How to earn full or side income as a freelance developer in 2024
Don’t waste time cold applying to job listings.
Your success rate will be painfully low after all that time invested. There is a better way that many never use.
Many have used it to guarantee responses and interviews every single time. This is my #1 way to find clients.
I use LinkedIn as a case study, but the strategy applies to any place you interact with other people.
It’s all about referrals at its core.
Referrals drastically improve your chances of getting a job at any company.
But you need to do it right.
Follow this ultimate step-by-step guide to landing high-paying, flexible freelance + full-time jobs.
1. Plan: Know what you want
This is extremely important, especially for future jobs.
The broader your niche the more jobs for you — but there’s more competition.
Example: “Web developer”.
Smaller niche makes you the big fish in a small pond — less jobs but standing out takes far less time.
Example: “Next.js developer, implements social media integrations into web apps”. Super specific.
If you’re just starting without much experience, start small. Then you can slowly broaden your niche as you gain experience and social proof.
2. Find jobs in your niche the right way
Now start action.
Find jobs in your niche don’t apply directly.
What are the best places to find jobs?
Wellfound
I previously talked about the massive number of software jobs from rapidly growing startups on Wellfound.
It has over 49,000 jobs on there right now with not enough people to fill them — incredible opportunity.
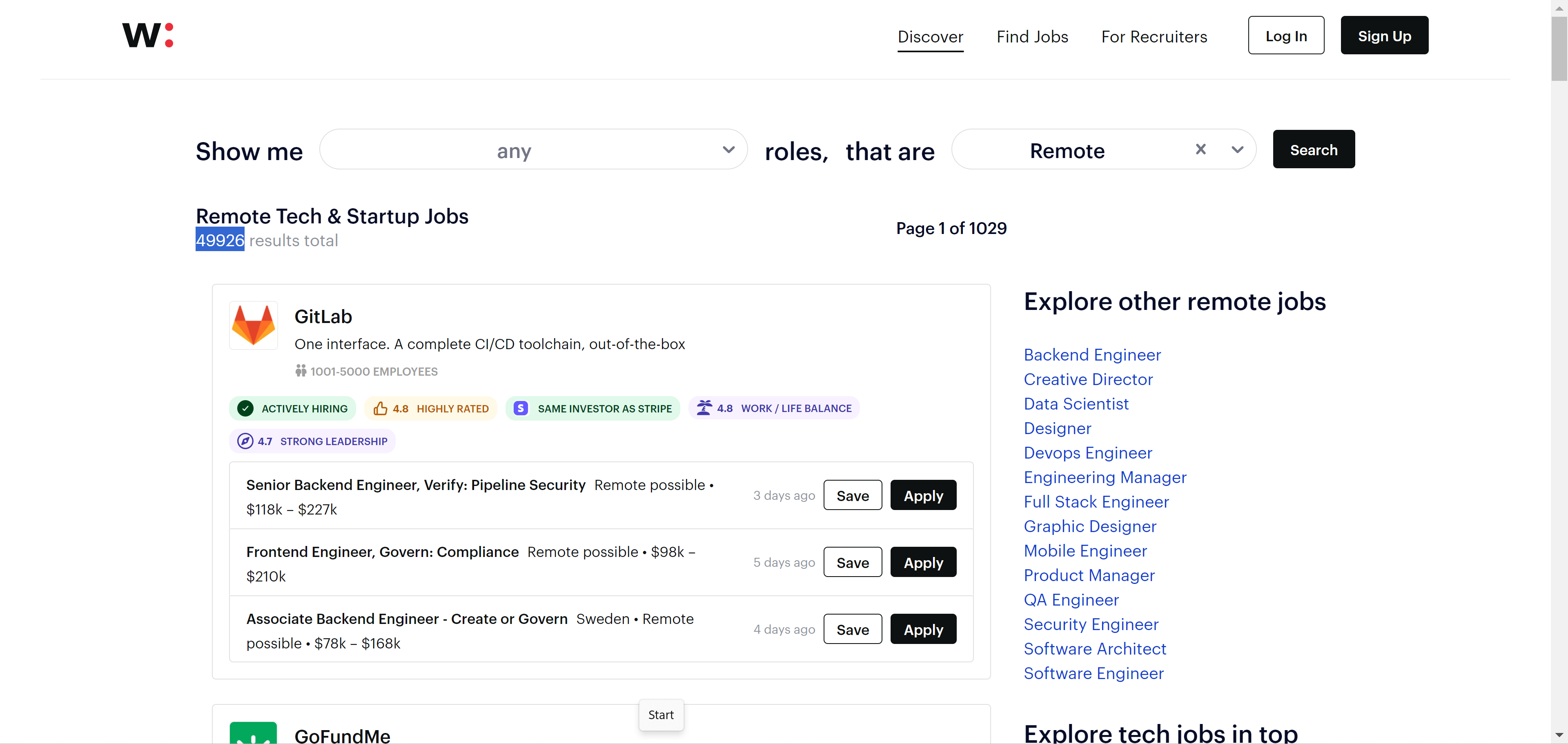
LinkedIn Jobs
LinkedIn is full of the latest job listings from high-quality companies. Filter by your niche and add to your collection.
