Are you currently experiencing the “Cannot use import statement outside a module” error in JavaScript?

This is a well-known error that happens when you use the import
keyword to load a file or package that is not a module.
Let’s learn how to easily fix this error in Node.js or the browser.
Fix “Cannot use import statement outside a module” in browser
Fix: Set type="module"
in <script>
tag
The “Cannot use import statement outside a module” error happens in a browser when you import a file that is a module without indicating this in the <script>
tag.
<!DOCTYPE html>
<html>
<head>
<title>Coding Beauty</title>
<link rel="icon" href="favicon.ico" />
</head>
<body>
A site to make you enjoy coding
<!-- ❌ SyntaxError: Cannot use import statement outside a module -->
<script src="index.js"></script>
</body>
</html>
To fix it, add type="module"
to the <script>
tag.
<!DOCTYPE html>
<html>
<head>
<title>Coding Beauty</title>
<link rel="icon" href="favicon.ico" />
</head>
<body>
A site to make you enjoy coding
<!-- ✅ Loads script successfully -->
<script type="module" src="index.js"></script>
</body>
</html>
Fix “Cannot use import statement outside a module” in Node.js
The “Cannot use import statement outside a module” error happens when you use the import
keyword in a Node.js project that doesn’t use the ES module import format.
// ❌ SyntaxError: Cannot use import statement outside a module
import axios from 'axios';
const { data } = (
await axios.post('https://api.example.com/hi', {
hello: 'world',
})
).data;
console.log(data);
Fix: Set type: "module"
in package.json
To fix the error in Node.js, set the package.json
type
field to “module”.
{
// ...
"type": "module",
// ...
}
Now you can use the import
statement with no errors:
// ✅ Imports successfully
import axios from 'axios';
const { data } = (
await axios.post('https://api.example.com/hi', {
hello: 'world',
})
).data;
console.log(data);
If there’s no package.json
in your project, you can initialize one with the npm init -y
command.
npm init -y
The -y
flags creates the package.json
with all the default options – no user prompts for the fields.
Fix: Use require
instead of import
Alternatively, you can fix the “Cannot use import statement outside a module” error in JavaScript by using the require()
function in place of the import
syntax:
// ✅ Works - require instead of import
const axios = require('axios');
const { data } = (
await axios.post('https://api.example.com/hi', {
hello: 'world',
})
).data;
console.log(data);
But require()
only works on the older, Node-specific CommonJS module system, and these days more and more modules are dropping support for CommonJS in favor of ES6+ modules.
For example, if you use require()
for modules like got
, node-fetch
, and chalk
, you’ll get the “require of ES modules is not supported error”.
// ❌ [ERR_REQUIRE_ESM]: require() of ES Module not supported
const chalk = require('chalk';
console.log(chalk.blue('Coding Beauty'));
If you can’t switch to an ES module environment, you can fix this by dynamically importing the module with the async
import()
function. For example:
// CommonJS module
// ✅ Imports successfully
const chalk = (await import('chalk')).default;
console.log(chalk.blue('Coding Beauty'));
Or, you can downgrade to an earlier version of the package that supported CommonJS and require()
.
npm install chalk@4
# Yarn
yarn add got@4
Here are the versions you should install for various well-known NPM packages to avoid this ERR_REQUIRE_ESM error:
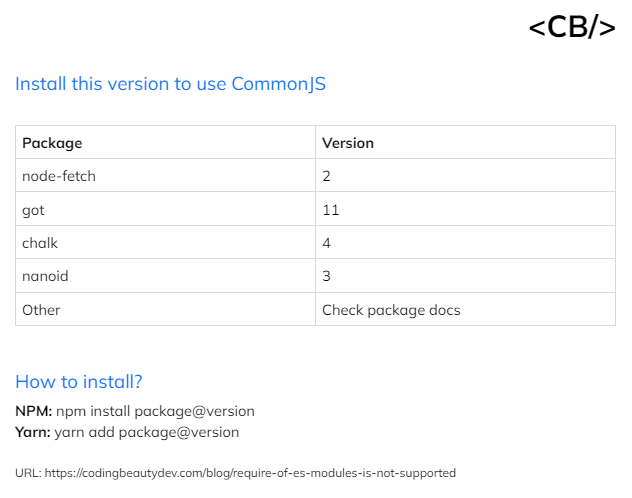
Fix “Cannot use import statement outside a module” in TypeScript
If the module
field in tsconfig.json
is set to commonjs
, any import
statement in the TypeScript source will change to require()
calls after the compilation and potentially cause this error.
