In this article, we’ll be looking at some ways to quickly get the last character of a string in JavaScript.
1. String at() Method
The get the last character of a string, we can call the at()
method on the string, passing -1
as an argument. For example, str.at(-1)
returns a new string containing the last character of str
.
const str = 'Coding Beauty';
const lastChar = str.at(-1);
console.log(lastChar); // y
The String
at()
method returns the character of a string at the specified index. When negative integers are passed to at()
, it counts back from the last string character.
2. String charAt() Method
Alternatively, to get the last character of a string, we can call the charAt()
method on the string, passing the last character index as an argument. For example, str.charAt(str.length - 1)
returns a new string containing the last character of str
.
const str = 'book';
const lastCh = str.charAt(str.length - 1);
console.log(lastCh); // k
The String
charAt()
method takes an index and returns the character of the string at that index.
Tip
In JavaScript, arrays use zero-based indexing. This means that the first character has an index of 0
, and the last character has an index of str.length - 1
.
Note
If we pass an index to charAt()
that doesn’t exist on the string, it returns an empty string (''
):
const str = 'book';
const lastCh = str.charAt(10);
console.log(lastCh); // ''
3. Bracket Notation ([]) Property Access
We can also use the bracket notation ([]
) to access the last character of a string. Just like with the charAt()
method we use str.length - 1
as an index to access the last character.
const str = 'book';
const lastCh = str[str.length - 1];
console.log(lastCh); // k
Note
Unlike with charAt()
, using the bracket notation to access a character at a non-existent index in the string will return undefined
:
const str = 'book';
const lastCh = str[10];
console.log(lastCh); // undefined
4. String split() and Array pop() Methods
With this method, we call the split()
method on the string to get an array of characters, then we call pop()
on this array to get the last character of the string.
const str = 'book';
const lastCh = str.split('').pop();
console.log(lastCh); // k
We passed an empty string (''
) to the split()
method to split the string into an array of all its characters.
const str = 'book';
console.log(str.split('')); // [ 'b', 'o', 'o', 'k' ]
The Array pop()
method removes the last element from an array and returns that element. We call it on the array of characters to get the last character.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
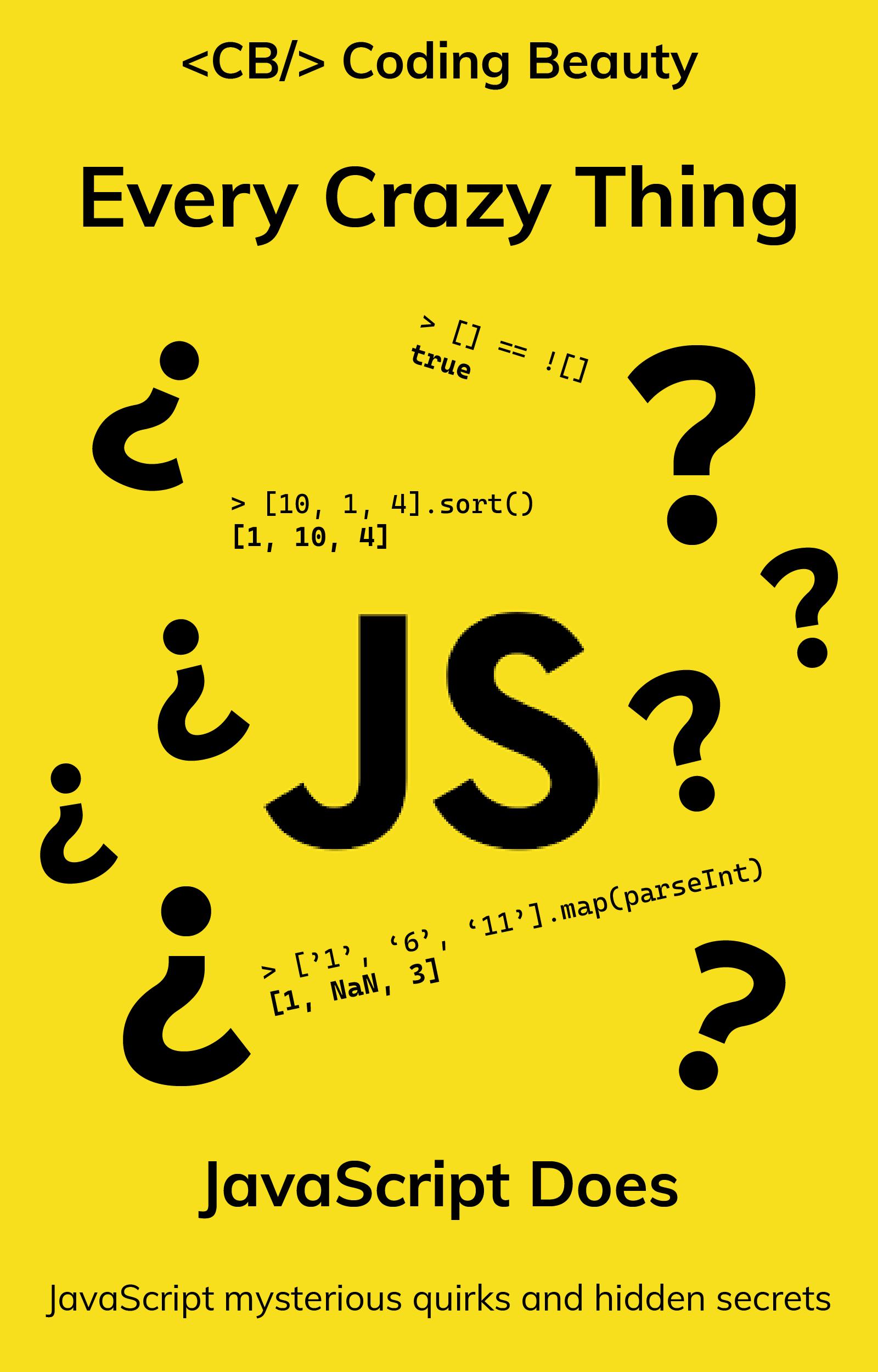