With the jspdf
library, we can easily convert any HTML page or form to a PDF:
For example:
import { jsPDF } from 'jspdf';
const pdfContentEl = document.getElementById('pdf-content');
const doc = new jsPDF();
await doc.html(pdfContentEl.innerHTML).save('test.pdf');
PDF is a popular file format we use to present and share documents with a fixed layout across different platforms and devices.
To start the conversion, we create a new jsPDF
object with the constructor. Then we call the html()
method, passing the element with the contents we want to be in the PDF. On the result, we call save()
, passing our desired name of the output PDF file.
Let’s say we had HTML like this:
<div id="pdf-content">
<h1>Test</h1>
<p>Here's what we're saving to PDF</p>
</div>
<button id="save-pdf">Save PDF</button>
with an output on the webpage like this:
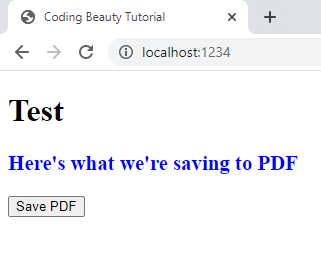
When we click the “Save PDF” button, jsPDF will create a new PDF from the HTML element and download it as a file in the browser
Here’s what displays when we open the PDF:
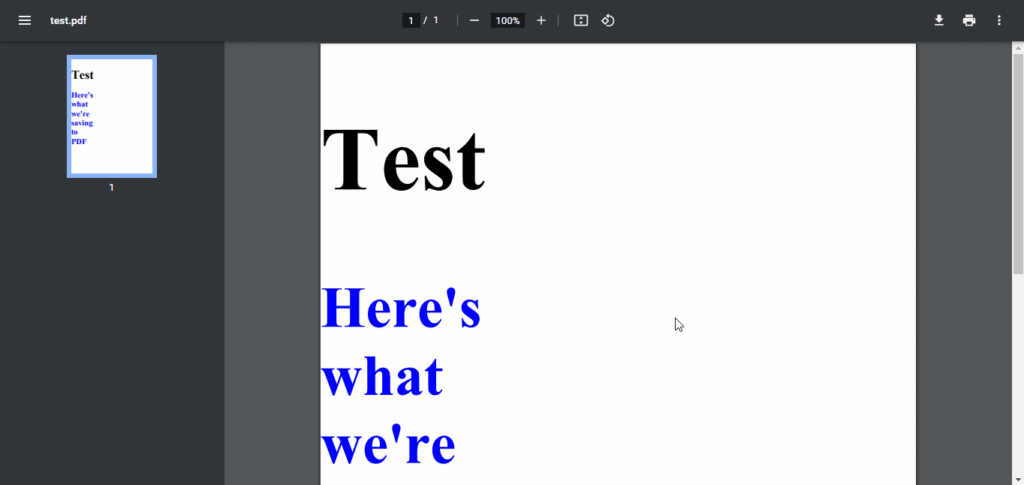
Install jsPDF
To get started with the jsPDF library, we can install it from NPM with this command.
npm i jspdf
After the installation, we’ll be able to import it into a JavaScript file, like this:
import { jsPDF } from 'jspdf';
For this file to work in the HTML, we can use a module bundler like Parcel, which is what I use.
With Parcel, we can include the script in the HTML like this:
<script type="module" src="index.js"></script>
We can use modern tools like TypeScript and ES module imports in the script, and it will work just fine because of Parcel.
As far as we run the HTML with npx parcel my-file.html
after installing Parcel with npm install parcel
.
npm install parcel
npx parcel my-file.html
Parcel makes the HTML available at localhost:1234
, as you might have seen in the demo above.
Customize HTML to PDF conversion
The jsPDF
constructor accepts an options object that customizes the PDF conversion process.
For example, the orientation
option sets the orientation of the resulting PDF.
By default, it’s portrait
, but we can set it to landscape
.
