1. Array filter() Method
To find the even numbers in array with JavaScript, call the filter()
method on the array, passing a callback that returns true
when the element is even, and false
otherwise
const numbers = [7, 10, 15, 8, 13, 18, 6];
const evens = numbers.filter((num) => num % 2 === 0);
// [10, 8, 18, 6]
console.log(evens);
The filter()
method creates a new array with all the elements that pass the test specified in the testing callback function. Only even numbers have a remainder of 0
when divided by 2
, so filter()
returns an array of all the even numbers in the original array.
Note: filter()
preserves the order of the elements from the original array.
2. Array forEach() Method
Alternatively, we can find the odd numbers in an array with the Array
forEach()
method. We call forEach()
on the array, and in the callback, we only add an element to the resulting array if it is even.
const numbers = [7, 10, 15, 8, 13, 18, 6];
const evens = [];
numbers.forEach((num) => {
if (num % 2 === 0) {
evens.push(num);
}
});
// [ 10, 8, 18, 6 ]
console.log(evens);
3. for…of Loop
We can use the for...of
loop in place of forEach()
to iterate through the array and find the even numbers.
const numbers = [7, 10, 15, 8, 13, 18, 6];
const evens = [];
for (const num of numbers) {
if (num % 2 === 0) {
evens.push(num);
}
}
// [ 10, 8, 18, 6 ]
console.log(evens);
4. Traditional for Loop
And we can’t forget the traditional for
loop:
const numbers = [7, 10, 15, 8, 13, 18, 6];
const evens = [];
for (let i = 0; i < numbers.length; i++) {
const num = numbers[i];
if (num % 2 === 0) {
evens.push(num);
}
}
// [ 10, 8, 18, 6 ]
console.log(evens);
Performance Comparison
Let’s compare the performance of these four methods using an array with 10 million elements (with elements from 1 to 10 million).
const numbers = [...Array(10000000)].map(
(_, index) => index + 1
);
function measurePerf(label, method) {
console.time(label);
method();
console.timeEnd(label);
}
measurePerf('filter', () => {
const evens = numbers.filter((num) => num % 2 === 0);
});
measurePerf('forEach', () => {
const evens = [];
numbers.forEach((num) => {
if (num % 2 === 0) {
evens.push(num);
}
});
});
measurePerf('for...of', () => {
const evens = [];
for (const num of numbers) {
if (num % 2 === 0) {
evens.push(num);
}
}
});
measurePerf('for', () => {
const evens = [];
for (let i = 0; i < numbers.length; i++) {
const num = numbers[i];
if (num % 2 === 0) {
evens.push(num);
}
}
});
Results
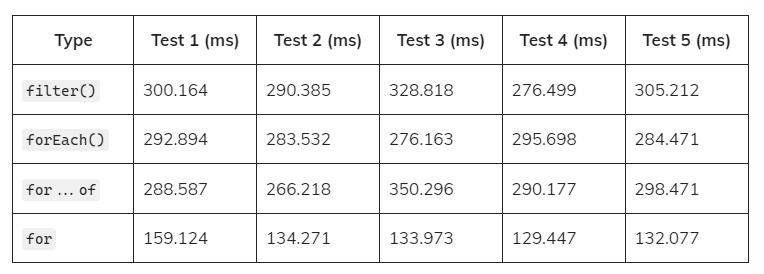
Average
filter()
: 300.216 ms
forEach()
: 286.516 ms
for...of
: 298.750 ms
for
: 137.778 ms
The traditional for
loop consistently comes out on top in this comparison. This doesn’t mean you must use it all the time, however. It’s not very often that you need to filter through an array with 10 million elements – in typical use cases, the performance gains you’d get from the for
loop will be minuscule. In most scenarios, you’d be better off using filter()
due to its greater conciseness and readability, and if you prefer the functional approach, despite its slower speed.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
