In this article, we’ll be learning how to concatenate a bunch of strings in JavaScript with a separator of our choice, like a comma or hyphen.
The Array join() Method
To concatenate strings with a separator, we can create an array containing the strings and call the join()
method, passing the separator as an argument. The join()
method will return a string containing the array elements joined with the separator. For example:
const str1 = 'html';
const str2 = 'css';
const str3 = 'javascript';
const spaceSeparated = [str1, str2, str3].join(' ');
console.log(spaceSeparated); // html css javascript
const commaSeparated = [str1, str2, str3].join(',');
console.log(commaSeparated); // html,css,javascript
Multi-Character Separator
The separator doesn’t have to be a single character, we can pass strings with multiple characters, like words:
const str1 = 'html';
const str2 = 'css';
const str3 = 'javascript';
const andSeparated = [str1, str2, str3].join(' and ');
console.log(andSeparated); // html and css and javascript
If we call the join()
method on the string array without passing arguments, the strings will be separated with a comma:
const str1 = 'html';
const str2 = 'css';
const str3 = 'javascript';
const withoutSeparator = [str1, str2, str3].join();
console.log(withoutSeparator); // html,css,javascript
While this works, it’s better to be explicit by passing the comma as an argument if you need comma separation, as not everyone is aware of the default behaviour.
Note: The join()
method will return an empty string if we call it on an empty array. For example:
console.log([].join(',')); // ''
Note: Elements like undefined
, null
, or empty array values get converted to an empty string during the join:
const joined = [null, 'html', undefined, 'css', []].join();
console.log(joined); // ,html,,css,
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
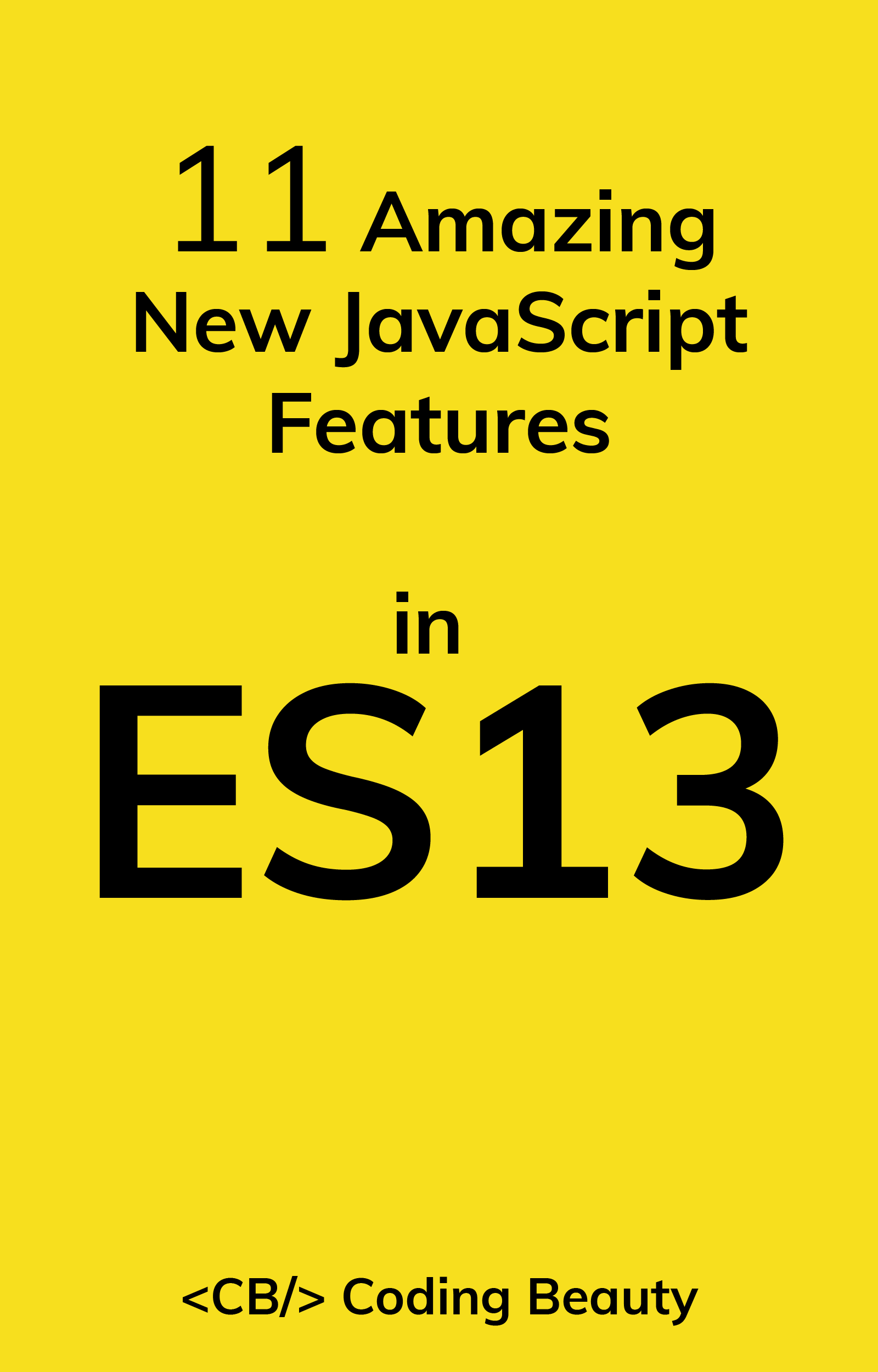