Customize PDF orientation
const doc = new jsPDF({ orientation: 'landscape' });
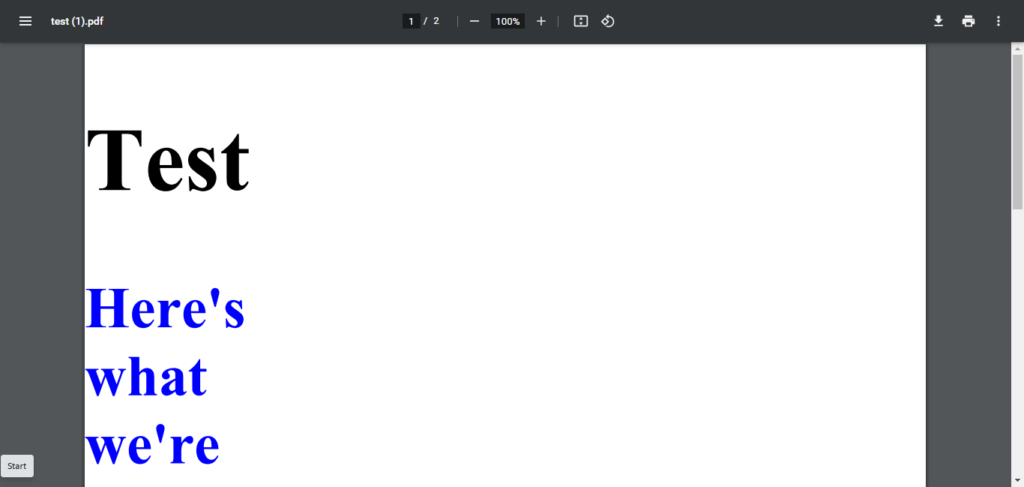
Customize PDF unit and dimensions
With the unit
and format
options, we can set the unit and dimensions of each PDF page in the output file.
const doc = new jsPDF({ orientation: 'l', unit: 'in', format: [4, 2] });
Here we specify a landscape export that is 2 by 4 inches.
Convert HTML form to PDF
jsPDF also works with HTML elements whose appearance can change dynamically from user interaction, like form inputs.
<form id="form">
<input type="email" name="email" id="email" placeholder="Email" />
<br />
<input
type="password"
name="password"
id="password"
placeholder="Password"
/>
<br /><br />
<button type="submit">Submit</button>
</form>
<br />
<button id="save-pdf">Save PDF</button>
import { jsPDF } from 'jspdf';
const doc = new jsPDF();
const savePdf = document.getElementById('save-pdf');
const formEl = document.getElementById('form');
savePdf.addEventListener('click', async () => {
await doc.html(formEl).save('test.pdf');
});
In the webpage, we’ve put in some test values in the two form inputs, to see that they display in the PDF output.
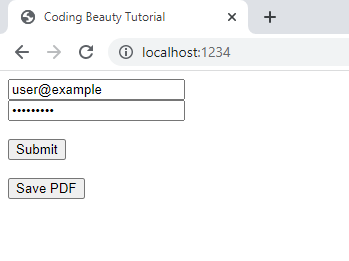
The PDF:
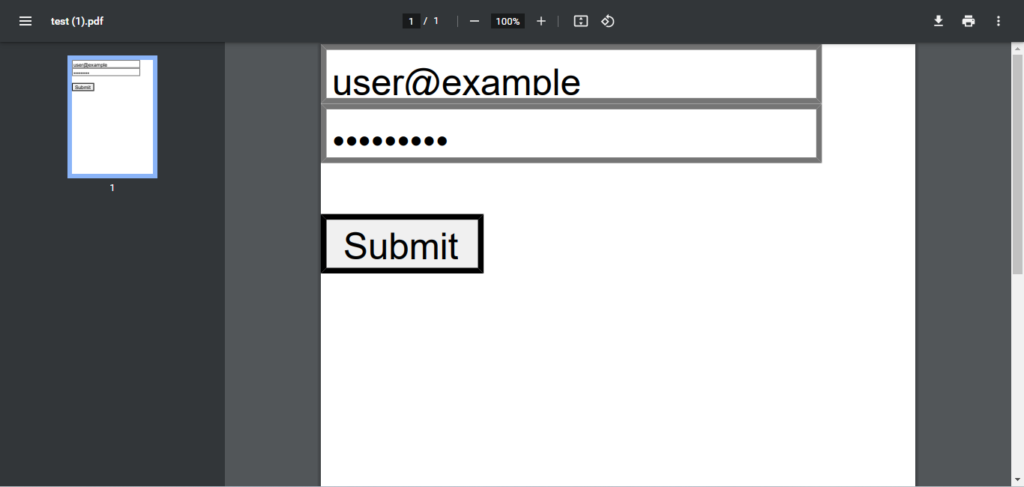
We can’t interact with the form inputs or button in the PDF file though.
