Let’s look at some ways to easily convert the values of a Map
object to an array in JavaScript.
1. Map values() and Array from()
To convert Map
values to an array, we can call the values()
method on the Map
, and pass the result to the Array.from()
method. For example:
const map = new Map([
['user1', 'John'],
['user2', 'Kate'],
['user3', 'Peter'],
]);
const values = Array.from(map.values());
console.log(values); // [ 'John', 'Kate', 'Peter' ]
The Map
values()
method returns an iterable of values in the Map
. The Array.from()
method can create arrays from iterables like this.
2. Map values() and Spread Syntax (…)
We can also use the spread syntax (...
) to unpack the elements of the iterable returned by the Map
values()
method into an array. For example:
const map = new Map([
['user1', 'John'],
['user2', 'Kate'],
['user3', 'Peter'],
]);
const values = [...map.values()];
console.log(values); // [ 'John', 'Kate', 'Peter' ]
Using the spread syntax allows us to combine the values of multiple Map
objects into one array. For example:
const map1 = new Map([['user1', 'John']]);
const map2 = new Map([
['user2', 'Kate'],
['user3', 'Peter'],
]);
const values = [...map1.values(), ...map2.values()];
console.log(values); // [ 'John', 'Kate', 'Peter' ]
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
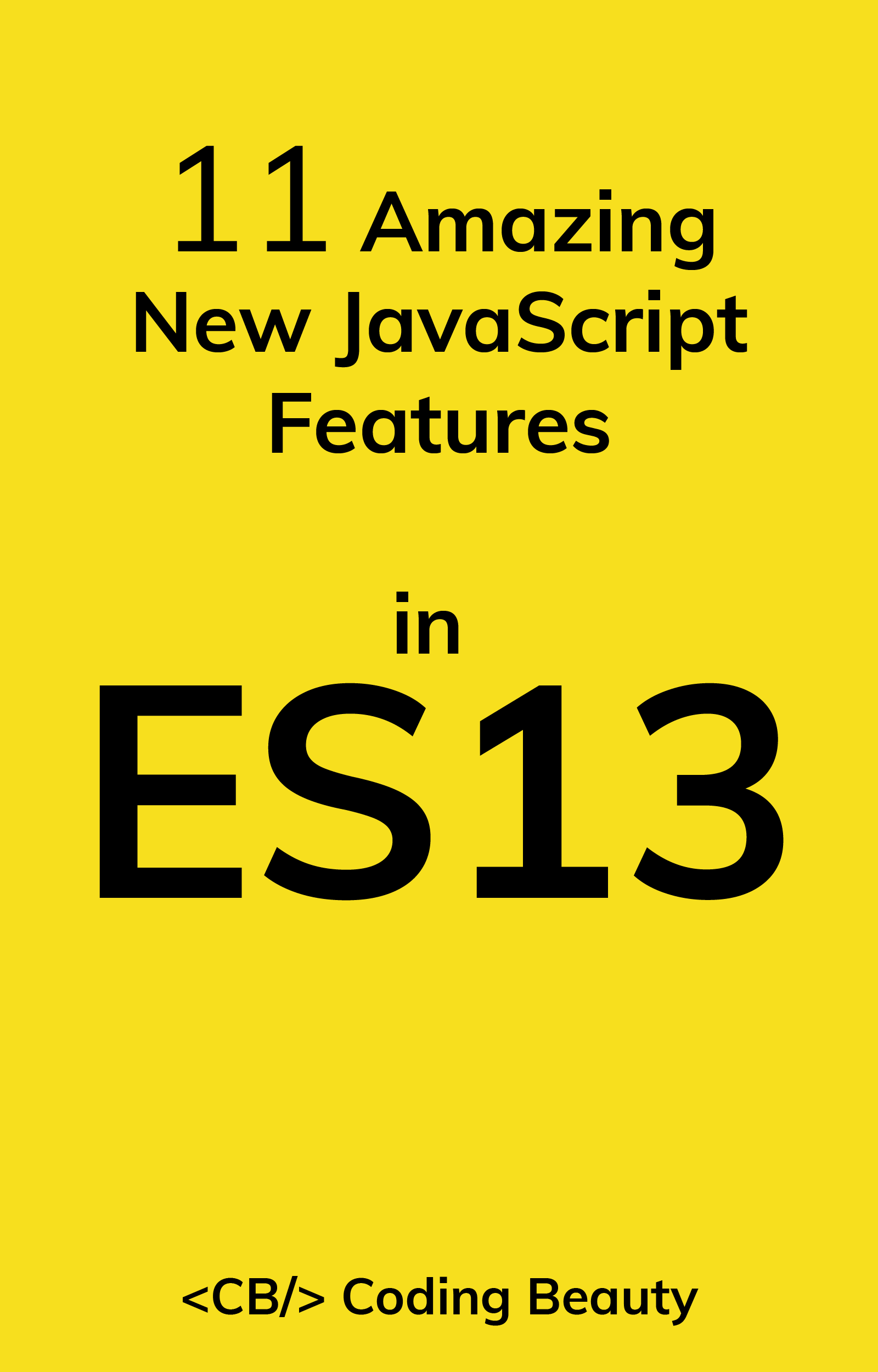