{
//...
"type": "module"
}
Prevent this by setting module
to esnext
or nodenext
{
"type": "module"
}
nodenext
indicates to the TypeScript compiler that the project can emit files in either ESM or CommonJS format.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
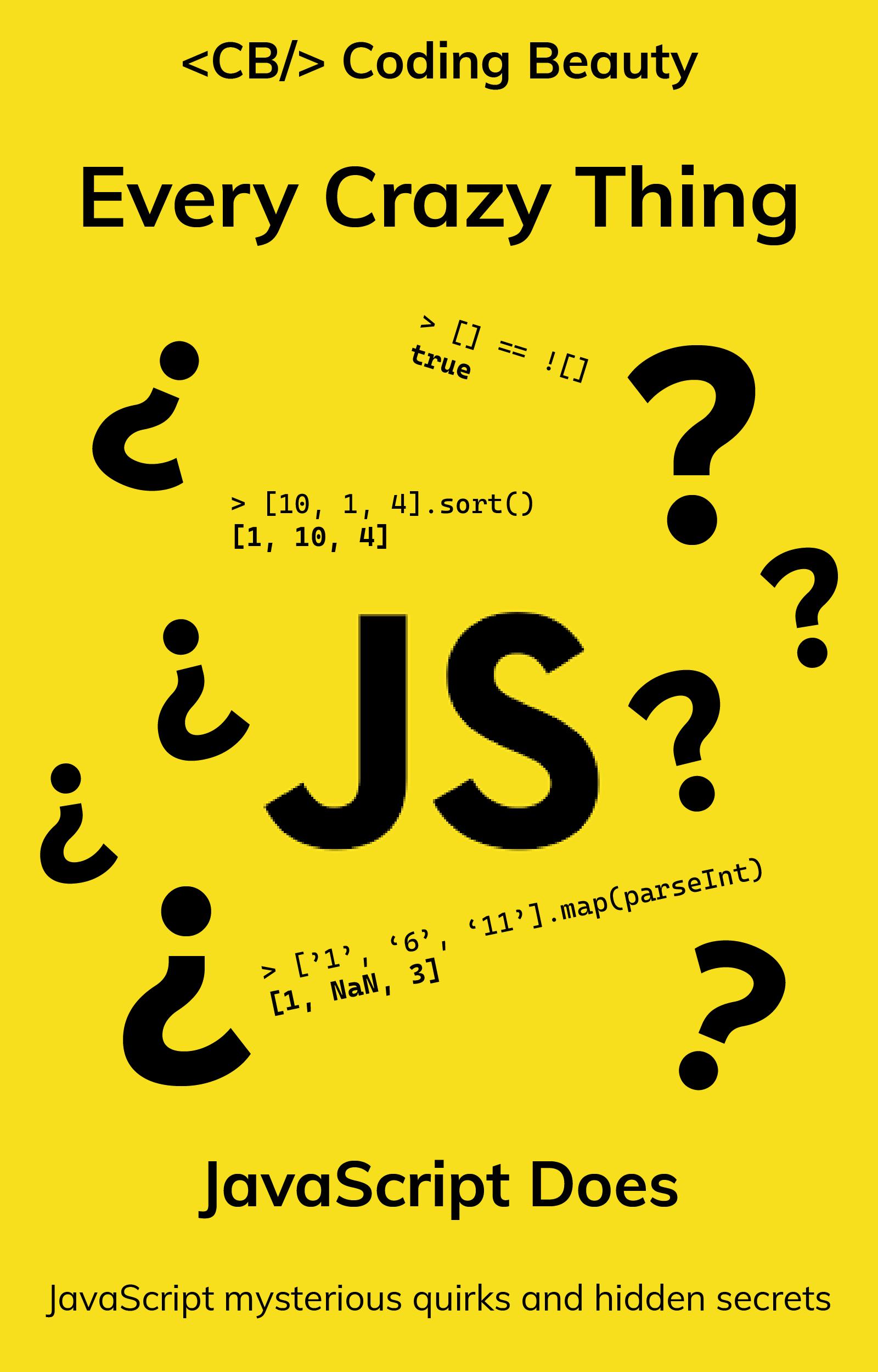