Key takeaways
The jsPDF library provides a convenient way to convert HTML content, including forms, to PDF format. The entire process is pretty easy, as we can create a new jsPDF
object, call the html()
method to specify the content, and then use the save()
method to generate the output file. Plus, we can customize the PDF output with options like orientation
, unit
, and format
. Overall, using jsPDF simplifies creating PDF files from HTML content in our web apps.
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
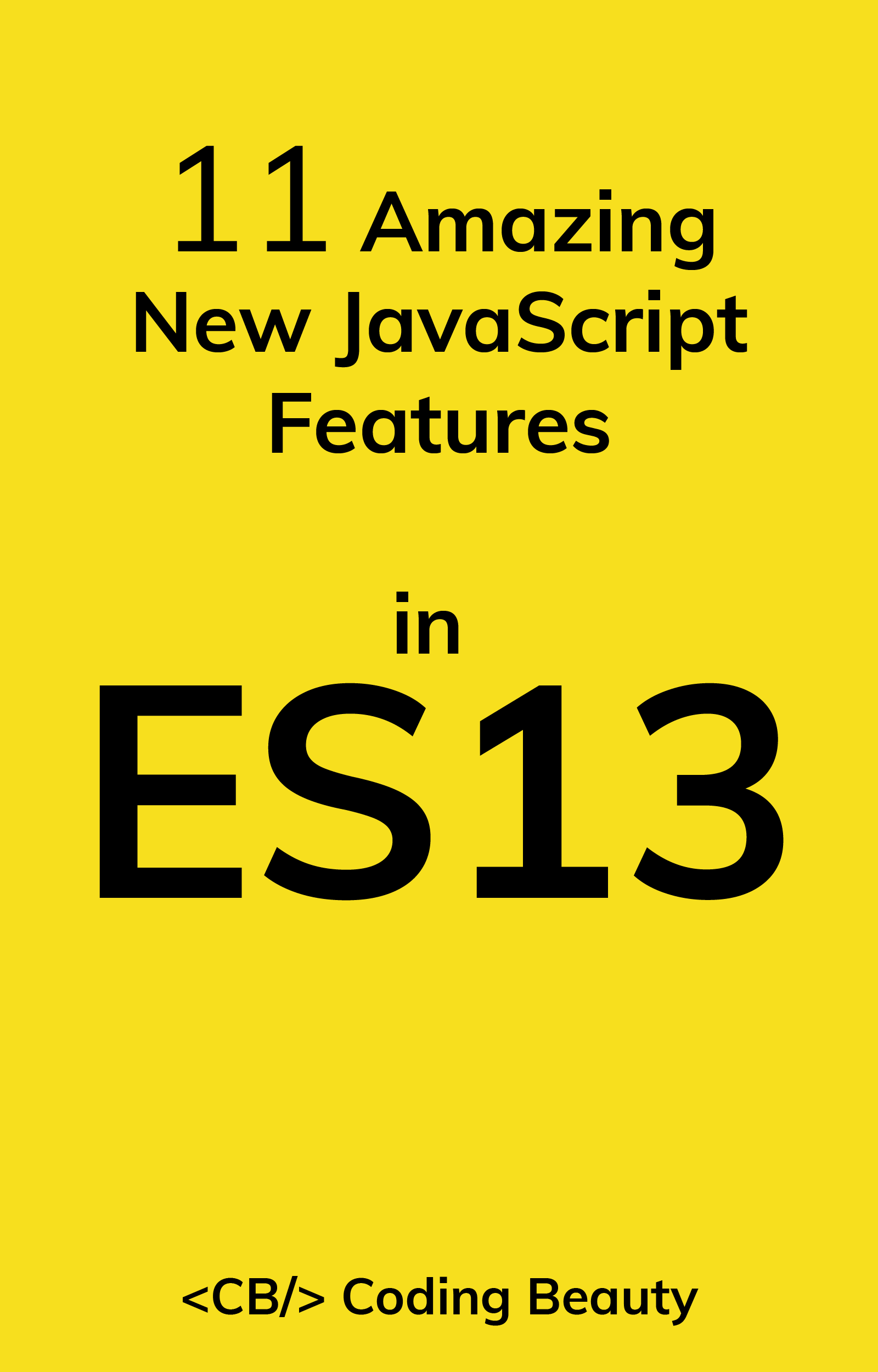