The static String
raw()
method is so named as we can use it to get the raw string form of template literals in JavaScript. This means that variable substitutions (e.g., ${num}
) are processed but escape sequences like \n
and \t
are not.
For example:
const message = String.raw`\n is for newline and \t is for tab`;
console.log(message); // \n is for newline and \t is for tab
We can use a raw string to avoid the need to use double backslashes for file paths and improve readability.
For example, instead of:
const filePath = 'C:\\Code\\JavaScript\\tests\\index.js';
console.log(`The file path is ${filePath}`); // The file path is C:\Code\JavaScript\tests\index.js
We can write:
const filePath = String.raw`C:\Code\JavaScript\tests\index.js`;
console.log(`The file path is ${filePath}`); // The file path is C:\Code\JavaScript\tests\index.js
We can also use it to write clearer regular expressions that include the backslash character. For example, instead of:
const patternString = 'The (\\w+) is (\\d+)';
const pattern = new RegExp(patternString);
const message = 'The number is 100';
console.log(pattern.exec(message)); // ['The number is 100', 'number', '100']
We can write:
const patternString = String.raw`The (\w+) is (\d+)`;
const pattern = new RegExp(patternString);
const message = 'The number is 100';
console.log(pattern.exec(message)); // ['The number is 100', 'number', '100']
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
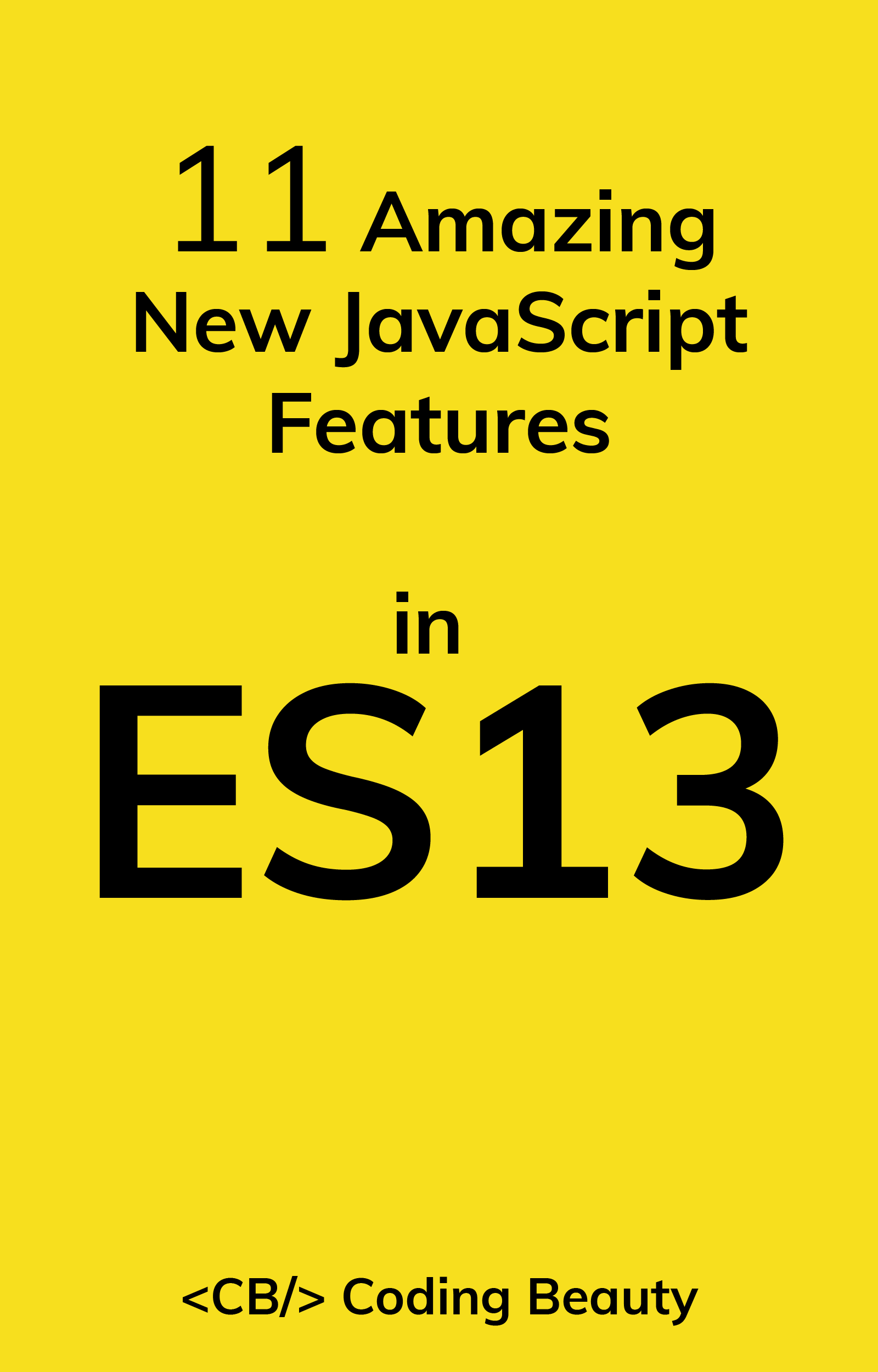
Sign up and receive a free copy immediately.