3. Create a unique LinkedIn profile
It’s the first thing anyone checking you out will see — make it incredible.
First impression matters everywhere and LinkedIn is no exception.
Great photo
Use a high-quality face photo with great lighting that shows confidence.
You can smile to appear friendly and approachable — not necessary though! Too fake for some.
Attention-grabbing bio
Forget about being quirky or cute here.
You mean business and the first piece of text describing you must show this.
What should you put in your bio?
1. Your niche
You know your ideal job type, now make it known to the world. Let people know you’re qualified.
2. Impressive achievement
Include a notable achievement to grab attention.
Example: 1,000+ stars on GitHub.
3. Open to work
Add “freelancer” if you’re specifically doing freelance.
Many won’t want to waste even a little energy to ask if you’re open to work; Let them be sure from the start.
Also use the #OpenToWork LinkedIn feature.
4. Start building your network with intent — like this
You’ve built up your LinkedIn profile beautifully, now it’s time to find valuable people.
Don’t just add any random person — focus on people who meet these criteria:
1. Connected to the target job
People who work at the companies of the job openings you found.
And people who know those people — 2nd-degree connections.
2. Something in common
- Is their industry and niche similar to yours? More on this shortly.
- Did they go to the same school as you?
- Are you two from the same city? Or the same not-so-popular country?
We are tribal and more likely with people similar to us in an important way.
3. Creators
Target software developers like yourself. Then designers, writers, authors — creators in general. But prioritize people who code professionally.
Focus on quality AND quantity connections.
The more people in your network the more likely opportunities will come your way.
And you can add up to 30,000 connections!
5. Nurture your relationships
Adding people is NOT enough — You must patiently strengthen your connections with them.
And how do you do that?
Engage
If you don’t show up in people’s lives, they’ll forget about your existence.
Leave genuine thoughtful comments on their posts.
Like posts that resonate with you — but don’t just like every post or you’ll cheapen the value — like a chronic flatterer.
Send them personal messages offering value. Ask them for advice and make them feel valued and invested in you.
Create posts
Another great way to offer value and maintain the relationship.
Share what you know and what you’ve done. Don’t give us coding platitudes — everybody knows about <br />
tag. We all know about HTTP POST.
Try not to always share cold impersonal facts; sprinkle in your thoughts and opinions. Make jokes without causing painful cringe.
6. Ask for referrals
The best way to do this: Ask to meet them physically or virtually to learn more about the role or company.
Prepare engaging questions in advance to show genuine interest in the company — not just about getting a job.
If you’ve built a cordial relationship at this point, they’ll probably ask you if you want a referral — ✅.
If not, you can say something like, “This sounds like an amazing place to work! Would it be possible to get a referral if I applied?”.
You don’t even have to tie the referral to any specific role — you can ask them to keep you posted with job requests.
If they’re a great developer they’re probably high in demand and get more offers than they can handle. With a strong relationship, they’ll be more than happy to refer you.
People trust people they know. You’re far more likely to get the job with referrals than with cold job listings.
Final thoughts
Follow this strategy focus and intent, and you’ll start landing high-paying freelance jobs faster than you think with high certainty.