In this article, we’ll be looking at two ways to quickly convert an array of objects to a Map
object in JavaScript.
1. Map() Constructor and Array map()
To convert an array of objects to a map, we can use the Array
map()
method to create an array of key-value pairs, and then pass the resulting array to a Map()
constructor to create a Map
object.
const arr = [
{ key: 'user1', value: 'John' },
{ key: 'user2', value: 'Kate' },
{ key: 'user3', value: 'Peter' },
];
const map = new Map(arr.map((obj) => [obj.key, obj.value]));
// Map(3) { 'user1' => 'John', 'user2' => 'Kate', 'user3' => 'Peter' }
console.log(map);
In the callback passed to the map()
method, we return an array containing the key and the value for each object. This will result in an array of key-value pairs:
// [ [ 'user1', 'John' ], [ 'user2', 'Kate' ], [ 'user3', 'Peter' ] ]
console.log(arr.map((obj) => [obj.key, obj.value]));
The Map
constructor can take an array of this form as an argument to create a Map
object.
2. Map set() and Array forEach()
Another way to convert an array of objects to a map is with the Array
forEach()
method. First, we create a new Map
object. Then, we add entries for all the array elements to the Map
, by calling the Map
set()
method in the callback passed to forEach()
. Here’s an example:
const arr = [
{ key: 'user1', value: 'John' },
{ key: 'user2', value: 'Kate' },
{ key: 'user3', value: 'Peter' },
];
const map = new Map();
arr.forEach((obj) => {
map.set(obj.key, obj.value);
});
// Map(3) { 'user1' => 'John', 'user2' => 'Kate', 'user3' => 'Peter' }
console.log(map);
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
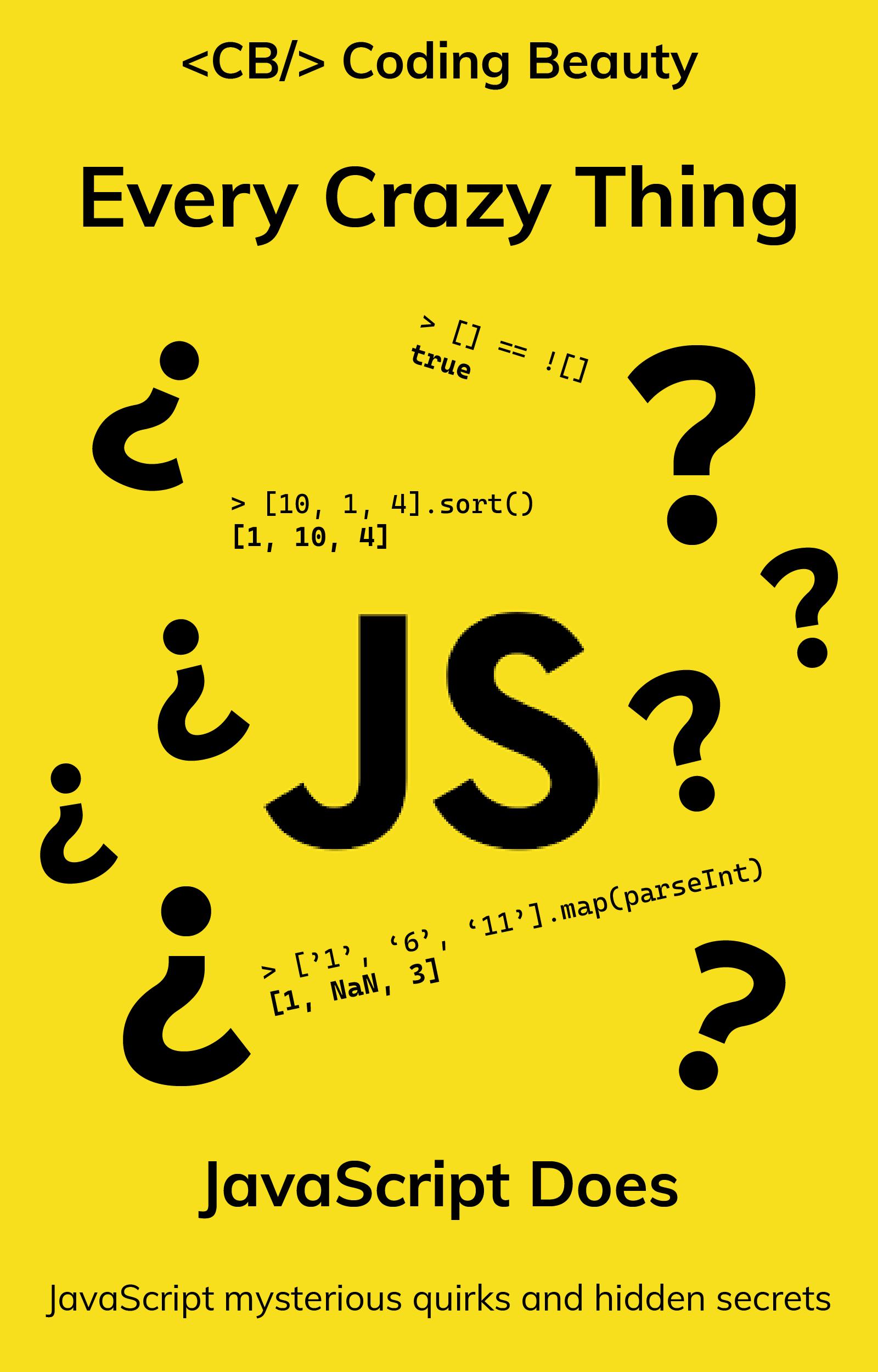