In this article, we’ll learn how to easily convert a string containing a comma-separated list of numbers to an array of these numbers in JavaScript.
String split() and Array map() Methods
To convert a string of numbers to an array, call the split()
method on the string, passing a comma (,
) as an argument, to get an array of the string representation of the numbers. Then use the map()
method to transform each string in the array into a number. For example:
const str = '1,2,3,4';
const nums = str.split(',').map(Number);
console.log(nums); // [ 1, 2, 3, 4 ]
The String
split()
method divides a string into an array of characters using the specified separator. We pass a comma to separate the stringified numbers into separate elements in the resulting array.
console.log('1,2,3,4'.split(',')); // [ '1', '2', '3', '4' ]
The Array
map()
method creates a new array from the result of calling a callback function on each element of the original array. We pass the Number
constructor to map()
, so Number()
will be called on each string in the array to convert it to a number.
We can use the map()
method more explicitly like this:
const str = '1,2,3,4';
const nums = str.split(',').map((item) => Number(item));
console.log(nums); // [ 1, 2, 3, 4 ]
You might prefer this approach since it lets you see exactly what arguments you’re passing to the callback function. This can prevent bugs in situations where the callback function behaves differently depending on the number of arguments passed.
Apart from the Number
constructor, we can also convert each item in the string array to a number with parseInt()
:
const str = '1,2,3,4';
const nums = str.split(',').map((item) => parseInt(item, 10));
console.log(nums); // [ 1, 2, 3, 4 ]
parseInt()
parses a string and returns an integer of the specified base. We pass 10
as the second argument, so parseInt()
uses base 10 to parse the string.
We can also use the unary operator (+
) in place of parseInt()
or Number()
. This operator converts a non-numeric value into a number.
const str = '1,2,3,4';
const nums = str.split(',').map((item) => +item);
console.log(nums); // [ 1, 2, 3, 4 ]
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
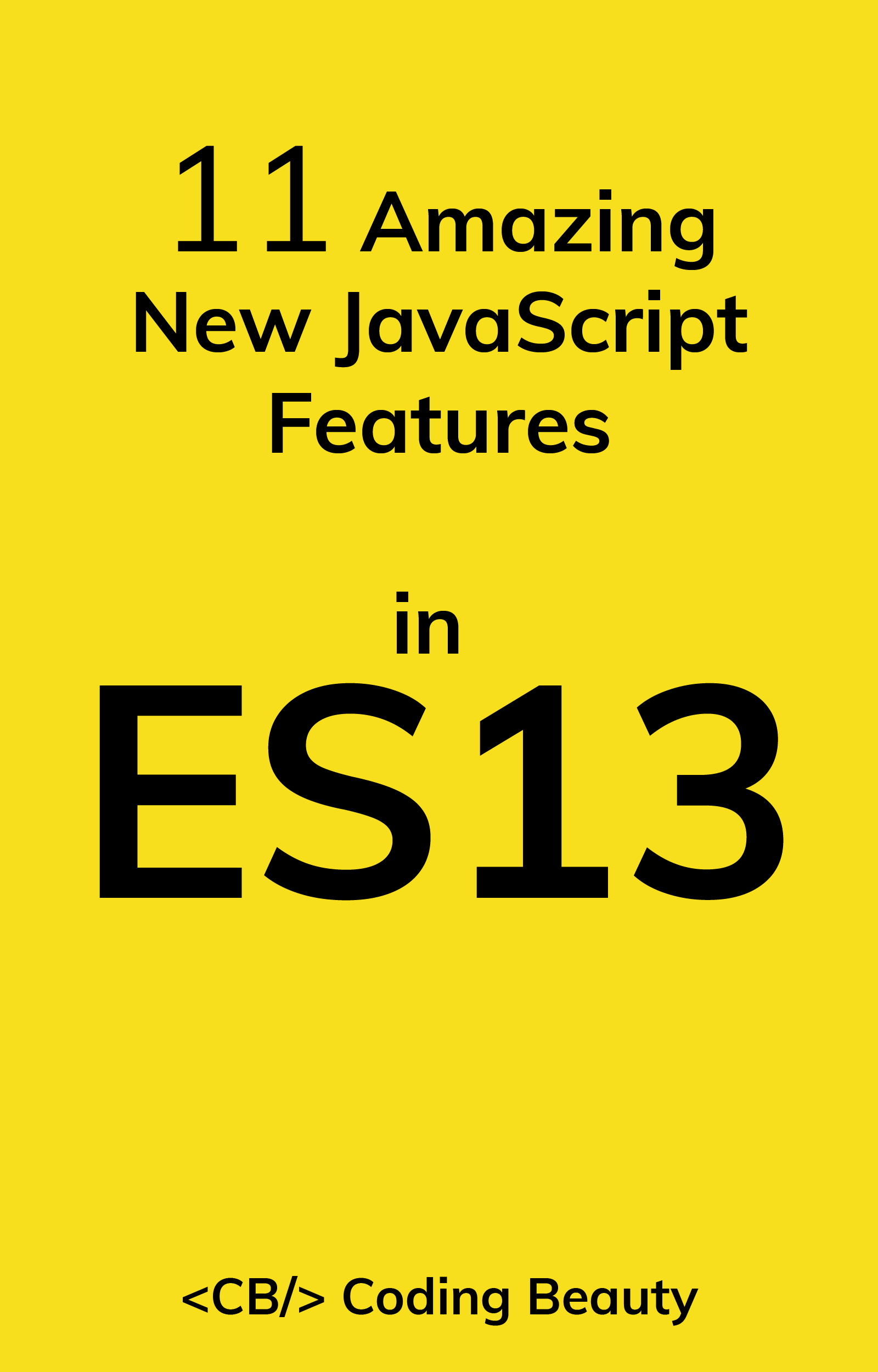