The “Cannot read property ‘then’ of undefined” error occurs in JavaScript when you call try then()
on a value – typically a function’s return value – but this value is undefined
.
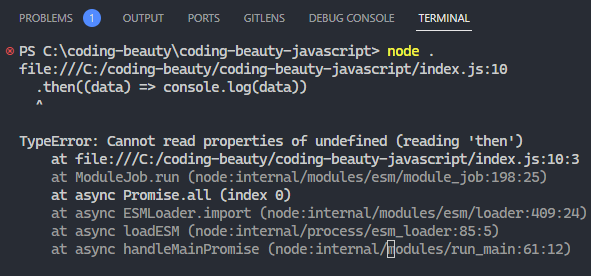
This could happen for one of the following reasons:
- Not returning the Promise in the function with
return
. - Not returning a value in an
async
function. - Not chaining Promises properly.
To fix it, ensure the function returns actually returns a Promise
.
Now let’s look at some specific cases of the error and learn how to fix it in a few easy steps.
Fix: Promise not returned
The “Cannot read property ‘then’ of undefined” happens when you forget to use the return
keyword return the Promise
from the function.
function fetchData(apiUrl) {
// 👇 `return` keyword missing
fetch(apiUrl).then((response) => {
return response.json();
});
}
// ❌ Cannot read property 'then' of undefined
fetchData('/api/data')
.then((data) => console.log(data))
To fix it, simply return the Promise
with return
:
function fetchData(apiUrl) {
// we return the Promise
return fetch(apiUrl).then((response) => {
return response.json();
});
}
// ✅ Runs successfully
fetchData('/api/data')
.then((data) => console.log(data))
Fix: Asynchronous function doesn’t return a value
The “Cannot read property ‘then’ of undefined” error happens in JavaScript when an async
function doesn’t return a value, for example, due to an oversight on our part when writing conditional control flow.
async function getUserData(userId) {
if (userId) {
const response = await fetch(`/api/users/${userId}`);
return response.json();
}
// 😕 No return if userId is absent
}
// ❌ Cannot read property 'then' of undefined if userId is absent
getUserData().then(data => console.log(data));
To fix it, check all flow paths and make sure the async
function always returns a value.
async function getUserData(userId) {
if (userId) {
const response = await fetch(`/api/users/${userId}`);
return response.json();
}
// 👍 Return a resolved Promise even if userId is absent
return Promise.resolve(null);
}
// ✅ Now, we can safely use 'then'
getUserData().then(data => console.log(data));
Fix: Promise
is not properly chained
The “Cannot read property ‘then’ of undefined” error occurs in JavaScript when you don’t chain the Promise
s properly:
function fetchAndParseUser(apiUrl) {
fetch(apiUrl)
.then((response) => {
console.log(response);
// 😕 Forgot to return the 'json' Promise
});
}
// ❌ Error: Cannot read property 'then' of undefined
fetchAndParseUser('/api/user')
.then(data => console.log(data))
To fix it in this case, make sure that each then
in the chain returns a Promise
if we want to continue the chain.
function fetchAndParseUser(apiUrl) {
// 👇 Here, we return the 'json' Promise
return fetch(apiUrl)
.then((response) => {
console.log(response);
return response.json(); // 👍 Return the Promise here
});
}
// ✅ Now, we can safely use 'then'
fetchAndParseUser('/api/user')
.then(data => console.log(data))
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
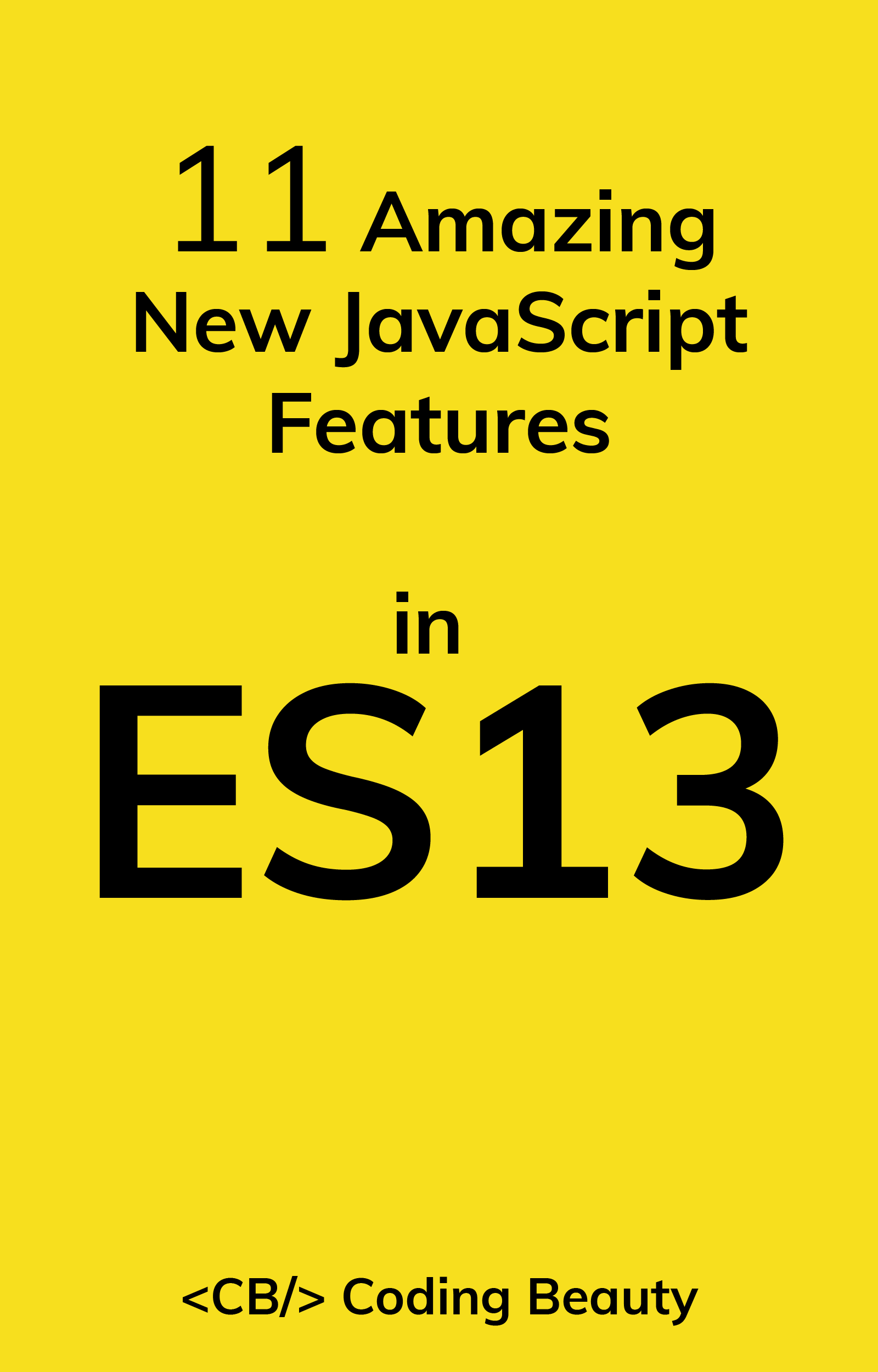