How to Capitalize the First Letter of a String in Vue.js
To capitalize the first letter of a string in Vue:
- Get the string’s first letter with
[0]
. - Uppercase this letter with
.toUpperCase()
. - Get the rest of the string with
.slice(1)
. - Add the results together.
For example:
App.vue
<template>
<div id="app">
<b>{{ name1 }}</b>
<br />
Capitalized: <b>{{ capitalized(name1) }}</b>
<br /><br />
<b>{{ name2 }}</b
>
<br />
Capitalized:
<b>{{ capitalized(name2) }}</b>
</div>
</template>
<script>
export default {
data() {
return { name1: 'coding beauty', name2: 'javascript' };
},
methods: {
capitalized(name) {
const capitalizedFirst = name[0].toUpperCase();
const rest = name.slice(1);
return capitalizedFirst + rest;
},
},
};
</script>
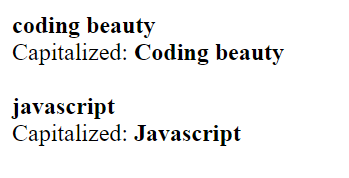
We create a reusable Vue instance method (capitalized
) that takes a string and capitalizes its first letter.
We use bracket indexing ([ ]
) to get the 0
property of the string – the character at index 0
. String (and array) indexing is zero-based in JavaScript, so the string’s first character is at index 0
, the second is at index 1
, and so on, until the last character at index str.length-1
.
After getting the string’s first character, we uppercase it with the toUpperCase()
method.
We use the slice()
method to get the rest of the string. slice()
returns the portion of a string between a specified start and an optional end index. If the end index is omitted, the substring will range from the start index to the end of the string.
Hence, slice(1)
returns a substring ranging from the second character to the end.
After getting the remaining part of the string, all that’s left is simply concatenating it with the capitalized first letter.
If it’s necessary for the resulting string to have only its first letter capitalized, you can call the toLowerCase()
method on the result of slice()
, to lowercase the rest of the string before concatenation.
const capitalizedFirst = name[0].toUpperCase();
// ๐ toLowerCase()
const rest = name.slice(1).toLowerCase();
return capitalizedFirst + rest;
Use Computed Property
If you’re trying to capitalize a string data property of the Vue instance (like in our example), you can use a computed property in place of a method.
<template>
<div id="app">
<b>{{ name1 }}</b>
<br />
Capitalized: <b>{{ capitalizedName1 }}</b> <br /><br />
<b>{{ name2 }}</b
><br />
Capitalized:
<b>{{ capitalizedName2 }}</b>
</div>
</template>
<script>
export default {
data() {
return { name1: 'coding beauty', name2: 'javascript' };
},
methods: {
capitalized(name) {
const capitalizedFirst = name[0].toUpperCase();
const rest = name.slice(1);
return capitalizedFirst + rest;
},
},
// ๐ Computed properties
computed: {
capitalizedName1() {
return this.capitalized(this.name1);
},
capitalizedName2() {
return this.capitalized(this.name2);
},
},
};
</script>
Using a computed property for this is useful when the data is likely to change in the app’s lifetime, e.g., in a two-way binding with an input field. When the data property changes, the depending computed property will be recalculated and updated automatically.