To check if a checkbox is checked in React:
- Create a boolean state variable to store the value of the checkbox.
- Set an
onChange
event listener on the input checkbox. - In the listener, use the event object’s
target.checked
property to check if the checkbox is checked. - Store the
checked
value in a state variable to be able to check whether the checkbox is checked from outside the event listener.
import { useState } from 'react';
export default function App() {
const [agreement, setAgreement] = useState(false);
const handleChange = (event) => {
setAgreement(event.target.checked);
}
return (
<div>
<div id="app">
<input
type="checkbox"
name="agreement"
onChange={handleChange}
/>
<label for="agreement">
I agree to the terms and conditions
</label>
<br /><br />
<button disabled={!agreement}>Continue</button>
</div>
</div>
);
}
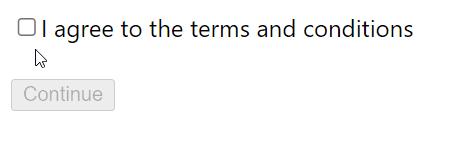
The event object’s target
property represents the checkbox input
element; the value of its checked
property indicates whether the checkbox is checked or not.
The event object is passed to the onChange
listener, which will be invoked whenever the checkbox is checked or unchecked.
We use the useState
React hook to store the checkbox’s checked state. useState
returns an array of two values; the first is a variable that stores the state, and the second is a function that updates the state when it is called.
So every time the checkbox is checked or unchecked, the agreement
state variable will be automatically toggled to true
or false
.
We set the button’s disabled
prop to the negation of agreement
to disable and enable it when agreement
is true
and false
respectively.
Check if checkbox is checked with ref
Instead of controlling the checkbox’s checked value with React state, we can create an uncontrolled checkbox and check whether it is checked with a ref.
For example:
import { useState, useRef } from 'react';
export default function App() {
const [message, setMessage] = useState('');
const checkbox = useRef();
const handleClick = () => {
if (checkbox.current.checked) {
setMessage('You know JS');
} else {
setMessage("You don't know JS");
}
}
return (
<div>
<div id="app">
<input
type="checkbox"
name="js"
ref={checkbox}
/>
<label for="js"> JavaScript </label>
<br />
<button onClick={handleClick}>Done</button>
<p>{message}</p>
</div>
</div >
);
}
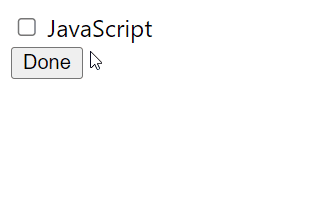
The data in a controlled input is handled by React state, but for uncontrolled inputs, it is handled by the DOM itself. This is why the input
checkbox in the example above doesn’t have a value
prop or onChange
event handler set. Instead, we check if the checkbox is checked with a React ref. The DOM updates the checked value when the user toggles the checkbox.
We create a ref object with the useRef
hook and assign it to the ref
prop of the checkbox input
. Doing this sets the current
property of the ref object to the DOM object that represents the checkbox.
useRef
returns a mutable object that maintains its value when a component updates. Also, modifying the value of this object’s current
property doesn’t cause a re-render. This is unlike the setState
update function return from the useState
hooks.
Although the React documentation recommends using controlled components, uncontrolled components offer some advantages. You might prefer them if the form is very simple and doesn’t need instant validation, and values only need to be accessed when the form is submitted.
Hey man,
Really appreciate your effort on sharing your knowledge.
Keep it up!!
Thank you for your appreciation!