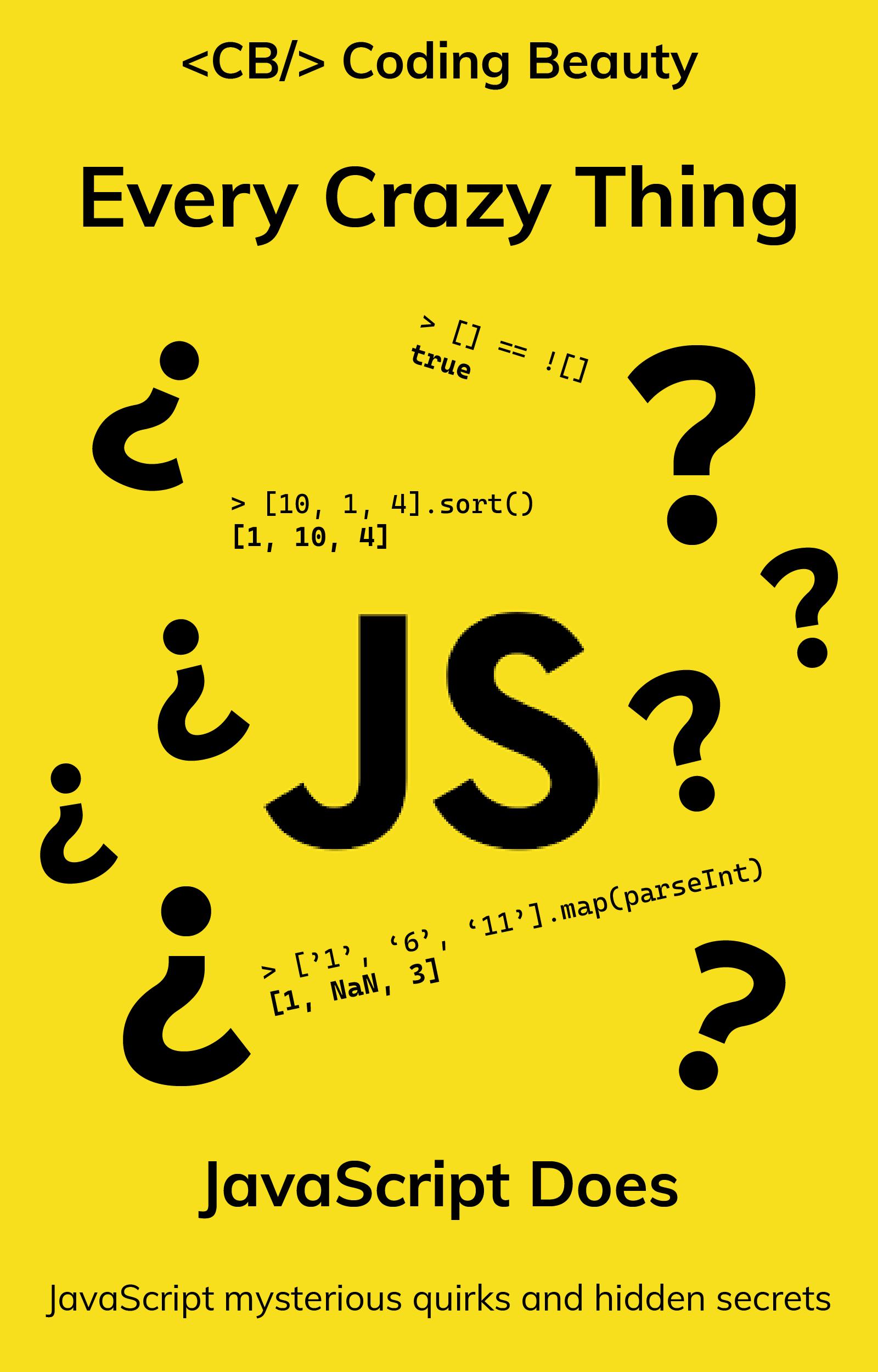