Let’s look at some ways to easily add 1 year to a Date
object in JavaScript.
1. Date setFullYear() and getFullYear() Methods
To add 1 year to a date, call the getFullYear(
) method on the date to get the year, then call the setFullYear()
method on the date, passing the sum of getFullYear()
and 1
as an argument, i.e., date.setFullYear(date.getFullYear() + 1)
.
For example:
function addOneYear(date) {
date.setFullYear(date.getFullYear() + 1);
return date;
}
// April 20, 2022
const date = new Date('2022-04-20T00:00:00.000Z');
const newDate = addOneYear(date);
// April 20, 2023
console.log(newDate); // 2023-04-20T00:00:00.000Z
Our addOneYear()
function takes a Date
object and returns the same Date
with the year increased by 1.
The Date
getFullYear()
method returns a number that represents the year of a particular date.
The Date
setFullYear()
method sets the year of a date to a specified number.
Avoiding Side Effects
The setFullYear()
method mutates the Date
object it is called on. This introduces a side effect into our addOneYear()
function. To avoid modifying the passed Date
and create a pure function, make a copy of the Date
and call setFullYear()
on this copy, instead of the original.
function addOneYear(date) {
// Making a copy with the Date() constructor
const dateCopy = new Date(date);
dateCopy.setFullYear(dateCopy.getFullYear() + 1);
return dateCopy;
}
// April 20, 2022
const date = new Date('2022-04-20T00:00:00.000Z');
const newDate = addOneYear(date);
// April 20, 2023
console.log(newDate); // 2023-04-20T00:00:00.000Z
// Original not modified
console.log(date); // 2022-04-20T00:00:00.000Z
Functions that don’t modify external state (i.e., pure functions) tend to be more predictable and easier to reason about. This makes it a good practice to limit the number of side effects in your programs.
2. date-fns addYears() Function
Alternatively, we can use the pure addYears()
function from the date-fns NPM package to quickly add 1 year to a date.
import { addYears } from 'date-fns';
// April 20, 2022
const date = new Date('2022-04-20T00:00:00.000Z');
const newDate = addYears(date, 1);
// April 20, 2023
console.log(newDate); // 2023-04-20T00:00:00.000Z
// Original not modified
console.log(date); // 2022-04-20T00:00:00.000Z
This function takes a Date
object and the number of years to add as arguments, and returns a new Date
object with the newly added years. It does not modify the original date passed to it.
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
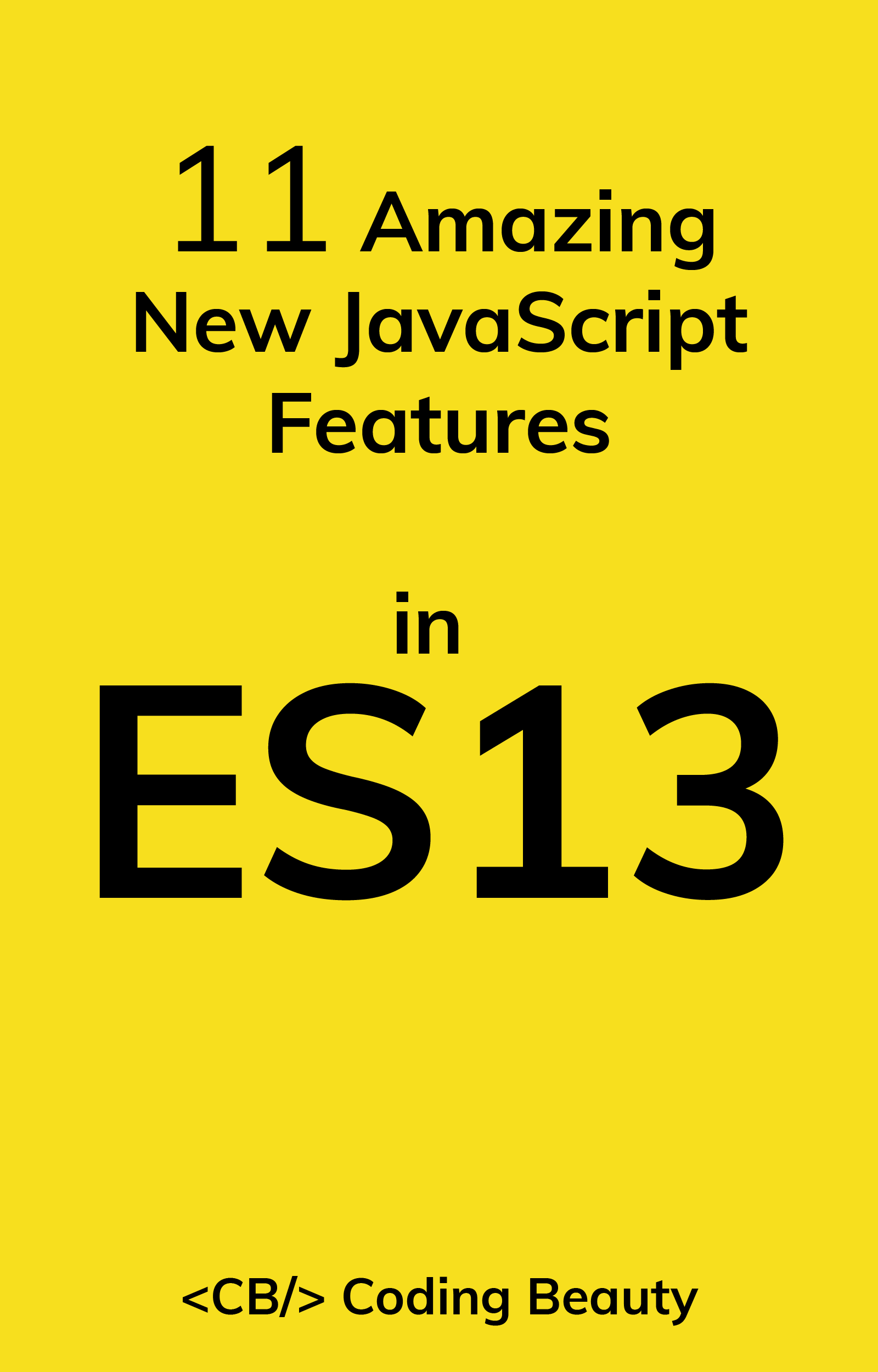