To get the intersection of two Sets in JavaScript, combine the filter()
and has()
array methods:
const set1 = new Set([1, 2, 3, 4, 5]);
const set2 = new Set([4, 5, 6, 7, 8]);
const intersection = new Set([...set1].filter(x => set2.has(x)));
console.log(intersection); // Set {4, 5}
The Array
filter()
method tests each element in an array against a condition specified by a callback function and creates a new array filled with elements that pass the test. It doesn’t modify the original array.
const arr = [1, 2, 3, 4];
const filtered = arr.filter((num) => num > 2);
console.log(filtered); // [ 3, 4 ]
The spread syntax (...
) converts the Set to an array:
const set1 = new Set([1, 2, 3, 4, 5]);
const arrayFromSet = [...set1];
console.log(arrayFromSet); // [1, 2, 3, 4, 5]
We can also do this with the Array
from()
method, which converts Sets and other iterables into arrays:
const set1 = new Set([1, 2, 3, 4, 5]);
const arrayFromSet = Array.from(set1);
console.log(arrayFromSet); // [1, 2, 3, 4, 5]
And the Set has()
method tells us whether or not the Set contains a particular element.
const set = new Set(['coding', 'beauty', 'dev']);
const hasElegance = set.has('elegance');
const hasBeauty = set.has('beauty');
console.log(hasElegance); // false
console.log(hasBeauty); // true
Get intersection of two sets with Array
forEach()
method
Alternatively, we can use the Array
forEach()
method to get the intersection of two Sets in JavaScript.
const set1 = new Set([1, 2, 3, 4, 5]);
const set2 = new Set([4, 5, 6, 7, 8]);
const intersection = new Set();
set1.forEach(value => {
if (set2.has(value)) {
intersection.add(value);
}
});
console.log(intersection); // Set {4, 5}
The Set
forEach loops through an array and calls a specified function on each element.
const fruits = new Set(['apple', 'orange', 'banana']);
fruits.forEach((fruit) => {
console.log(fruit.toUpperCase());
});
// Output 👇
// APPLE
// ORANGE
// BANANA
The Set
add()
method adds a new element to a Set:
const set = new Set([1, 2, 3]);
console.log(set); // Set(3) { 1, 2, 3 }
set.add(10);
console.log(set); // Set(4) { 1, 2, 3, 10 }
Get intersection of two Sets with for..of
loop
Anywhere you see the forEach()
, you can use the for..of
loop in its place:
const set1 = new Set([1, 2, 3, 4, 5]);
const set2 = new Set([4, 5, 6, 7, 8]);
const intersection = new Set();
for (const value of set1) {
if (set2.has(value)) {
intersection.add(value);
}
}
console.log(intersection); // Set {4, 5}
Get intersection of two Sets with reduce()
method
You can also use the reduce()
method to get the intersection of two Set
objects in JavaScript:
const set1 = new Set([1, 2, 3, 4, 5]);
const set2 = new Set([4, 5, 6, 7, 8]);
const intersection = [...set1].reduce((acc, value) => {
if (set2.has(value)) {
acc.add(value);
}
return acc;
}, new Set());
console.log(intersection); // Set {4, 5}
The reduce()
method calls a function on each element of an array to accumulate a resulting value:
const nums = [1, 2, 3, 4, 5];
const sum = nums.reduce((acc, num) => acc + num, 0);
const product = nums.reduce((acc, num) => acc * num, 1);
console.log(sum); // 15
console.log(product); // 120
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
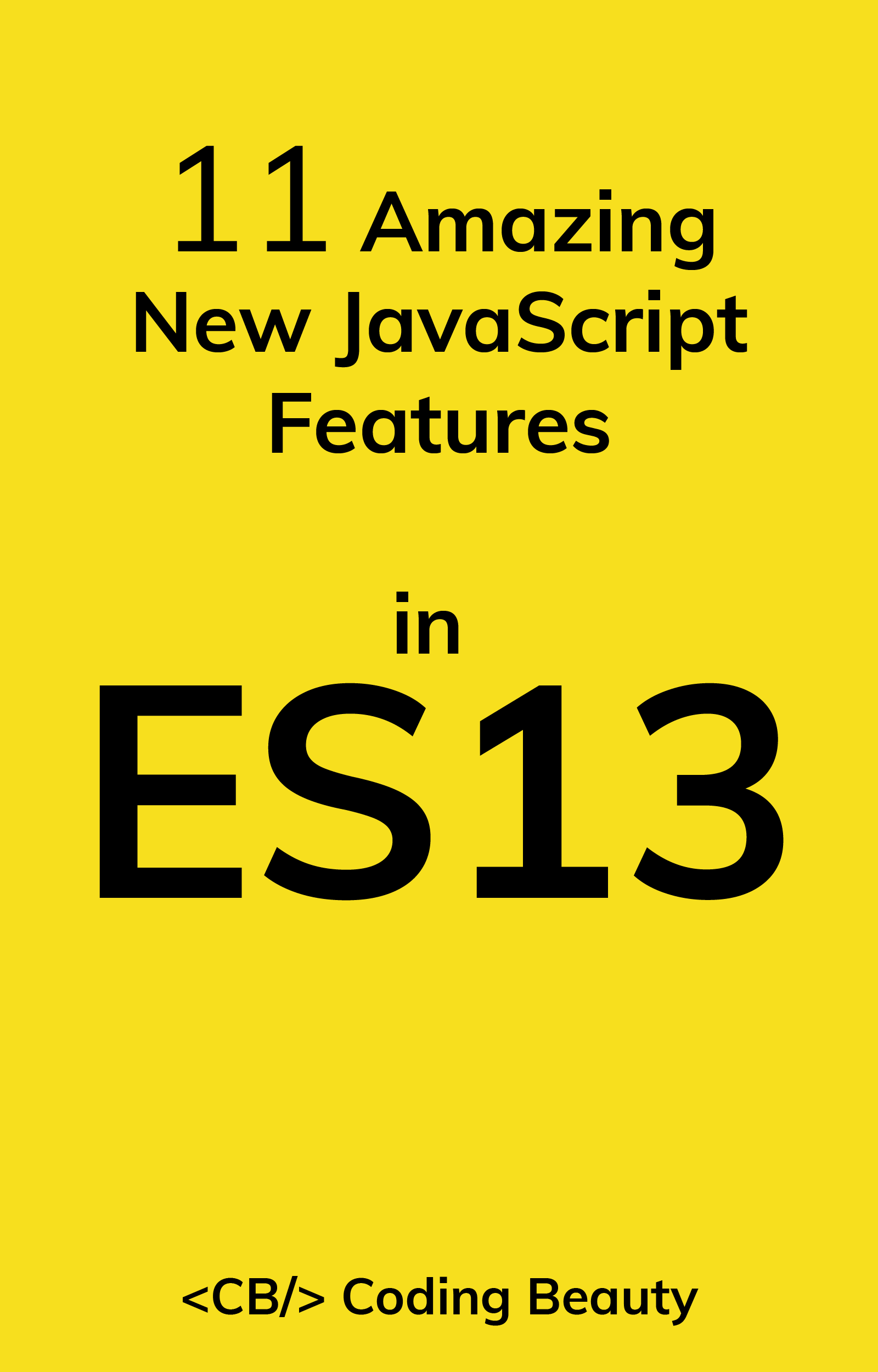