Remove empty strings from array with filter()
method
To remove empty strings from an array in JavaScript, call the filter()
method on the array, passing a callback that returns true
for every element in the array that is not an empty string. The filter()
method will return a new array excluding the empty strings.
For example:
const arr = ['c', '', 'o', '', '', 'd', '', 'e'];
const noEmptyStrings = arr.filter((str) => str !== '');
console.log(noEmptyStrings); // [ 'c', 'o', 'd', 'e' ]
The Array
filter()
method creates a new array filled with elements that pass a test specified by a callback function. It doesn’t modify the original array.
const arr = [1, 2, 3, 4];
const filtered = arr.filter((num) => num > 2);
console.log(filtered); // [ 3, 4 ]
In our example, each element in the array will only pass the test if it is not an empty string (''
). So no empty strings are included in the resulting array.
Remove empty strings from array with loop
Here’s an alternative way to remove empty strings from an array in JavaScript.
const arr = ['c', '', 'o', '', '', 'd', '', 'e'];
const noEmptyStrings = [];
for (const item of arr) {
if (item !== '') {
noEmptyStrings.push(item);
}
}
console.log(noEmptyStrings); // [ 'c', 'o', 'd', 'e' ]
We create an array variable (noEmptyStrings
) that will store the elements in the original array that are not empty strings. Then we use a for..of
loop to iterate over the array. For each element, we check if it is a non-empty string, using an if
statement. If it is, we add it to the array variable. At the end of the loop, we’ll have an array containing no empty strings.
Either method is okay, the main difference between them is that the first is declarative and functional. It’s a short one-liner that uses a pure function and is focused on the result. But the second method is imperative, as it emphasizes the steps taken to get that result.
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
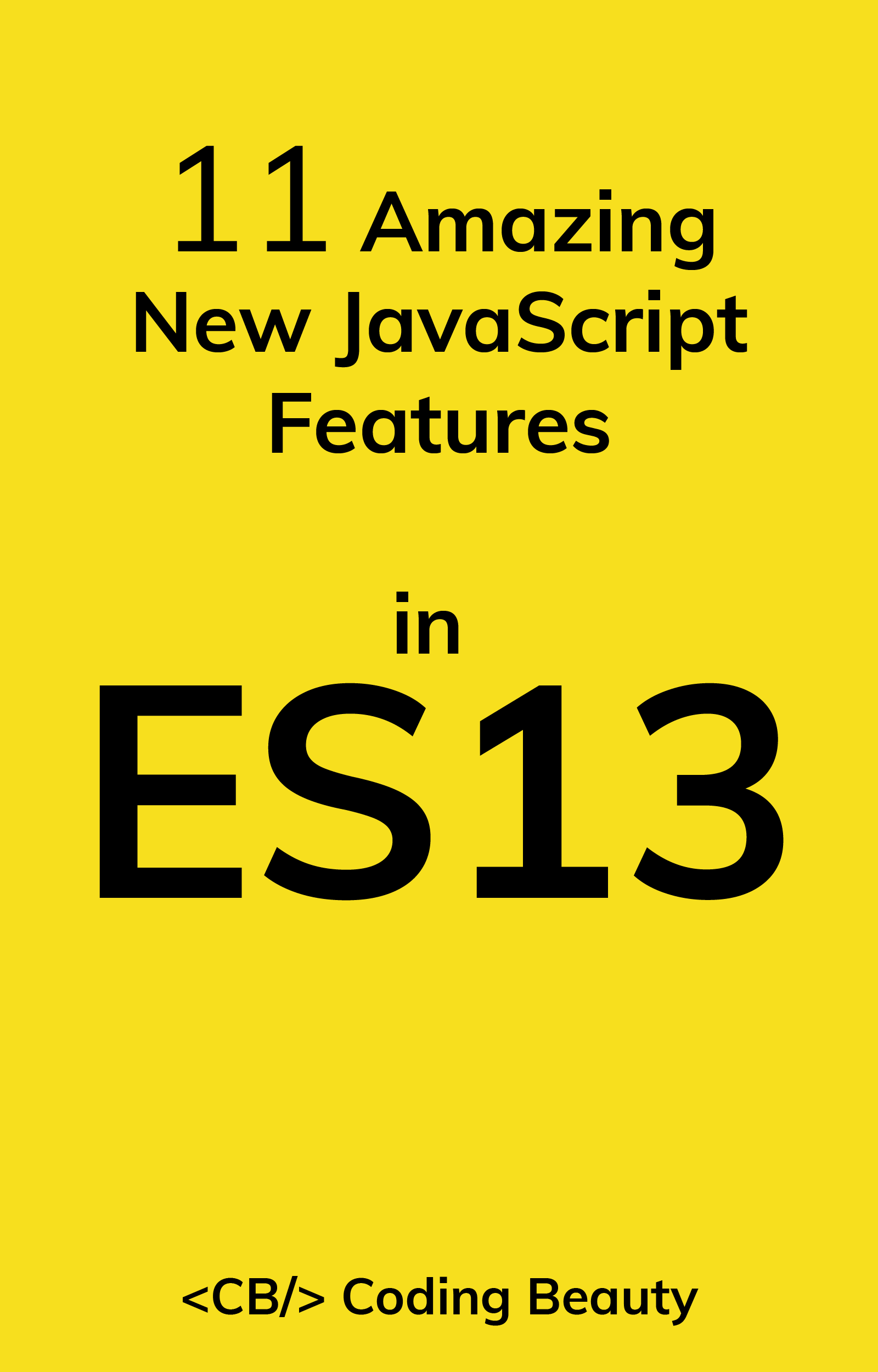